


How to design a maintainable MySQL table structure to implement online shopping cart functionality?
How to design a maintainable MySQL table structure to implement the online shopping cart function?
When designing a maintainable MySQL table structure to implement the online shopping cart function, we need to consider the following aspects: shopping cart information, product information, user information and order information. This article details how to design these tables and provides specific code examples.
- Shopping cart information table (cart)
The shopping cart information table is used to store the items added by the user in the shopping cart. The table contains the following fields: - cart_id: Shopping cart ID as the primary key.
- user_id: User ID, a table used to associate shopping cart information with user information.
- product_id: product ID, a table used to correlate shopping cart information and product information.
- quantity: Product quantity.
- created_at: Creation time.
The following is a code example to create a shopping cart information table:
CREATE TABLE cart ( cart_id INT PRIMARY KEY AUTO_INCREMENT, user_id INT, product_id INT, quantity INT, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (user_id) REFERENCES user(user_id), FOREIGN KEY (product_id) REFERENCES product(product_id) );
- Product information table (product)
The product information table is used to store product information in the online mall. The table contains the following fields: - product_id: product ID as the primary key.
- name: product name.
- price: product price.
- description: product description.
The following is a code example for creating a product information table:
CREATE TABLE product ( product_id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255), price DECIMAL(10, 2), description TEXT );
- User information table (user)
The user information table is used to store user information for the online mall. The table contains the following fields: - user_id: User ID as the primary key.
- name: User name.
- email: User email.
- password: User password.
The following is a code example for creating a user information table:
CREATE TABLE user ( user_id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255), email VARCHAR(255), password VARCHAR(255) );
- Order information table (order)
The order information table is used to store order information submitted by users. The table contains the following fields: - order_id: order ID as the primary key.
- user_id: User ID, a table used to associate order information and user information.
- product_id: product ID, a table used to associate order information and product information.
- quantity: Product quantity.
- total_price: total order price.
- created_at: Creation time.
The following is a code example for creating an order information table:
CREATE TABLE order ( order_id INT PRIMARY KEY AUTO_INCREMENT, user_id INT, product_id INT, quantity INT, total_price DECIMAL(10, 2), created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (user_id) REFERENCES user(user_id), FOREIGN KEY (product_id) REFERENCES product(product_id) );
Through the above table structure design, we can implement a basic online shopping cart function. In actual use, you may need to adjust and expand table fields according to specific needs. Hope this article is helpful to you!
The above is the detailed content of How to design a maintainable MySQL table structure to implement online shopping cart functionality?. For more information, please follow other related articles on the PHP Chinese website!
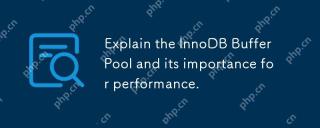
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
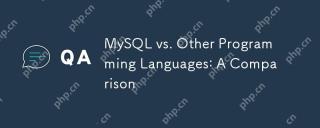
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
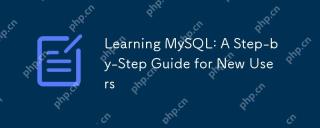
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
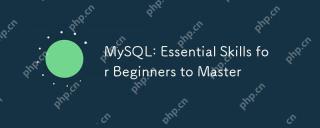
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
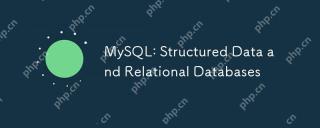
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
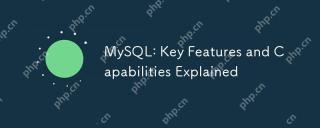
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
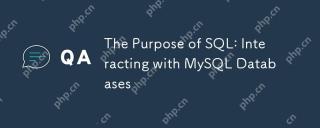
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
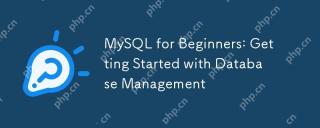
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
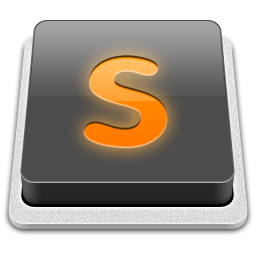
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment