


Advanced application of Laravel permission function: How to implement fine-grained permission control requires specific code examples
As the complexity of web applications continues to increase, for The management and control of user rights have also become more important. The Laravel framework provides rich permission functions to facilitate us to manage user roles and permissions. However, sometimes we need to implement more fine-grained permission control, that is, to restrict permissions for a specific operation. This article will introduce how to implement fine-grained permission control in the Laravel framework and give specific code examples.
First, we need to create corresponding tables in the database to store roles, permissions, and permission-role relationships. Create a table named "roles" that contains "id" and "name" fields to store the unique ID and name of the role. Create a table named "permissions" that contains "id" and "name" fields to store the unique identifier and name of the permission. Create a table named "permission_role" that contains the "permission_id" and "role_id" fields to store the relationship between permissions and roles.
Next, we need to define the models of roles and permissions, and establish a many-to-many relationship between the models. First, we create a model named "Role" and define the corresponding relationship with the "roles" table. In this model, we need to define a many-to-many relationship with the "permissions" table. The code is as follows:
namespace AppModels; use IlluminateDatabaseEloquentFactoriesHasFactory; use IlluminateDatabaseEloquentModel; class Role extends Model { use HasFactory; public function permissions() { return $this->belongsToMany(Permission::class, 'permission_role'); } }
Then, we create a model named "Permission" and define it with the "permissions" table. corresponding relationship. In this model, we need to define a many-to-many relationship with the "roles" table, the code is as follows:
namespace AppModels; use IlluminateDatabaseEloquentFactoriesHasFactory; use IlluminateDatabaseEloquentModel; class Permission extends Model { use HasFactory; public function roles() { return $this->belongsToMany(Role::class, 'permission_role'); } }
Here, we pass $this->belongsToMany()
Method to define a many-to-many relationship, the first parameter is the associated model, and the second parameter is the associated intermediate table name.
Next, we need to define the association with roles and permissions in the user model. In "LaravelJetstream", this can be achieved by modifying the AppModelsUser
model. In the user model, we need to define a many-to-many relationship with the "roles" table. The code is as follows:
namespace AppModels; use IlluminateFoundationAuthUser as Authenticatable; use IlluminateDatabaseEloquentFactoriesHasFactory; use IlluminateDatabaseEloquentSoftDeletes; class User extends Authenticatable { use HasFactory, SoftDeletes; // ... public function roles() { return $this->belongsToMany(Role::class, 'role_user'); } public function hasPermission($permission) { foreach ($this->roles as $role) { if ($role->permissions()->where('name', $permission)->exists()) { return true; } } return false; } }
In the above code, we define hasPermission($permission)
Method used to check whether the user has a certain permission. This method iterates through the roles the user has and checks whether each role has the permission.
Now, we can use these roles and permissions for fine-grained permission control in the application. Let's say we have a permission called "create-post" and we only want users with that permission to be able to create posts. In the controller, we can call the $user->hasPermission('create-post')
method to check whether the user has the permission before performing relevant operations. If the user has this permission, continue to perform related operations; otherwise, an error message can be returned or redirected to other pages.
namespace AppHttpControllers; use IlluminateHttpRequest; class PostController extends Controller { public function create(Request $request) { $user = $request->user(); if ($user->hasPermission('create-post')) { // 允许用户创建文章 } else { // 不允许用户创建文章 } } }
In the above code, we obtain the currently logged in user through the $request->user()
method, and then call hasPermission('create-post')
Method to check if the user has permission to create articles.
Through the above steps, we can achieve fine-grained permission control in the Laravel framework. By defining model relationships of roles, permissions, and intermediate tables, we can easily manage and control user permissions. By calling the $user->hasPermission($permission)
method, we can check whether the user has the corresponding permissions before the specific operation is performed. This fine-grained permission control can improve application security and controllability, ensuring that only users with appropriate permissions can perform certain operations.
The above is the method and sample code to implement fine-grained permission control in the Laravel framework. By rationally utilizing the permissions functions provided by the Laravel framework, we can better manage and control user permissions and make applications more secure and reliable.
The above is the detailed content of Advanced application of Laravel permission function: how to achieve fine-grained permission control. For more information, please follow other related articles on the PHP Chinese website!
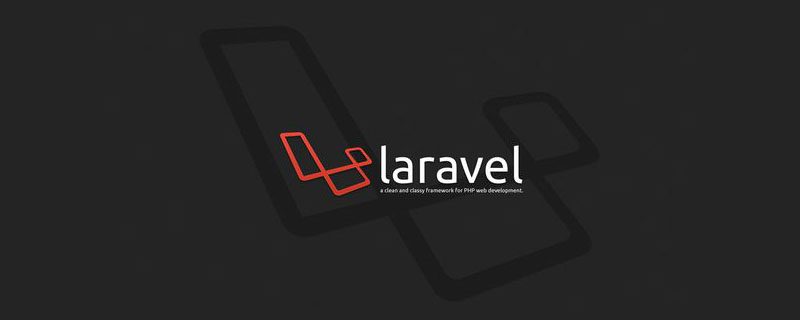
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
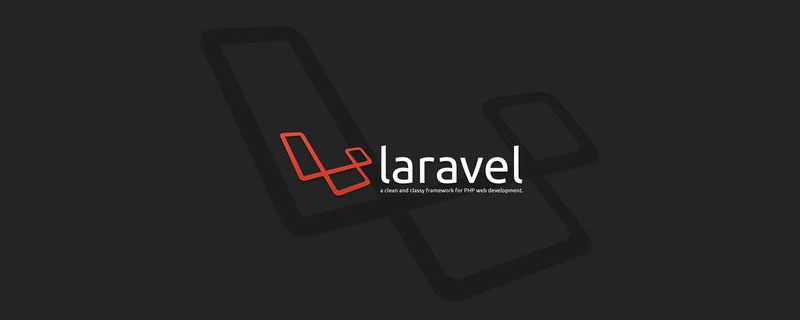
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
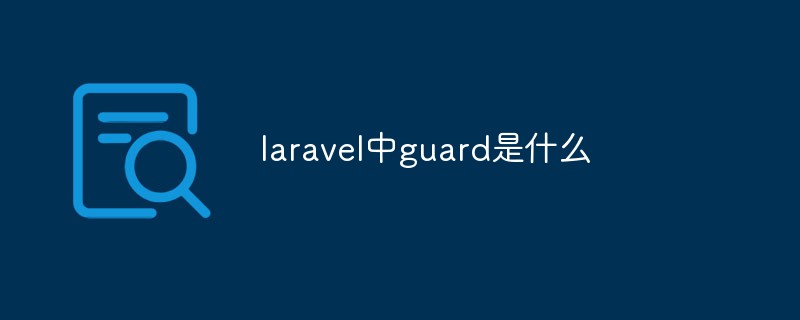
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
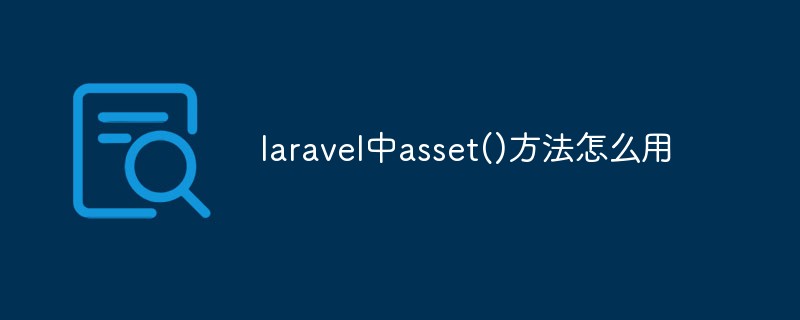
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
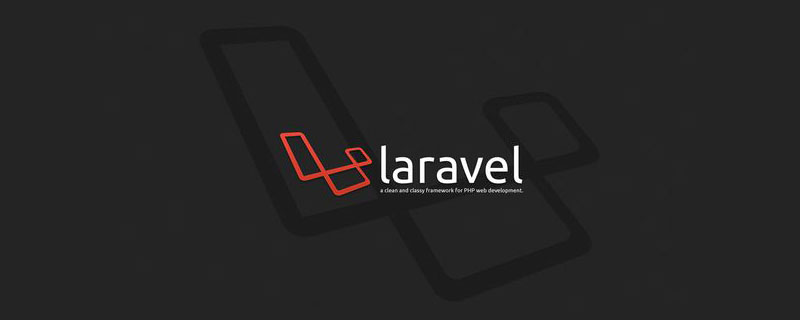
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
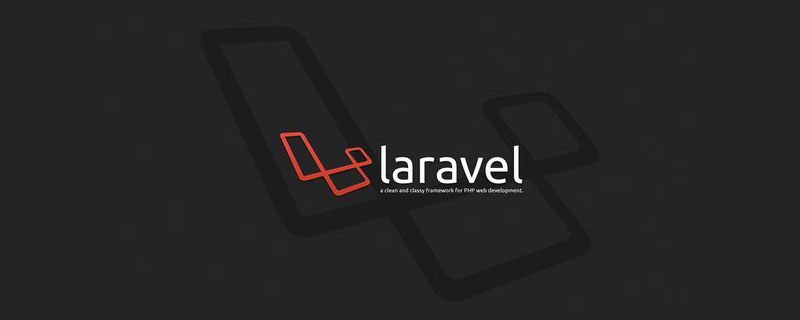
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。
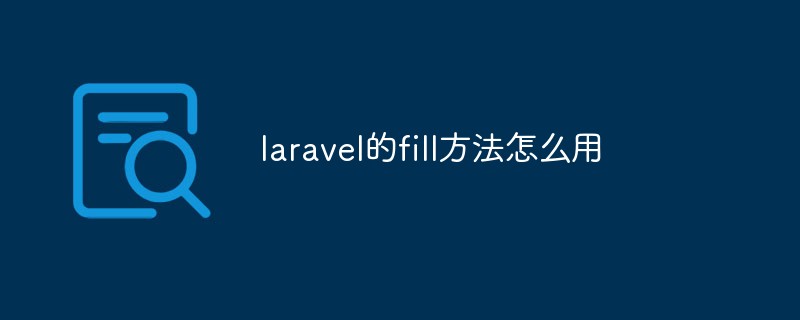
在laravel中,fill方法是一个给Eloquent实例赋值属性的方法,该方法可以理解为用于过滤前端传输过来的与模型中对应的多余字段;当调用该方法时,会先去检测当前Model的状态,根据fillable数组的设置,Model会处于不同的状态。
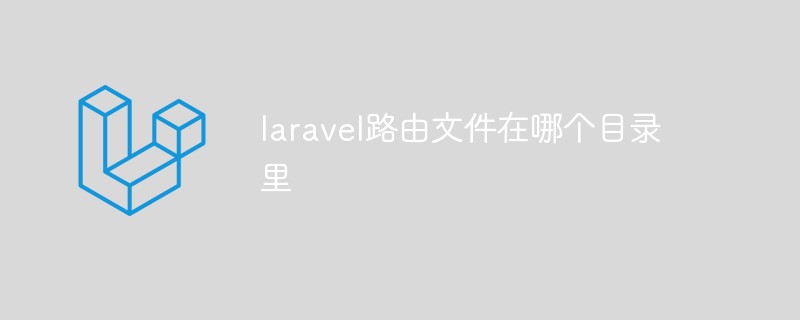
laravel路由文件在“routes”目录里。Laravel中所有的路由文件定义在routes目录下,它里面的内容会自动被框架加载;该目录下默认有四个路由文件用于给不同的入口使用:web.php、api.php、console.php等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
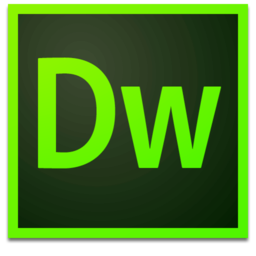
Dreamweaver Mac version
Visual web development tools
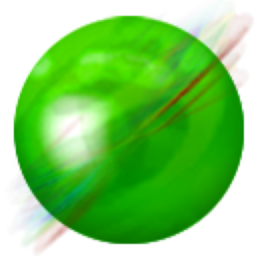
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
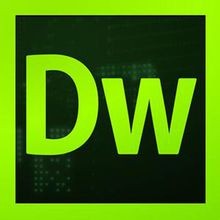
Dreamweaver CS6
Visual web development tools