How to use Hyperf framework for authentication
How to use the Hyperf framework for authentication
In modern web applications, user authentication is a very important feature. To protect sensitive information and ensure application security, authentication ensures that only authenticated users can access restricted resources.
Hyperf is a high-performance PHP framework based on Swoole, which provides many modern and efficient functions and tools. In the Hyperf framework, we can use multiple methods to implement identity authentication. Two of the commonly used methods will be introduced below.
- Using JWT (JSON Web Token)
JWT is an open standard (RFC 7519) that defines a concise, self-contained method for Securely transmit information between communicating parties. In the Hyperf framework, we can use the lcobucci/jwt
extension library to achieve JWT generation and verification.
First, we need to add the lcobucci/jwt
library dependencies in the composer.json file:
"require": { "lcobucci/jwt": "^3.4" }
Then execute the composer update
command to install Dependencies.
Next, we can create a JwtAuthenticator
class for generating and validating JWT:
<?php declare(strict_types=1); namespace AppAuth; use HyperfExtAuthAuthenticatable; use HyperfExtAuthContractsAuthenticatorInterface; use LcobucciJWTConfiguration; use LcobucciJWTToken; class JwtAuthenticator implements AuthenticatorInterface { private Configuration $configuration; public function __construct(Configuration $configuration) { $this->configuration = $configuration; } public function validateToken(string $token): bool { $parsedToken = $this->configuration->parser()->parse($token); $isVerified = $this->configuration->validator()->validate($parsedToken, ...$this->configuration->validationConstraints()); return $isVerified; } public function generateToken(Authenticatable $user): string { $builder = $this->configuration->createBuilder(); $builder->issuedBy('your_issuer') ->issuedAt(new DateTimeImmutable()) ->expiresAt((new DateTimeImmutable())->modify('+1 hour')) ->withClaim('sub', (string) $user->getAuthIdentifier()); $token = $builder->getToken($this->configuration->signer(), $this->configuration->signingKey()); return $token->toString(); } }
Then, we need to register it in the container of the Hyperf framework JwtAuthenticator
class:
HyperfUtilsApplicationContext::getContainer()->define(AppAuthJwtAuthenticator::class, function (PsrContainerContainerInterface $container) { $configuration = LcobucciJWTConfiguration::forAsymmetricSigner( new LcobucciJWTSignerRsaSha256(), LcobucciJWTSignerKeyLocalFileReference::file('path/to/private/key.pem') ); return new AppAuthJwtAuthenticator($configuration); });
Finally, in a route or controller method that requires authentication, we can use the JwtAuthenticator
class to verify the user's JWT:
<?php declare(strict_types=1); namespace AppController; use AppAuthJwtAuthenticator; use HyperfHttpServerAnnotationController; use HyperfHttpServerAnnotationRequestMapping; /** * @Controller(prefix="/api") */ class ApiController { private JwtAuthenticator $authenticator; public function __construct(JwtAuthenticator $authenticator) { $this->authenticator = $authenticator; } /** * @RequestMapping(path="profile", methods="GET") */ public function profile() { $token = $this->request->getHeader('Authorization')[0] ?? ''; $token = str_replace('Bearer ', '', $token); if (!$this->authenticator->validateToken($token)) { // Token验证失败,返回错误响应 return 'Unauthorized'; } // Token验证成功,返回用户信息 return $this->authenticator->getUserByToken($token); } }
- Using Session
In addition to JWT authentication, the Hyperf framework also supports the use of Session for identity authentication. We can enable the Session authentication function through the configuration file.
First, we need to make the corresponding configuration in the configuration file config/autoload/session.php
, for example:
return [ 'handler' => [ 'class' => HyperfRedisSessionHandler::class, 'options' => [ 'pool' => 'default', ], ], ];
Then, in the routing or control that requires authentication In the server method, we can use the AuthManager
class provided by the Hyperf framework to authenticate the user's Session:
<?php declare(strict_types=1); namespace AppController; use HyperfHttpServerAnnotationController; use HyperfHttpServerAnnotationRequestMapping; use HyperfExtAuthContractsAuthManagerInterface; /** * @Controller(prefix="/api") */ class ApiController { private AuthManagerInterface $authManager; public function __construct(AuthManagerInterface $authManager) { $this->authManager = $authManager; } /** * @RequestMapping(path="profile", methods="GET") */ public function profile() { if (!$this->authManager->check()) { // 用户未登录,返回错误响应 return 'Unauthorized'; } // 用户已登录,返回用户信息 return $this->authManager->user(); } }
In the above code, the AuthManagerInterface
interface provides many Authentication and user operation methods can be called according to actual needs.
The above are two common methods for identity authentication using the Hyperf framework, which implement user authentication through JWT or Session. Based on actual needs and project characteristics, select appropriate methods to ensure application security and user experience. In actual development, you can learn more about advanced usage and best practices based on the documentation and sample code provided by the framework.
The above is the detailed content of How to use Hyperf framework for authentication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
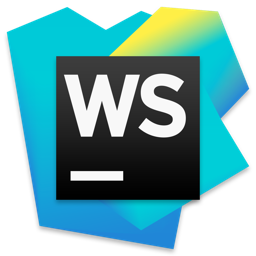
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
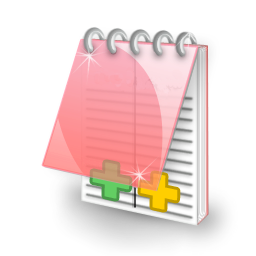
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software