How to handle null value case using Optional function in Java
How to use the Optional function to handle null values in Java
In Java programming, we often encounter situations where null values are handled. Null pointer exception is a common error. To avoid this situation, Java 8 introduced the Optional class to handle null value situations. The Optional class is a container class that can contain a non-empty value or no value.
Using the Optional class, we can handle null value situations more elegantly and avoid null pointer exceptions. The following will introduce how to use the Optional function in Java to handle null value situations, and provide specific code examples.
- Create Optional object
When using the Optional class, you first need to create an Optional object. An Optional object can be created by calling the static methods of the Optional class. There are three commonly used ways to create Optional objects:
- Using the of method: the of method receives a non-null value as a parameter and returns an Optional object containing the value. If the value passed in is null, a NullPointerException will be thrown.
- Use ofNullable method: ofNullable method receives a value as a parameter and returns an Optional object containing the value. If the value passed in is null, an empty Optional object is returned, namely Optional.empty().
- Use empty method: empty method returns an empty Optional object.
The following is a code example for creating an Optional object:
Optional<String> nonEmptyOptional = Optional.of("Hello"); Optional<String> nullableOptional = Optional.ofNullable(null); Optional<String> emptyOptional = Optional.empty();
- Determine whether the Optional object contains a value
When dealing with Optional objects, we often need to determine whether the Optional object Contains a non-null value. You can determine whether the Optional object contains a value by calling the isPresent method. The isPresent method returns a Boolean value, true if the Optional object contains a non-null value, false otherwise.
The following is a code example to determine whether the Optional object contains a value:
Optional<String> optional = Optional.ofNullable("Hello"); if (optional.isPresent()) { System.out.println("Optional对象包含值"); } else { System.out.println("Optional对象不包含值"); }
- Get the value of the Optional object
If the Optional object contains a non-empty value, we The value can be obtained by calling the get method. The get method returns the value contained in the Optional object. If the Optional object is empty, a NoSuchElementException will be thrown.
In order to avoid throwing NoSuchElementException, we can use the isPresent method to determine whether the Optional object contains a non-null value, and make a judgment before calling the get method to obtain the value.
The following is a code example to get the value of the Optional object:
Optional<String> optional = Optional.ofNullable("Hello"); if (optional.isPresent()) { String value = optional.get(); System.out.println("获取到的值为:" + value); } else { System.out.println("Optional对象不包含值"); }
However, using the get method to get the value is an unsafe way, because if the Optional object is empty, it will throw abnormal. Therefore, a better approach is to use the ifPresent method, which receives a Consumer function interface as a parameter. If the Optional object contains a non-null value, the function interface will be called to process the value.
The following is a code example of using the ifPresent method to obtain the value of an Optional object:
Optional<String> optional = Optional.ofNullable("Hello"); optional.ifPresent(value -> System.out.println("获取到的值为:" + value));
- Use the orElse method to set the default value
When dealing with Optional objects, we often need to set an The default value is used when the Optional object is empty. You can use the orElse method to set a default value. The orElse method receives a value as a parameter and returns the value when the Optional object is empty.
The following is a code example for using the orElse method to set a default value:
Optional<String> optional = Optional.ofNullable(null); String value = optional.orElse("默认值"); System.out.println("获取到的值为:" + value);
- Using the orElseGet method to set a default value
In addition to using the orElse method to set a default value, You can also use the orElseGet method. Compared with the orElse method, the orElseGet method receives a Supplier function interface as a parameter, which defines a get method to return a default value. When the Optional object is empty, this function interface will be called to obtain the default value.
The following is a code example that uses the orElseGet method to set a default value:
Optional<String> optional = Optional.ofNullable(null); String value = optional.orElseGet(() -> { // 通过一些逻辑来计算默认值 return "计算得到的默认值"; }); System.out.println("获取到的值为:" + value);
- Use the map method to convert the value of the Optional object
When dealing with Optional objects, we often Some operations need to be performed on the values in the Optional object, such as conversion, filtering, etc. You can use the map method to convert values in Optional objects. The map method receives a Function function interface as a parameter, which defines an apply method for converting the value in the Optional object.
The following is a code example that uses the map method to convert the value of an Optional object:
Optional<String> optional = Optional.ofNullable("Hello"); Optional<String> transformedOptional = optional.map(value -> value.toUpperCase()); transformedOptional.ifPresent(value -> System.out.println("转换后的值为:" + value));
- Use the flatMap method to convert an Optional object
When dealing with Optional objects, sometimes we Further operations are required on the value in the Optional object, such as obtaining a new Optional object based on the value in the Optional object. You can use the flatMap method to achieve this functionality. The flatMap method receives a Function function interface as a parameter, which defines an apply method to convert the value in the Optional object and return a new Optional object.
The following is a code example of using the flatMap method to convert an Optional object:
Optional<String> optional = Optional.ofNullable("Hello"); Optional<String> flatMappedOptional = optional.flatMap(value -> { if (value.equals("Hello")) { return Optional.of("World"); } else { return Optional.empty(); } }); flatMappedOptional.ifPresent(value -> System.out.println("转换后的值为:" + value));
总结
在Java编程中,处理空值情况是一个非常常见的需求。使用Optional函数可以更加优雅地处理空值情况,避免出现空指针异常。本文介绍了How to handle null value case using Optional function in Java,并提供了具体的代码示例。通过学习和使用Optional函数,可以使我们的代码更加安全和健壮。
The above is the detailed content of How to handle null value case using Optional function in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
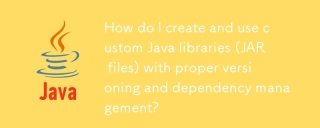
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
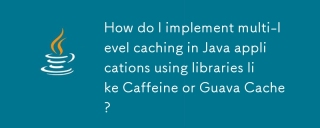
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
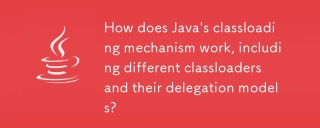
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
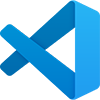
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.