How to use middleware for data recovery in Laravel
#Laravel is a popular PHP web application framework that provides many fast and easy ways to build efficient, secure and scalable web applications. When developing Laravel applications, we often need to consider the issue of data recovery, that is, how to recover data and ensure the normal operation of the application in the event of data loss or damage. In this article, we will introduce how to use Laravel middleware to implement data recovery functions and provide specific code examples.
1. What is Laravel middleware?
Laravel middleware is a piece of code that executes before or after a request reaches your application. They can be used for operations such as verification, data processing, and data recovery. In Laravel, middleware is typically used to perform some filtering or protection operations and then forward the request to the appropriate controller or handler.
In this article, we will use middleware to restore data in the application. Specifically, if the requested data is lost or corrupted, we will attempt to restore the data from backup storage to ensure the application operates properly.
2. How to use middleware for data recovery in Laravel?
In Laravel, there are two types of middleware: global middleware and routing middleware. Global middleware is middleware that is executed before all requests from the application reach the controller, while routing middleware is just middleware that is applied on a specified route. In this article, we will use global middleware to implement data recovery functionality.
- Create a global middleware
First, we need to create a new middleware. You can create a middleware named RestoreData using the following command:
php artisan make:middleware RestoreData
This command will create a new RestoreData in the app/Http/Middleware directory .php file, which contains a handle method and some comments.
- Modify the middleware
Next, we need to modify the RestoreData middleware to our needs. We will perform the data recovery operation in the handle method.
namespace AppHttpMiddleware;
use Closure;
use IlluminateSupportFacadesCache;
class RestoreData
{
public function handle($request, Closure $next) { // 尝试从缓存中获取数据 $data = Cache::get('backup_data'); // 如果缓存数据不存在,尝试从备份存储中获取数据 if(!$data){ $backup_data = $request->cookie('backup_data'); if($backup_data){ $data = json_decode($backup_data, true); // 将备份数据存储到缓存中 Cache::put('backup_data', $data, 60); } } // 如果数据存在,将其注入到请求中 if($data){ $request->merge(['data' => $data]); } return $next($request); }
}
In the above code, we first try to get the data from the cache. If there is no data in the cache, then get the data from the backup storage. We store the backup data in the requested cookie and convert it into an array format. Finally, we store the data in the request data for subsequent processing.
- Register middleware
We need to register the RestoreData middleware into the application and set the global middleware. We can register in the app/Http/Kernel.php file:
namespace AppHttp;
use IlluminateFoundationHttpKernel as HttpKernel;
class Kernel extends HttpKernel
{
protected $middleware = [ IlluminateFoundationHttpMiddlewareCheckForMaintenanceMode::class, IlluminateFoundationHttpMiddlewareValidatePostSize::class, AppHttpMiddlewareTrimStrings::class, IlluminateFoundationHttpMiddlewareConvertEmptyStringsToNull::class, AppHttpMiddlewareRestoreData::class, // 将自定义中间件添加到全局中间件 ]; // ...
}
Now, we have completed the writing and registration of the data recovery middleware. However, we also need to use the data in our application. We can access the data in the controller or model and process it.
namespace AppHttpControllers;
use IlluminateHttpRequest;
class HomeController extends Controller
{
public function index(Request $request) { $data = $request->input('data'); // ... }
}
In the above code, we can get the data from the request and use it to perform the corresponding operation.
3. Summary
In this article, we introduced how to use middleware for data recovery in Laravel. We created a global middleware in which the data recovery logic was implemented. We also added middleware to the application and used data in controllers or models. We hope this article was helpful and that you can better understand how Laravel middleware works.
The above is the detailed content of How to use middleware for data recovery in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

Laravel's popularity includes its simplified development process, providing a pleasant development environment, and rich features. 1) It absorbs the design philosophy of RubyonRails, combining the flexibility of PHP. 2) Provide tools such as EloquentORM, Blade template engine, etc. to improve development efficiency. 3) Its MVC architecture and dependency injection mechanism make the code more modular and testable. 4) Provides powerful debugging tools and performance optimization methods such as caching systems and best practices.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
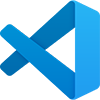
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use