How to design a simple teacher scoring system in Java?
How to design a simple teacher scoring system in Java?
With the development of education, evaluating the work of teachers has become more and more important. In order to better evaluate teachers' teaching quality, it is necessary to design a simple teacher rating system. This article will introduce how to design a simple teacher scoring system using Java language.
First, we need to determine the need for a teacher grading system. The main functions of the teacher scoring system include: entering teacher information, entering student evaluations, calculating scoring results, displaying scoring results, etc.
In order to realize these functions, we need to design corresponding classes and methods. First, create a class named Teacher to store and manage teacher information. The Teacher class can contain attributes such as the teacher's name, ID, subjects taught, and methods related to grading.
public class Teacher { private String name; private String id; private String subject; // 构造方法 public Teacher(String name, String id, String subject) { this.name = name; this.id = id; this.subject = subject; } // 设置和获取教师信息的方法 public void setName(String name) { this.name = name; } public String getName() { return name; } public void setId(String id) { this.id = id; } public String getId() { return id; } public void setSubject(String subject) { this.subject = subject; } public String getSubject() { return subject; } // 计算评分结果的方法 public float calculateRating() { // 根据学生评价计算教师评分 // 这里可以假设评分算法为简单的平均分 float[] ratings = {4.5f, 3.2f, 4.8f}; // 假设有3个学生的评分 float sum = 0; for (float rating : ratings) { sum += rating; } return sum / ratings.length; } }
Next, we create a class named Student to store and manage student information and ratings. The Student class can contain attributes such as the student's name, ID, and scoring methods.
public class Student { private String name; private String id; // 构造方法 public Student(String name, String id) { this.name = name; this.id = id; } // 设置和获取学生信息的方法 public void setName(String name) { this.name = name; } public String getName() { return name; } public void setId(String id) { this.id = id; } public String getId() { return id; } // 学生评分的方法 public float giveRating(float rating) { // 学生进行评分 return rating; } }
In the main program, we can create teacher and student objects and perform teacher grading operations.
public class Main { public static void main(String[] args) { Teacher teacher = new Teacher("张老师", "1001", "数学"); Student student1 = new Student("小明", "001"); Student student2 = new Student("小红", "002"); float rating1 = student1.giveRating(4.5f); float rating2 = student2.giveRating(3.2f); // 将学生评分添加到教师对象中 teacher.calculateRating(); System.out.println("教师姓名:" + teacher.getName()); System.out.println("教师ID:" + teacher.getId()); System.out.println("教师所教科目:" + teacher.getSubject()); System.out.println("教师评分结果:" + teacher.calculateRating()); } }
The above is the design process of a simple teacher scoring system. In practical applications, more complex functional extensions can be carried out according to needs, such as adding administrator classes for scoring management, using databases to store teacher and student information, etc.
By using Java language to design a simple teacher scoring system, teachers' teaching quality can be better evaluated, thereby improving teaching effectiveness. I hope this article will be helpful to readers in designing a teacher scoring system in Java.
The above is the detailed content of How to design a simple teacher scoring system in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
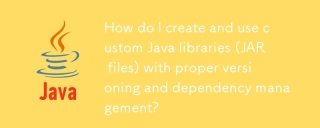
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
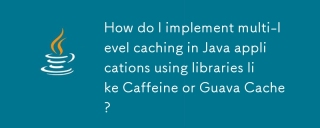
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
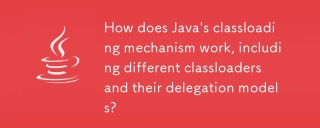
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
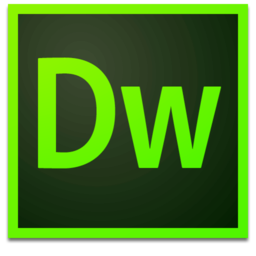
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
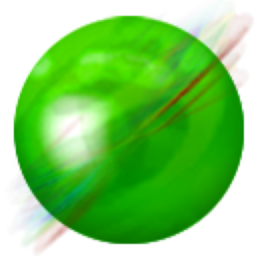
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!