


How the uniapp application implements music ratings and song recommendations
How the uni-app application implements music ratings and song recommendations
Introduction:
With the popularity and development of music, more and more users are beginning to use music player to enjoy music. However, how to make it more convenient for users to rate and recommend their favorite songs has become a problem. This article will introduce how to use the uni-app application to implement music ratings and song recommendations, and provide specific code examples.
- Implementation of music rating function
The music rating function allows users to rate songs they like or dislike, so that the system can analyze the user's preferences and make recommendations based on the ratings. In the uni-app application, you can use local storage or cloud storage to save user rating records for songs.
First, we need to create a data storage object in the application to save the user's rating records. You can use the local storage function provided by uni-app, such as using localStorage or using a cloud storage service.
The following is a sample code using local storage:
// 存储歌曲评分的数组 let songRatings = [] // 获取本地存储中的评分记录 const getSongRatings = () => { const ratings = localStorage.getItem('songRatings') if (ratings) { songRatings = JSON.parse(ratings) } } // 存储歌曲评分记录到本地存储 const setSongRating = (songId, rating) => { songRatings.push({ songId, rating }) localStorage.setItem('songRatings', JSON.stringify(songRatings)) }
When the user rates a song, call the setSongRating
method to save the rating record to local storage.
In addition, in order to conveniently obtain the user's rating records in the application, you can write a getSongRatings
method to obtain the rating records from local storage.
- Implementation of the song recommendation function
The song recommendation function can analyze the user's preferences based on the user's rating records, and then recommend songs that match their preferences for the user. In the uni-app application, we can use algorithms or machine learning methods to recommend songs.
Here is a simple sample code that illustrates how to record recommended songs based on user ratings:
// 根据评分记录推荐歌曲 const recommendSongs = () => { // 从本地存储中获取评分记录 getSongRatings() // 进行歌曲推荐算法 // 此处可以使用机器学习或其他算法来进行推荐 // 假设推荐结果为一个歌曲数组 const recommendedSongs = [ { id: 1, name: 'Song 1' }, { id: 2, name: 'Song 2' }, { id: 3, name: 'Song 3' } ] // 返回推荐的歌曲 return recommendedSongs }
In the above code, by calling the getSongRatings
method from Get rating records from local storage. The rating records can then be analyzed using machine learning or other algorithms and recommendations derived.
- Code example in uni-app application
For ease of understanding, the following is a code example that uses uni-app to implement music ratings and song recommendations:
<template> <view> <!-- 歌曲列表 --> <view v-for="song in songs" :key="song.id" @click="rateSong(song.id)"> <!-- 歌曲名称 --> <text>{{ song.name }}</text> <!-- 歌曲评分 --> <star-rating :rating="getSongRating(song.id)" :max-rating="5" /> </view> <!-- 推荐歌曲 --> <view v-if="recommendedSongs.length > 0"> <text>推荐歌曲:</text> <view v-for="song in recommendedSongs" :key="song.id"> <text>{{ song.name }}</text> </view> </view> </view> </template> <script> import { setSongRating, recommendSongs, getSongRatings } from '@/utils/songUtil' export default { data() { return { songs: [ { id: 1, name: 'Song 1' }, { id: 2, name: 'Song 2' }, { id: 3, name: 'Song 3' } ], recommendedSongs: [] } }, methods: { rateSong(songId, rating) { // 设置歌曲评分 setSongRating(songId, rating) // 推荐歌曲 this.recommendedSongs = recommendSongs() }, getSongRating(songId) { // 获取歌曲评分 const ratings = getSongRatings() const songRating = ratings.find(rating => rating.songId === songId) return songRating ? songRating.rating : 0 } } } </script>
In the above code, the uni-app component star-rating
is used to display the song's rating. After the user clicks on the song, call the rateSong
method to set the song rating and update the recommended songs.
Conclusion:
By using the uni-app application, we can implement music rating and song recommendation functions. Users can easily rate songs and get personalized song recommendations based on rating records. The code examples provided above can help developers quickly implement this function. Of course, the specific implementation of the song recommendation function can be adjusted and optimized according to needs.
The above is the detailed content of How the uniapp application implements music ratings and song recommendations. For more information, please follow other related articles on the PHP Chinese website!
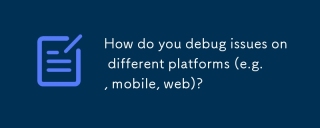
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
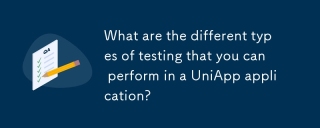
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
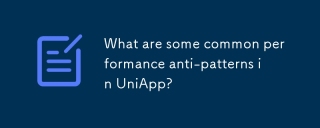
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
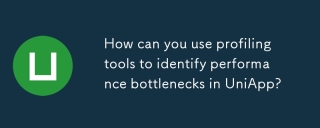
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
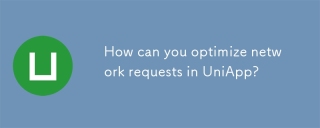
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
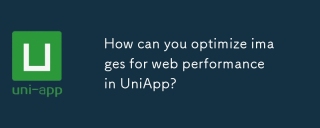
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.