How to implement lazy loading of tab content using JavaScript?
How to use JavaScript to implement lazy loading of tab content?
In modern web design, tabs are a common interface element, which can easily switch between different contents and give users a better experience. However, when a large amount of content is loaded in the tab, the page loading speed will slow down, affecting the user's access experience. In order to solve this problem, we can use JavaScript to implement the lazy loading function of tab content, and the corresponding content will only be loaded when the user clicks the relevant tab.
The steps to implement the lazy loading function of tab content are as follows:
- Create the HTML structure of the tab
First, we need to create the HTML structure of the tab. Typically, a tab consists of a menu section and a content section. The menu section contains multiple tab titles, and the content section contains the corresponding content.
<div class="tabs"> <div class="tab-menu"> <a href="#" class="tab-link">Tab 1</a> <a href="#" class="tab-link">Tab 2</a> <a href="#" class="tab-link">Tab 3</a> </div> <div class="tab-content"> <div class="tab-item">Content 1</div> <div class="tab-item">Content 2</div> <div class="tab-item">Content 3</div> </div> </div>
- Add lazy loading JavaScript code
Next, we can add JavaScript code to implement the lazy loading function of the tab content. We need to listen to the click event of the tab title and load the corresponding content when clicked.
// 获取选项卡标题和内容元素 var tabLinks = document.querySelectorAll('.tab-link'); var tabItems = document.querySelectorAll('.tab-item'); // 遍历选项卡标题,为每个标题添加点击事件监听器 for(var i = 0; i < tabLinks.length; i++) { tabLinks[i].addEventListener('click', function(e) { e.preventDefault(); // 阻止默认点击事件 // 获取点击的选项卡标题的索引 var index = Array.prototype.indexOf.call(tabLinks, this); // 遍历所有选项卡内容,隐藏非当前选项卡的内容 for(var j = 0; j < tabItems.length; j++) { if(j !== index) { tabItems[j].style.display = 'none'; } } // 显示当前选项卡的内容 tabItems[index].style.display = 'block'; }); }
- Use CSS for style control
Finally, we can use CSS to style the tab for better presentation.
.tab-menu { display: flex; } .tab-link { margin-right: 10px; padding: 5px; background-color: lightgray; cursor: pointer; } .tab-item { display: none; padding: 10px; background-color: white; }
Through the above three steps, we can use JavaScript to implement the lazy loading function of tab content. When the user clicks on the tab title, the corresponding content will be loaded and displayed, avoiding performance issues when the page is loaded. In this way, we can improve the user experience and optimize the loading speed of web pages.
It is worth noting that the above sample code is for reference only, and may need to be appropriately adjusted and expanded according to specific needs in actual applications.
The above is the detailed content of How to implement lazy loading of tab content using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
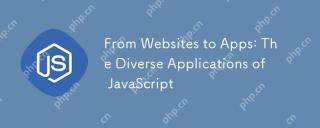
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
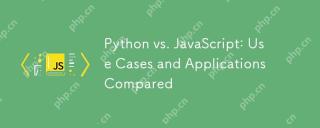
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
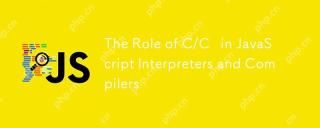
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
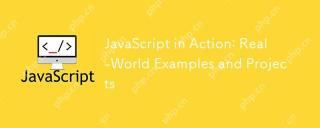
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
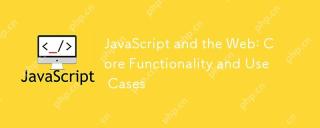
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
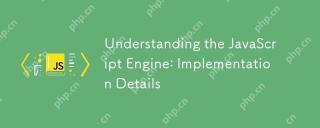
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
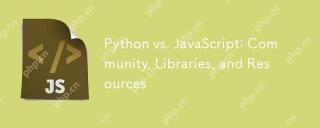
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
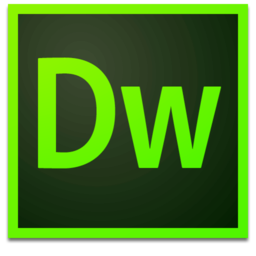
Dreamweaver Mac version
Visual web development tools
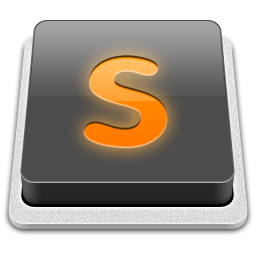
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
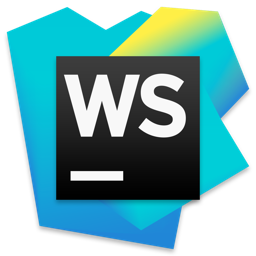
WebStorm Mac version
Useful JavaScript development tools