How to implement microservices and interfaces for permissions in Laravel
How to implement microservices and interfaces of permissions in Laravel
With the expansion of the scale of software systems and the complexity of business, permission management has become more and more complex. The more important it is. In popular PHP frameworks like Laravel, implementing microservices and interfaces for permissions can help us better organize and manage permissions, and achieve sharing and reuse of permissions between different applications and modules. This article will introduce how to implement microservices and interfaces for permissions in Laravel, and provide code examples.
1. Microservices of permissions
The so-called microservices of permissions mean that the permissions function is separated into an independent service and provided to other applications or modules through API interfaces. The advantage of this is that it can achieve unified management and reuse of permissions and avoid repeated definition and maintenance of permissions.
- Create permission service
First, we need to create an independent permission service. In Laravel, this can be achieved by creating a separate project or module.
- Define the permission data structure
In the permission service, we need to define the permission data structure. Generally speaking, permissions can be divided into two levels: roles and permissions.
In Laravel, we can use database tables to define the data structure of permissions, such as creating a roles
table and a permissions
table.
// roles 表 Schema::create('roles', function (Blueprint $table) { $table->increments('id'); $table->string('name')->unique(); $table->timestamps(); }); // permissions 表 Schema::create('permissions', function (Blueprint $table) { $table->increments('id'); $table->string('name')->unique(); $table->timestamps(); });
- Implement the interface for adding, deleting, modifying and checking permissions
In the permission service, we need to implement the interface for adding, deleting, modifying and checking permissions for calls by other applications or modules. For example, the following interface can be implemented:
class RoleController extends Controller { public function index() { return Role::all(); } public function show($id) { return Role::findOrFail($id); } public function store(Request $request) { // 保存角色数据 } public function update(Request $request, $id) { // 更新角色数据 } public function destroy($id) { // 删除角色数据 } }
Through the above steps, we can create an independent permission service and provide the function of adding, deleting, modifying and checking permissions through the API interface.
2. Interface of permissions
In addition to separating the permission function into an independent service, the permission function can also be provided to other applications or modules in the form of an interface. Through interfaced permissions, we can enable different applications or modules to share and reuse permission functions, improving the flexibility and maintainability of the system.
- Create permission interface
In Laravel, we can use Laravel's routing function to create a permission interface. Permission-related routes can be defined in the routes/api.php
file.
// 获取所有角色 Route::get('/roles', [RoleController::class, 'index']); // 获取指定角色 Route::get('/roles/{id}', [RoleController::class, 'show']); // 创建角色 Route::post('/roles', [RoleController::class, 'store']); // 更新角色 Route::put('/roles/{id}', [RoleController::class, 'update']); // 删除角色 Route::delete('/roles/{id}', [RoleController::class, 'destroy']);
- Call permission interface
Other applications or modules can obtain and manage permissions by calling the permission interface. For example, you can use Axios
to send an HTTP request to obtain the data of all roles:
axios.get('/api/roles') .then((response) => { console.log(response.data); }) .catch((error) => { console.error(error); });
Through the above steps, we can provide the permission function in the form of an interface to other applications or modules.
Summary:
Through the above steps, we can implement microservices and interfaces for permissions in Laravel. By separating the permission function into an independent service and providing it to other applications or modules through the API interface, unified management and reuse of permissions can be achieved, and the flexibility and maintainability of the system can be improved. I hope this article will be helpful to implement microservices and interfaces of permissions in Laravel.
The above is the detailed content of How to implement microservices and interfaces for permissions in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

Laravel's popularity includes its simplified development process, providing a pleasant development environment, and rich features. 1) It absorbs the design philosophy of RubyonRails, combining the flexibility of PHP. 2) Provide tools such as EloquentORM, Blade template engine, etc. to improve development efficiency. 3) Its MVC architecture and dependency injection mechanism make the code more modular and testable. 4) Provides powerful debugging tools and performance optimization methods such as caching systems and best practices.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.

LaravelisabackendframeworkbuiltonPHP,designedforwebapplicationdevelopment.Itfocusesonserver-sidelogic,databasemanagement,andapplicationstructure,andcanbeintegratedwithfrontendtechnologieslikeVue.jsorReactforfull-stackdevelopment.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
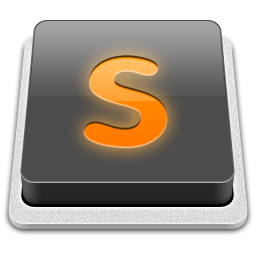
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
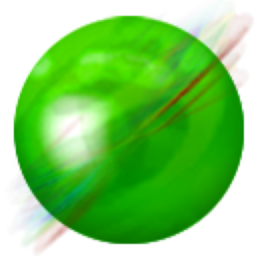
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment