


Best Practices for Laravel Permissions Features: How to Correctly Control User Permissions
Best practices for Laravel permission functions: How to correctly control user permissions requires specific code examples
Introduction:
Laravel is a very powerful software and popular PHP framework that provides many features and tools to help us develop efficient and secure web applications. One important feature is permission control, which restricts user access to different parts of the application based on their roles and permissions.
Proper permission control is a key component of any web application to protect sensitive data and functionality from being accessed by unauthorized users. In this article, we will discuss best practices for permission control in Laravel and provide concrete code examples.
1. Install and set up the authorization function of Laravel
First, we need to install and set up the authorization function in Laravel. We can use Laravel's built-in commands to accomplish this task. Open a terminal and run the following command:
composer require laravel/ui php artisan ui bootstrap --auth
The above command will install Laravel's user interface package and generate the basic authentication and registration controller.
Next, we need to create a table named roles
in the database to save user role information. We can use the migration tool provided by Laravel to accomplish this task. Run the following command:
php artisan make:migration create_roles_table --create=roles
After running the above command, Laravel will generate a new migration file in the database/migrations
folder. Open the file and update the up
method as follows:
use IlluminateDatabaseMigrationsMigration; use IlluminateDatabaseSchemaBlueprint; use IlluminateSupportFacadesSchema; class CreateRolesTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('roles', function (Blueprint $table) { $table->id(); $table->string('name')->unique(); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('roles'); } }
After saving and closing the file, run the following command to execute the migration file:
php artisan migrate
Now, we have Completed the setup of Laravel's authorization function.
2. Define users and role models
Next, we need to define users and role models and establish relationships between them.
First, we need to create a Role
model. Run the following command to generate the model file:
php artisan make:model Role
Next, we need to add the association with the user in the Role
model. Open the app/Role.php
file and add the following code to the class:
public function users() { return $this->hasMany(User::class); }
Next, we need to create the User
model. Run the following command to generate the model file:
php artisan make:model User
Then we need to add the association to the role in the User
model. Open the app/User.php
file and add the following code to the class:
public function role() { return $this->belongsTo(Role::class); }
After saving and closing the file, run the following command in the terminal to ensure User
The model is associated with the users
data table:
composer dump-autoload
We have successfully defined the user and role models and established the relationship between them.
3. Define user access control methods
Now, we need to define some user access control methods to perform permission checks in the application.
First, we need to define a hasPermission
method to check whether the user has specific permissions. Open the app/User.php
file and add the following method in the User
class:
public function hasPermission($permission) { return $this->role->permissions()->where('name', $permission)->exists(); }
Next, we need to define a role
Method to check the user's role. Open the app/User.php
file and add the following method in the User
class:
public function role() { return $this->belongsTo(Role::class); }
After saving and closing the file, we have successfully defined the user Access control methods.
4. Define roles and permissions models
Next, we need to define roles and permissions models and establish relationships between them.
First, we need to create a Permission
model. Run the following command to generate the model file:
php artisan make:model Permission
Next, we need to add the association to the role in the Permission
model. Open the app/Permission.php
file and add the following code to the class:
public function roles() { return $this->belongsToMany(Role::class); }
Next, we need to create a Role
model. Run the following command to generate the model file:
php artisan make:model Role
Then we need to add the association with the permissions in the Role
model. Open the app/Role.php
file and add the following code to the class:
public function permissions() { return $this->belongsToMany(Permission::class); }
After saving and closing the file, run the following command to ensure that the model is associated with the corresponding data table:
composer dump-autoload
We have successfully defined the roles and permissions models and established the relationships between them.
5. Define access control middleware
Finally, we need to define an access control middleware to perform permission checks when accessing restricted routes.
First, we need to register the middleware in the app/Http/Kernel.php
file. Open the file and add the following code to the routeMiddleware
array:
'permission' => AppHttpMiddlewarePermissionMiddleware::class,
Next, we need to create a PermissionMiddleware
class. Run the following command to generate the class file:
php artisan make:middleware PermissionMiddleware
Then, we need to implement the logic in the PermissionMiddleware
middleware class to perform permission checking. Open the app/Http/Middleware/PermissionMiddleware.php
file and add the following code to the class:
public function handle($request, Closure $next, $permission) { $user = Auth::user(); if (!$user->hasPermission($permission)) { abort(403, 'Unauthorized'); } return $next($request); }
以上代码会检查当前用户是否具有特定的权限。如果用户没有该权限,则会返回 HTTP 403 状态码。
保存并关闭文件后,我们已经成功定义了访问控制中间件。
结束语:
通过本文中的步骤,我们已经了解了 Laravel 中权限控制的最佳实践,以及如何正确控制用户权限。我们在代码示例中演示了如何安装和设置 Laravel 的授权功能,定义用户和角色模型,访问控制方法,角色和权限模型,以及访问控制中间件的实现。
通过正确实现权限控制,我们可以保护敏感数据和功能,并根据用户角色和权限来限制其对应用程序中不同部分的访问。这不仅可以增加应用程序的安全性,还可以提供更好的用户体验。
希望本文能够帮助您理解 Laravel 中权限控制的最佳实践,以及如何正确控制用户权限。通过合理应用这些技术,您可以开发出更安全和高效的Web应用程序。
The above is the detailed content of Best Practices for Laravel Permissions Features: How to Correctly Control User Permissions. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
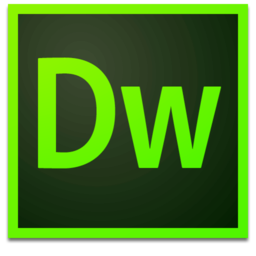
Dreamweaver Mac version
Visual web development tools
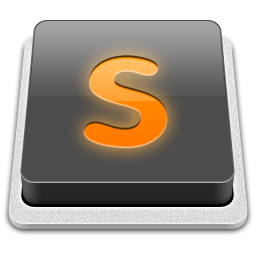
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
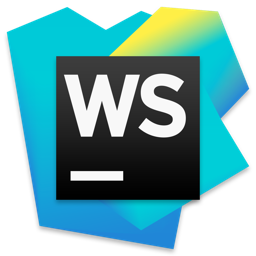
WebStorm Mac version
Useful JavaScript development tools