


How to use Go language to write the user account recharge module in the door-to-door cooking system?
As the takeout market becomes increasingly mature, home cooking has become the first choice for many families for dinner. As a provider of door-to-door cooking services, it is essential to provide reliable user account recharge. This article will introduce how to use Go language to write the user account recharge module in the door-to-door cooking system.
1. Design
When designing the recharge module, we need to consider the following aspects:
- The data structure to be used
In the recharge module, we need to store the user's balance before and after recharging. Therefore, we can use the following data structure:
type Account struct { UserID int Balance float64 }
Here we use UserID
to identify the user, and Balance
to store its account balance.
- Functions to be implemented
In the user recharge module, we need to implement the following functions:
- Query the current user balance
- Recharge
- Deduction
Considering that multiple account operations may be involved in the same transaction, we recommend using transaction management database operations.
2. Implementation
In specific implementation, we can use the ORM framework provided by the Go language, such as GORM.
- Installing GORM
It is very convenient to install GORM in Go language. Just run the following command in the terminal:
go get -u github.com/jinzhu/gorm
- Connect to database
Before using the GORM framework, we need to connect to the database first. We can use MySQL as the database and use MySQL in the Go language. We can use the third-party library go-sql-driver/mysql
.
import ( "fmt" "github.com/jinzhu/gorm" _ "github.com/go-sql-driver/mysql" ) DB, err := gorm.Open("mysql", "username:password@tcp(127.0.0.1:3306)/database_name?charset=utf8mb4&parseTime=True&loc=Local") if err != nil { panic(fmt.Sprintf("database connection error: %v", err)) }
In the above code, we need to replace username
, password
and database_name
with the specific database username, password and database name . Among them, tcp(127.0.0.1:3306)
indicates connecting to the local database, and the port is 3306. charset=utf8mb4&parseTime=True&loc=Local
means using utf8mb4 character encoding, turning on time parsing and local time zone storage.
- Define the data model
In order to better manage the data in the database, we need to define the corresponding data model. In the recharge module, we need to define the account data model.
type Account struct { gorm.Model UserID int Balance float64 }
In this data model, we use gorm.Model
structure embedding to get ID
, CreatedAt
, UpdatedAt# Basic fields such as ## and
DeletedAt. At the same time, we define the
UserID and
Balance fields for this data model.
- Recharge
func Recharge(userID int, amount float64) error { account := Account{} res := DB.Where("user_id = ?", userID).First(&account) if res.Error != nil && res.Error != gorm.ErrRecordNotFound { return res.Error } if res.Error == gorm.ErrRecordNotFound { account.UserID = userID account.Balance = amount res = DB.Create(&account) if res.Error != nil { return res.Error } } else { account.Balance += amount res = DB.Save(&account) if res.Error != nil { return res.Error } } return nil }In this recharge function, we first query the user account through
DB.Where("user_id = ?", userID).First(&account). If the account does not exist, we create a new account; otherwise, we query the account based on the user ID and add the recharge amount
amount to the account balance. Finally, we save the updated data to the database through
DB.Save(&account).
- Deduction
func Deduct(userID int, amount float64) error { if amount <= 0 { return errors.New("invalid deduct amount") } account := Account{} res := DB.Where("user_id = ?", userID).First(&account) if res.Error != nil { return res.Error } if account.Balance-amount < 0 { return errors.New("insufficient balance") } account.Balance -= amount res = DB.Save(&account) if res.Error != nil { return res.Error } return nil }In this deduction function, we first verify the deduction amount
amount to ensure that it is greater than zero. Then, we query the user account and determine whether the balance is sufficient to support the deduction. Finally, we deduct the debit amount from the balance and save the updated data to the database.
The above is the detailed content of How to use Go language to write the user account recharge module in the door-to-door cooking system?. For more information, please follow other related articles on the PHP Chinese website!
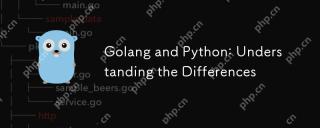
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
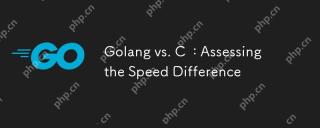
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
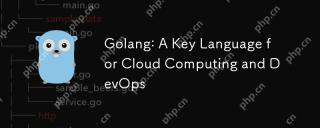
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
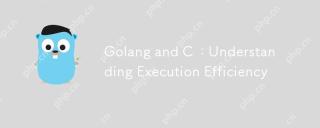
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
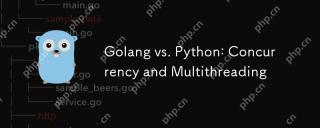
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
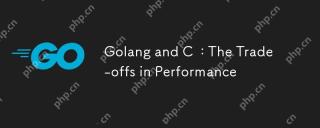
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
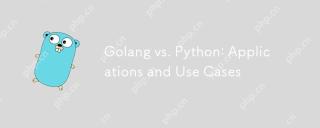
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
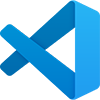
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft