Leveraging Laravel's Features for Both Frontend and Backend Development
Laravel can be effectively used for both frontend and backend development. 1) Backend: Utilize Laravel's Eloquent ORM for simplified database interactions. 2) Frontend: Leverage Blade templates for clean HTML and integrate Vue.js for dynamic SPAs, ensuring seamless frontend-backend integration.
When diving into the world of web development with Laravel, it's fascinating to see how this PHP framework can serve as a powerhouse for both frontend and backend development. Laravel isn't just about crafting robust server-side applications; it also offers a suite of features that can significantly enhance your frontend work. This dual capability makes Laravel a go-to choice for developers looking to streamline their workflow and deliver seamless user experiences.
Let's explore how Laravel can be your secret weapon for both frontend and backend development. From my experience, the key lies in understanding and utilizing Laravel's built-in tools and functionalities to their fullest potential.
For the backend, Laravel's elegance shines through its expressive syntax, robust ORM (Eloquent), and powerful routing system. I've found that Eloquent, in particular, simplifies database interactions to an extent that it feels like you're working with objects rather than rows in a database. Here's a quick example of how Eloquent can be used:
// Fetching all users with their posts $users = User::with('posts')->get(); // Creating a new user $user = User::create([ 'name' => 'John Doe', 'email' => 'john@example.com', 'password' => bcrypt('password123'), ]);
On the frontend side, Laravel's Blade templating engine is a game-changer. It allows you to write clean, maintainable HTML templates with PHP logic embedded directly within them. This integration means you can keep your frontend logic close to your backend, reducing the complexity of managing separate frontend and backend codebases. Here's a snippet of how you might use Blade:
<!-- resources/views/welcome.blade.php --> @extends('layouts.app') @section('content') <h1 id="Welcome-Auth-user-name">Welcome, {{ Auth::user()->name }}!</h1> @if(count($posts) > 0) <ul> @foreach($posts as $post) <li>{{ $post->title }}</li> @endforeach </ul> @else <p>No posts yet!</p> @endif @endsection
One of the most powerful aspects of Laravel for frontend development is its integration with Vue.js. Laravel comes with Vue.js out of the box, allowing you to build dynamic, single-page applications (SPAs) right within your Laravel project. This integration is seamless, and it's where I've seen developers truly leverage Laravel's potential for frontend work. Here's a basic example of how you might set up a Vue component in Laravel:
// resources/js/components/ExampleComponent.vue <template> <div> <h1 id="message">{{ message }}</h1> </div> </template> <script> export default { data() { return { message: 'Hello from Vue!' } } } </script>
To use this component in your Blade template, you simply include it like so:
<!-- resources/views/welcome.blade.php --> @extends('layouts.app') @section('content') <example-component></example-component> @endsection
When it comes to leveraging Laravel for both frontend and backend, there are a few considerations and best practices to keep in mind:
API Development: Laravel is excellent for building RESTful APIs. By using Laravel's API resources and route model binding, you can create clean, maintainable APIs that serve as the backbone for your frontend applications. However, be cautious about over-fetching data; always consider what data your frontend really needs.
State Management: When integrating Vue.js with Laravel, managing state becomes crucial. While Vuex is a popular choice for state management in Vue applications, consider whether your application's complexity truly warrants it. Sometimes, simpler solutions like using Vue's reactivity system or even Laravel's session can suffice.
Performance: Laravel's eager loading capabilities with Eloquent can significantly improve performance by reducing the number of database queries. However, be mindful of N 1 query problems, which can sneak up on you and degrade performance.
Security: Laravel provides robust security features out of the box, like CSRF protection and input validation. When building frontend applications, ensure you're leveraging these features to protect your application from common vulnerabilities.
In my journey with Laravel, I've learned that the key to successfully leveraging its features for both frontend and backend development is to strike a balance. You want to utilize Laravel's strengths without overcomplicating your project. For instance, while Laravel's Blade templates are powerful, for more complex frontend logic, you might want to lean more heavily on Vue.js components.
One pitfall I've encountered is the temptation to overuse Laravel's backend features in the frontend. For example, while it's tempting to use Laravel's session management for frontend state, this can lead to tight coupling between your frontend and backend, making it harder to separate concerns or even switch to a different backend technology in the future.
In conclusion, Laravel's versatility in handling both frontend and backend development is one of its strongest suits. By understanding and applying its features wisely, you can create applications that are not only powerful and efficient but also maintainable and scalable. Whether you're building a simple blog or a complex SPA, Laravel provides the tools you need to succeed on both ends of the development spectrum.
The above is the detailed content of Leveraging Laravel's Features for Both Frontend and Backend Development. For more information, please follow other related articles on the PHP Chinese website!

Collaborative document editing is an effective tool for distributed teams to optimize their workflows. It improves communication and project progress through real-time collaboration and feedback loops, and common tools include Google Docs, Microsoft Teams, and Notion. Pay attention to challenges such as version control and learning curve when using it.

ThepreviousversionofLaravelissupportedwithbugfixesforsixmonthsandsecurityfixesforoneyearafteranewmajorversion'srelease.Understandingthissupporttimelineiscrucialforplanningupgrades,ensuringprojectstability,andleveragingnewfeaturesandsecurityenhancemen

Laravelcanbeeffectivelyusedforbothfrontendandbackenddevelopment.1)Backend:UtilizeLaravel'sEloquentORMforsimplifieddatabaseinteractions.2)Frontend:LeverageBladetemplatesforcleanHTMLandintegrateVue.jsfordynamicSPAs,ensuringseamlessfrontend-backendinteg

Laravelcanbeusedforfullstackdevelopment.1)BackendmasterywithLaravel'sexpressivesyntaxandfeatureslikeEloquentORMfordatabasemanagement.2)FrontendintegrationusingBladefordynamicHTMLtemplates.3)EnhancingfrontendwithLaravelMixforassetcompilation.4)Fullsta

Answer: The best tools for upgrading Laravel include Laravel's UpgradeGuide, LaravelShift, Rector, Composer, and LaravelPint. 1. Use Laravel's UpgradeGuide as the upgrade roadmap. 2. Use LaravelShift to automate most of the upgrade work, but it requires manual review. 3. Automatically refactor the code through Rector, and you need to understand and possibly customize its rules. 4. Use Composer to manage dependencies and pay attention to possible dependency conflicts. 5. Run LaravelPint to maintain code style consistency, but it does not solve the functional problems.

ToenhanceengagementandcohesionamongdistributedteamsbeyondZoom,implementthesestrategies:1)Organizevirtualcoffeebreaksforinformalchats,2)UseasynchronoustoolslikeSlackfornon-workdiscussions,3)Introducegamificationwithteamgamesorchallenges,and4)Encourage

Laravel10introducesseveralbreakingchanges:1)ItrequiresPHP8.1orhigher,2)TheRouteServiceProvidernowusesabootmethodforloadingroutes,3)ThewithTimestamps()methodonEloquentrelationshipsisdeprecated,and4)TheRequestclassnowpreferstherules()methodforvalidatio

Tomaintainfocusandmotivationinremotework,createastructuredenvironment,managedigitaldistractions,fostermotivationthroughsocialinteractionsandgoalsetting,maintainwork-lifebalance,anduseappropriatetechnology.1)Setupadedicatedworkspaceandsticktoaroutine.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
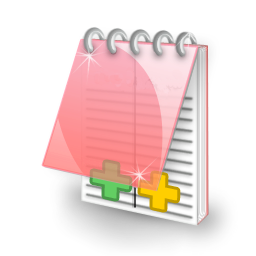
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
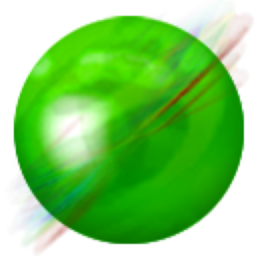
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
