How to implement workplace recruitment and talent management in uniapp
UniApp is a cross-platform application development framework based on Vue.js, which can help developers quickly build multi-terminal applications. In workplace recruitment and talent management applications, we can use UniApp to implement various functions, such as talent recruitment, resume management, job postings, and interview arrangements. The following will introduce the specific implementation method and provide code examples.
1. Page construction
First we need to create relevant pages, including homepage, job list page, resume list page, job details page and resume details page, etc. Through page writing in uniapp, page display and navigation are realized.
2. Data interaction and interface request
- Create an API folder to store files related to the backend interface.
- Create job.js and resume.js files in the API folder, which are used to handle job-related and resume-related interface requests respectively.
- In the job.js file, write the interface request function to obtain the job list and job details. An example is as follows:
// job.js import request from '@/utils/request' // 获取职位列表 export function getJobList() { return request({ url: '/job/list', method: 'get' }) } // 获取职位详情 export function getJobDetail(id) { return request({ url: '/job/detail', method: 'get', params: { id } }) }
- In the resume.js file, write the interface request function to obtain the resume list and resume details. An example is as follows:
// resume.js import request from '@/utils/request' // 获取简历列表 export function getResumeList() { return request({ url: '/resume/list', method: 'get' }) } // 获取简历详情 export function getResumeDetail(id) { return request({ url: '/resume/detail', method: 'get', params: { id } }) }
- Create a request.js file in the utils folder to encapsulate request functions, error handling, etc. An example is as follows:
// request.js import axios from 'axios' const service = axios.create({ baseURL: process.env.BASE_API, // 根据实际情况配置baseURL timeout: 5000 // 请求超时时间 }) service.interceptors.response.use( response => { const res = response.data if (res.code !== 200) { // 处理错误信息 return Promise.reject(new Error(res.message || 'Error')) } else { return res } }, error => { return Promise.reject(error) } ) export default service
3. Page data display
- In the job list page, use the
getJobList
interface to request the job list data, and displayed on the page. An example is as follows:
// job/list.vue <template> <view> <view v-for="(job, index) in jobList" :key="index"> <text>{{ job.title }}</text> </view> </view> </template> <script> import { getJobList } from '@/api/job' export default { data() { return { jobList: [] } }, mounted() { this.getJobListData() }, methods: { getJobListData() { getJobList().then(res => { this.jobList = res.data }) } } } </script>
- In the job details page, use the
getJobDetail
interface to request the job details data and display it on the page. Examples are as follows:
// job/detail.vue <template> <view> <text>{{ job.title }}</text> <text>{{ job.description }}</text> </view> </template> <script> import { getJobDetail } from '@/api/job' export default { data() { return { job: {} } }, mounted() { this.getJobDetailData() }, methods: { getJobDetailData() { const id = this.$route.params.id getJobDetail(id).then(res => { this.job = res.data }) } } } </script>
- The implementation of resume list page and resume details page is similar to that of job list page and job details page.
4. Implementation of other functions
In addition to page data display, other functions can also be implemented in the application, such as resume uploading, job postings, and interview arrangements. By combining the components and APIs provided by uniapp, we can quickly implement these functions.
To sum up, through UniApp combined with interface requests and page construction, we can realize various functions in workplace recruitment and talent management applications, and provide users with a convenient recruitment and talent management experience. Hope the above content is helpful to you.
The above is the detailed content of How to implement workplace recruitment and talent management in uniapp. For more information, please follow other related articles on the PHP Chinese website!
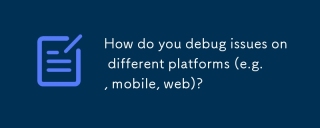
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
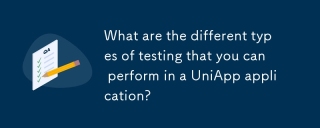
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
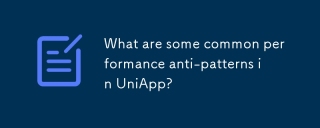
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
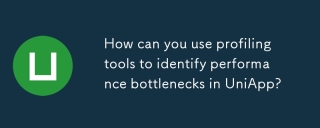
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
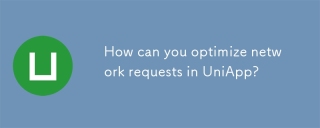
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
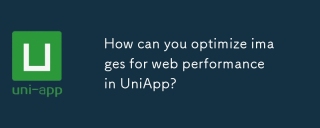
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
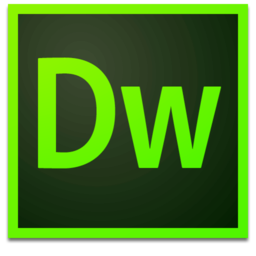
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
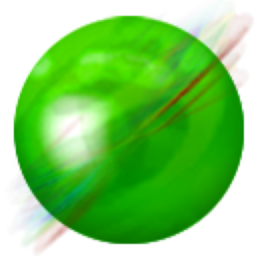
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!