How to implement scrolling switching effect of images in JavaScript?
JavaScript How to achieve the scrolling switching effect of images?
In modern web design, image scrolling switching effect is one of the commonly used design elements, which can add dynamics and vividness to the web page. JavaScript, as a commonly used scripting language, can help us achieve this effect. In this article, I will introduce a method to use JavaScript to achieve image scrolling switching effect, and provide corresponding code examples.
First, we need to prepare an HTML structure for displaying images. The specific code is as follows:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <style> .slider { width: 600px; height: 300px; overflow: hidden; position: relative; } .slider img { width: 600px; height: 300px; position: absolute; transition: transform 0.5s ease-in-out; } </style> </head> <body> <div class="slider"> <img src="/static/imghwm/default1.png" data-src="image1.jpg" class="lazy" alt="Image 1"> <img src="/static/imghwm/default1.png" data-src="image2.jpg" class="lazy" alt="Image 2"> <img src="/static/imghwm/default1.png" data-src="image3.jpg" class="lazy" alt="Image 3"> </div> <script src="slider.js"></script> </body> </html>
In the above code, we created a div element named "slider" with a width of 600px and a height of 300px. The div element has the overflow:hidden attribute, which hides images that exceed the border. And we set the width and height of the image in CSS, as well as the absolute positioning properties of the image, so that they overlap. In addition, we also added a transition effect to the image so that there will be a smooth animation effect when the image switches.
Next, we need to use JavaScript to achieve the image switching effect. We can create a JavaScript file named "slider.js" and copy and paste the following code into the file:
window.addEventListener('DOMContentLoaded', function () { var slider = document.querySelector('.slider'); var images = slider.getElementsByTagName('img'); var currentIndex = 0; var intervalId; function changeImage() { currentIndex = (currentIndex + 1) % images.length; for (var i = 0; i < images.length; i++) { images[i].style.transform = 'translateX(' + (i - currentIndex) * 100 + '%)'; } } intervalId = setInterval(changeImage, 2000); });
In the above code, we first obtain the div element with the "slider" class name and all img elements under this element. Then, we define a variable "currentIndex" to track the currently displayed image index, and initialize intervalId to the ID of a timer.
Next, we created a function called "changeImage" for switching images. In this function, we use a loop to traverse the images array and set a transform attribute for each image to achieve the scrolling effect of the image. By setting the translateX value to (i - currentIndex) * 100%, we can keep the currently displayed image in the middle while other images scroll to the left and right.
Finally, we use the setInterval function to regularly call the changeImage function to achieve automatic image switching. In this example, we switch between pictures at intervals of 2 seconds.
After completing the above code, we link the JavaScript file to the HTML file, and then we can view the image scrolling switching effect in the browser.
To sum up, by using JavaScript, we can easily achieve the scrolling switching effect of images. By getting the elements in the HTML structure and using CSS styles and JavaScript functions, we can achieve smooth switching of images. I hope this article can help readers understand and master this implementation.
The above is the detailed content of How to implement scrolling switching effect of images in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
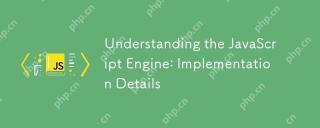
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
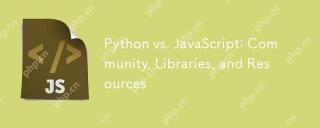
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
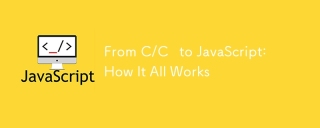
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
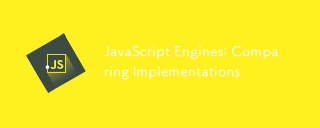
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
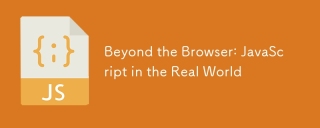
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
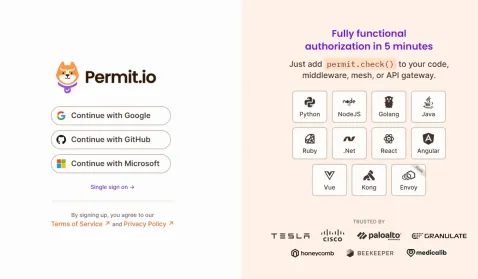
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
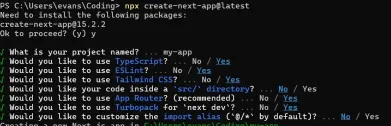
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
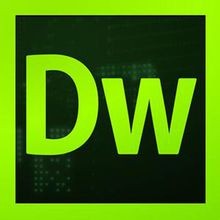
Dreamweaver CS6
Visual web development tools
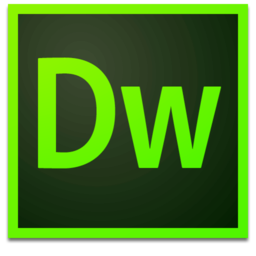
Dreamweaver Mac version
Visual web development tools