How to use middleware for data decryption transmission in Laravel
How to use middleware for data decryption and transmission in Laravel
In modern web applications, the security of data transmission is crucial. Especially when it comes to the transmission of sensitive user information, we need to take appropriate security measures to protect this data. The Laravel framework provides an easy way to encrypt and decrypt data for transmission - using middleware.
Middleware is a core feature of the Laravel framework, which allows us to insert custom code into the request processing process. We can use middleware to implement data encryption and decryption operations. This article will focus on how to use middleware for data decryption transmission in Laravel applications.
First, we need to generate a middleware. Run the following command on the command line to generate a middleware named DecryptMiddleware:
php artisan make:middleware DecryptMiddleware
The generated middleware file will be located in the app/Http/Middleware directory. Open the DecryptMiddleware.php file and add the following code in the handle method:
<?php namespace AppHttpMiddleware; use Closure; class DecryptMiddleware { public function handle($request, Closure $next) { $encryptedData = $request->getContent(); $decryptedData = decrypt($encryptedData); $request->replace(json_decode($decryptedData, true)); return $next($request); } }
In the above code, we first obtain the encrypted data from the request. Then, use the decrypt function provided by Laravel to decrypt the data. After decryption, we convert the data into an associative array and replace it with the original request data. Finally, we pass the request to the next middleware or route for processing by calling $next($request).
Next, we need to use middleware to define which routes or routing groups require data decryption and transmission.
Find the $middlewareGroups array in the app/Http/Kernel.php file and add our DecryptMiddleware to it:
protected $middlewareGroups = [ 'web' => [ // ... // 其他中间件 // ... AppHttpMiddlewareDecryptMiddleware::class, ], 'api' => [ 'throttle:60,1', 'bindings', // 其他中间件 AppHttpMiddlewareDecryptMiddleware::class, ], ];
In the above code snippet, we added DecryptMiddleware to 'web' middleware group and 'api' middleware group. This means that all routes in these groups will be decrypted by the DecryptMiddleware.
Now, we only need to use these middleware groups in our route definition to realize the decryption and transmission of data.
For example, in the routes/api.php file, we can define the following route:
<?php use IlluminateSupportFacadesRoute; Route::group(['middleware' => ['api']], function () { Route::post('/users', 'UserController@store'); // ... // 其他路由 // ... });
In the above code, we specified the 'middleware' option in the routing group and set it to ['api'], this will apply all middleware registered in the 'middlewareGroups' array to this routing group.
So far, we have used middleware to implement data decryption and transmission. Now, when the request goes through the route with middleware, the data will be automatically decrypted.
It should be noted that we use the encryption and decryption functions encrypt and decrypt provided by Laravel in the example. These functions use the application's keys for encryption and decryption operations. Therefore, before using middleware, make sure the correct keys are set up in your application.
To summarize, by using middleware, we can easily implement data decryption and transmission in Laravel. Just follow the steps above to generate the middleware, add the middleware to a middleware group, and then use the middleware group in routes that need to decrypt transmissions. In this way, we are able to protect the secure transmission of users' sensitive data.
The above is the detailed content of How to use middleware for data decryption transmission in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel optimizes the web development process including: 1. Use the routing system to manage the URL structure; 2. Use the Blade template engine to simplify view development; 3. Handle time-consuming tasks through queues; 4. Use EloquentORM to simplify database operations; 5. Follow best practices to improve code quality and maintainability.

Laravel is a modern PHP framework that provides a powerful tool set, simplifies development processes and improves maintainability and scalability of code. 1) EloquentORM simplifies database operations; 2) Blade template engine makes front-end development intuitive; 3) Artisan command line tools improve development efficiency; 4) Performance optimization includes using EagerLoading, caching mechanism, following MVC architecture, queue processing and writing test cases.

Laravel's MVC architecture improves the structure and maintainability of the code through models, views, and controllers for separation of data logic, presentation and business processing. 1) The model processes data, 2) The view is responsible for display, 3) The controller processes user input and business logic. This architecture allows developers to focus on business logic and avoid falling into the quagmire of code.

Laravel is a PHP framework based on MVC architecture, with concise syntax, powerful command line tools, convenient data operation and flexible template engine. 1. Elegant syntax and easy-to-use API make development quick and easy to use. 2. Artisan command line tool simplifies code generation and database management. 3.EloquentORM makes data operation intuitive and simple. 4. The Blade template engine supports advanced view logic.

Laravel is suitable for building backend services because it provides elegant syntax, rich functionality and strong community support. 1) Laravel is based on the MVC architecture, simplifying the development process. 2) It contains EloquentORM, optimizes database operations. 3) Laravel's ecosystem provides tools such as Artisan, Blade and routing systems to improve development efficiency.
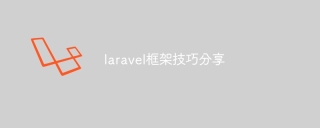
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
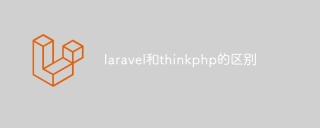
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
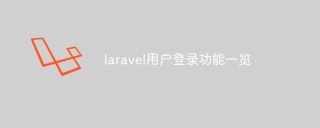
Building user login capabilities in Laravel is a crucial task and this article will provide a comprehensive overview covering every critical step from user registration to login verification. We will dive into the power of Laravel’s built-in verification capabilities and guide you through customizing and extending the login process to suit specific needs. By following these step-by-step instructions, you can create a secure and reliable login system that provides a seamless access experience for users of your Laravel application.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
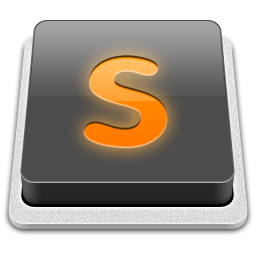
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.