How to use the Hyperf framework for geolocation services
How to use the Hyperf framework for geolocation services, you can connect to Baidu Map API
Geolocation services are widely used in many applications, and the Hyperf framework serves as a The high-performance PHP framework can also be combined with geolocation services to provide geolocation-related functionality to our applications. This article will introduce how to use the Hyperf framework to connect to Baidu Map API for geographical location services, and provide specific code examples.
1. Apply for Baidu Map API Key
First, we need to register a developer account on Baidu Map Open Platform and apply for an API key. After logging in to the Baidu Map Open Platform, find "My Applications" in the console menu, create a new application, and obtain the API key.
2. Install the Hyperf framework
Next, install the Hyperf framework in your development environment. You can install it through the composer command. The specific command is as follows:
$ composer create-project hyperf/hyperf-skeleton project-name
3. Add Baidu Map PHP SDK
The composer.json file of the Hyperf framework has automatically added the dependency of Baidu Map PHP SDK . You just need to run composer command to install it.
$ composer install
4. Create a geographical location service class
Create a geographical location service class and name it LocationService.php. In this class, we will use the reverse geocoding interface provided by Baidu Map API to obtain the detailed address of a location.
<?php namespace AppService; use GuzzleHttpClient; class LocationService { protected $baseUrl = 'http://api.map.baidu.com/reverse_geocoding/v3/'; protected $apiKey; public function __construct($apiKey) { $this->apiKey = $apiKey; } public function getAddress($latitude, $longitude) { $client = new Client(); $response = $client->get($this->baseUrl, [ 'query' => [ 'ak' => $this->apiKey, 'output' => 'json', 'coordtype' => 'wgs84ll', 'location' => "{$latitude},{$longitude}" ] ]); $data = json_decode($response->getBody()->getContents(), true); if (isset($data['result']['formatted_address'])) { return $data['result']['formatted_address']; } return null; } }
In the above code, we created a LocationService class. The constructor accepts an API key as a parameter and assigns it to the member variable $apiKey. The getAddress method accepts a latitude and longitude as parameters, obtains the detailed address of the location by calling the Baidu Map API, and returns the result.
5. Using the geolocation service in the controller
In the Hyperf framework, we can use the geolocation service class we created in the controller to obtain the detailed address of the location. Here is a sample controller code:
<?php namespace AppController; use HyperfDiAnnotationInject; use HyperfHttpServerAnnotationController; use HyperfHttpServerAnnotationGetMapping; use AppServiceLocationService; /** * @Controller */ class LocationController { /** * @Inject * @var LocationService */ protected $locationService; /** * @GetMapping("/location") */ public function index($latitude, $longitude) { $address = $this->locationService->getAddress($latitude, $longitude); return [ 'latitude' => $latitude, 'longitude' => $longitude, 'address' => $address ]; } }
In the above code, we have injected an instance of the LocationService class in the dependency injection annotation of the controller. In the index method, obtain the detailed address of the location by calling the getAddress method of LocationService and return the result.
6. Configure routing
Finally, we need to configure a route in the routing file to access the index method in LocationController. Open the config/routes.php file and add the following code:
<?php use HyperfHttpServerRouterRouter; Router::addRoute(['GET'], '/location', 'AppControllerLocationController@index');
Now, we can get the detailed address of a location by accessing http://localhost:9501/location?latitude=latitude&longitude=longitude.
Conclusion
This article introduces how to use the Hyperf framework to connect to Baidu Map API for geographical location services, and provides specific code examples. By reading this article, you can learn how to use the geolocation service in the Hyperf framework and extend this service according to your needs. Hope this article is helpful to you!
The above is the detailed content of How to use the Hyperf framework for geolocation services. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
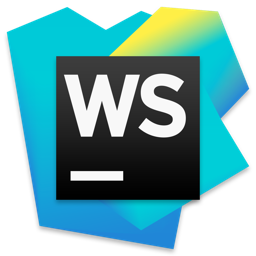
WebStorm Mac version
Useful JavaScript development tools
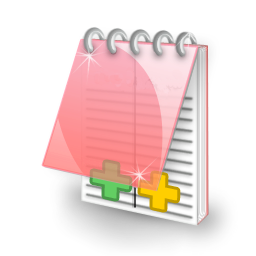
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!