


How to implement multi-threaded programming using multi-threaded functions in Java
在Java中,多线程编程是一种重要的技术,可以提高程序的并发性和性能。在这篇文章中,我们将探讨如何使用多线程函数来实现多线程编程,并给出具体的代码示例。
- 创建多线程对象
在Java中,我们可以通过继承Thread类或实现Runnable接口来创建多线程对象。下面是使用继承Thread类的示例代码:
public class MyThread extends Thread { public void run() { // 线程执行的代码逻辑 } public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); } }
在这个示例中,我们继承了Thread类,并重写了其中的run()方法,用来定义线程实际执行的代码逻辑。在main()方法中,我们创建了一个MyThread对象,并通过调用start()方法来启动线程。
- 实现线程的同步
在多线程编程中,线程的同步是一个重要的问题。如果多个线程同时对共享资源进行读写操作,会导致数据不一致的问题。Java提供了synchronized关键字和Lock接口来实现线程的同步。下面是使用synchronized关键字的示例代码:
public class MyThread extends Thread { private static int counter = 0; public void run() { synchronized (MyThread.class) { for (int i = 0; i < 1000; i++) { counter++; } } } public static void main(String[] args) throws InterruptedException { int numThreads = 10; MyThread[] threads = new MyThread[numThreads]; for (int i = 0; i < numThreads; i++) { threads[i] = new MyThread(); threads[i].start(); } for (int i = 0; i < numThreads; i++) { threads[i].join(); } System.out.println("Counter: " + counter); } }
在这个示例中,我们创建了10个线程,每个线程的run()方法中都通过synchronized关键字来对counter变量进行同步操作。通过join()方法等待所有线程执行完毕,并打印最终的计数器值。
- 使用线程池
在实际的多线程编程中,通常会使用线程池来管理线程的创建和销毁。Java提供了Executor框架来实现线程池管理。下面是使用线程池的示例代码:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class MyThread implements Runnable { private int id; public MyThread(int id) { this.id = id; } public void run() { // 线程执行的代码逻辑 System.out.println("Thread " + id + " is running"); } public static void main(String[] args) { int numThreads = 10; ExecutorService executor = Executors.newFixedThreadPool(numThreads); for (int i = 0; i < numThreads; i++) { executor.execute(new MyThread(i)); } executor.shutdown(); } }
在这个示例中,我们使用了Executors类的newFixedThreadPool()方法来创建一个固定大小的线程池。通过execute()方法提交任务给线程池执行,并在最后调用shutdown()方法关闭线程池。
总结
本文介绍了How to implement multi-threaded programming using multi-threaded functions in Java,包括创建多线程对象、实现线程的同步以及使用线程池。多线程编程是一种强大的工具,可以提高程序的并发性和性能。但在实际应用过程中,需要注意线程同步和资源共享的问题,以确保程序的正确性和稳定性。希望本文能对读者理解和应用多线程编程提供帮助。
The above is the detailed content of How to implement multi-threaded programming using multi-threaded functions in Java. For more information, please follow other related articles on the PHP Chinese website!
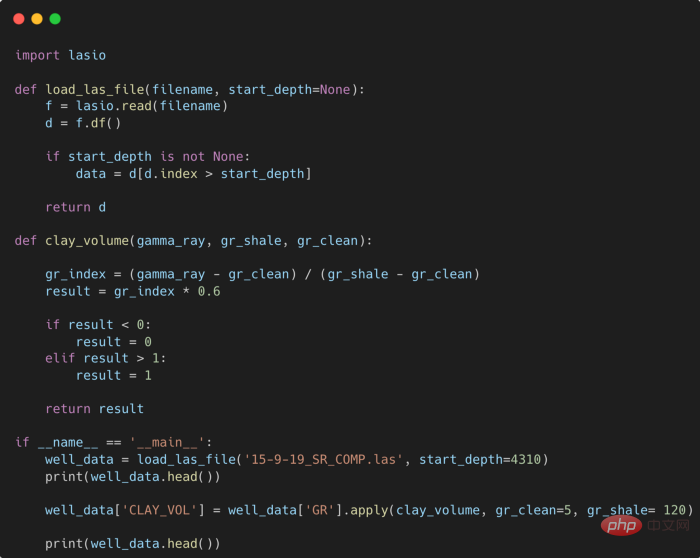
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
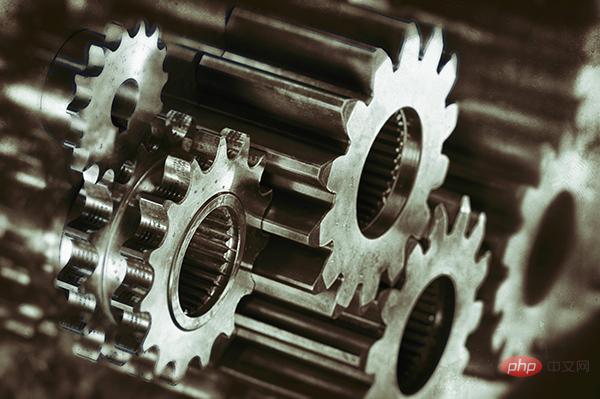
连续分级概率评分(Continuous Ranked Probability Score, CRPS)或“连续概率排位分数”是一个函数或统计量,可以将分布预测与真实值进行比较。机器学习工作流程的一个重要部分是模型评估。这个过程本身可以被认为是常识:将数据分成训练集和测试集,在训练集上训练模型,并使用评分函数评估其在测试集上的性能。评分函数(或度量)是将真实值及其预测映射到一个单一且可比较的值 [1]。例如,对于连续预测可以使用 RMSE、MAE、MAPE 或 R 平方等评分函数。如果预测不是逐点
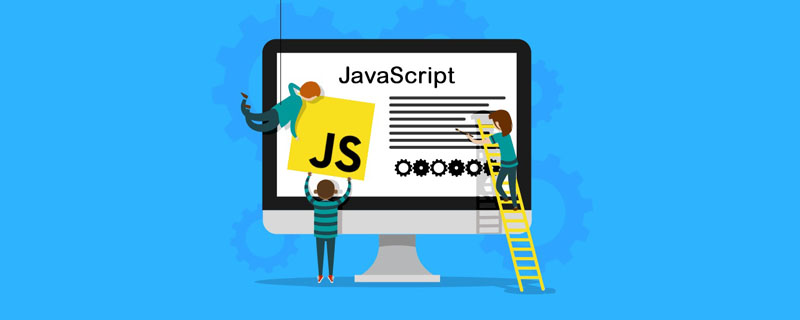
js是弱类型语言,不能像C#那样使用param关键字来声明形参是一个可变参数。那么js中,如何实现这种可变参数呢?下面本篇文章就来聊聊JavaScript函数可变参数的实现方法,希望对大家有所帮助!
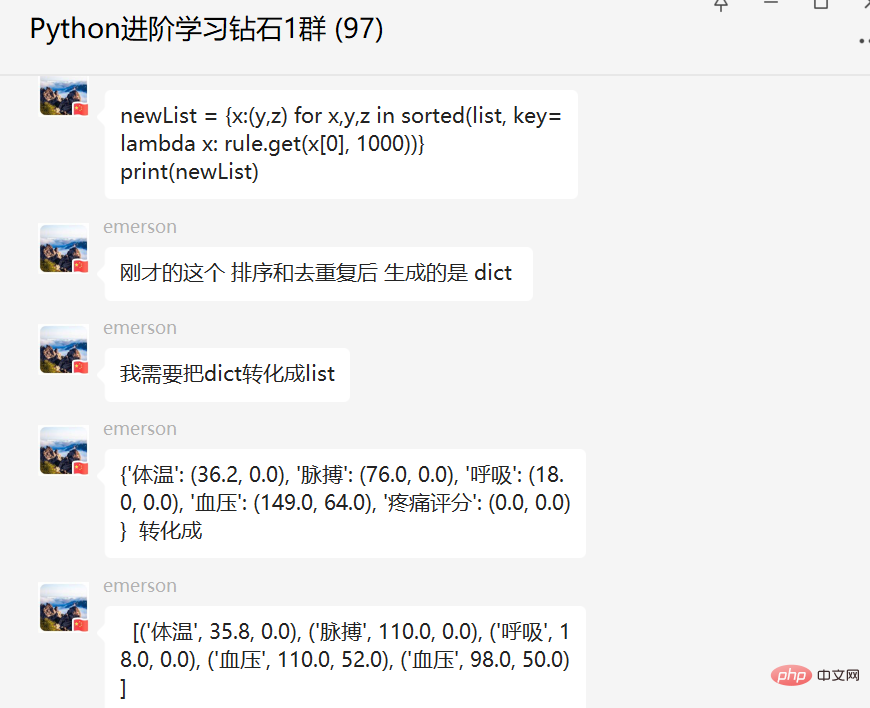
一、前言前几天在Python钻石交流群有个叫【emerson】的粉丝问了一个Python排序的问题,这里拿出来给大家分享下,一起学习下。其实这里【瑜亮老师】、【布达佩斯的永恒】等人讲了很多,只不过对于基础不太好的小伙伴们来说,还是有点难的。不过在实际应用中内置函数sorted()用的还是蛮多的,这里也单独拿出来讲一下,希望下次再有小伙伴遇到的时候,可以不慌。二、基础用法内置函数sorted()可以用来做排序,基础的用法很简单,看个例子,如下所示。lst=[3,28,18,29,2,5,88
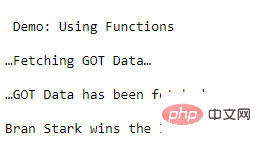
Python 中的 main 函数充当程序的执行点,在 Python 编程中定义 main 函数是启动程序执行的必要条件,不过它仅在程序直接运行时才执行,而在作为模块导入时不会执行。要了解有关 Python main 函数的更多信息,我们将从如下几点逐步学习:什么是 Python 函数Python 中 main 函数的功能是什么一个基本的 Python main() 是怎样的Python 执行模式Let’s get started什么是 Python 函数相信很多小伙伴对函数都不陌生了,函数是可

好嘞,今天我们继续剖析下Python里的类。[[441842]]先前我们定义类的时候,使用到了构造函数,在Python里的构造函数书写比较特殊,他是一个特殊的函数__init__,其实在类里,除了构造函数还有很多其他格式为__XXX__的函数,另外也有一些__xx__的属性。下面我们一一说下:构造函数Python里所有类的构造函数都是__init__,其中根据我们的需求,构造函数又分为有参构造函数和无惨构造函数。如果当前没有定义构造函数,那么系统会自动生成一个无参空的构造函数。例如:在有继承关系
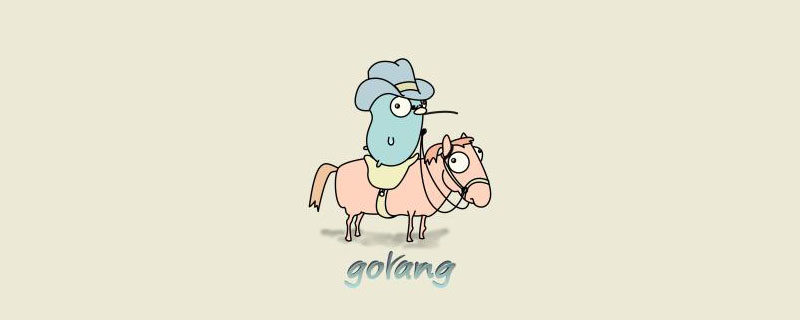
形参变量在未出现函数调用时并不占用内存,只在调用时才占用,调用结束后将释放内存。形参全称“形式参数”,是函数定义时使用的参数;但函数定义时参数是没有任实际何数据的,因而在函数被调用前没有为形参分配内存,其作用是说明自变量的类型和形态以及在过程中的作用。
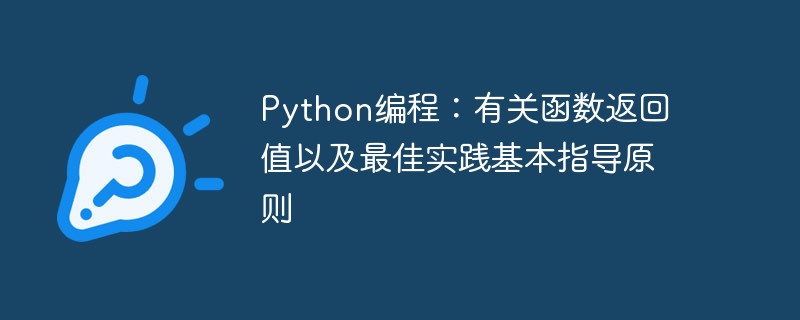
本篇内容作为以函数为主题的最后一篇,来介绍一下函数返回值以及编写函数的一些基本的最佳实践指导原则。函数输出:返回值函数的返回值是Python领先于竞争对手的东西之一。在大多数其他语言中,函数通常只允许返回一个对象,但是在Python中,你可以返回一个元组——这意味着可以返回任何你想要的东西。这个特性允许程序员编写用其他语言编写的软件要困难得多,或者肯定会更加乏味。我们已经说过,要从函数返回一些东西,我们需要使用return语句,后面跟着我们想要返回的东西。函数体中可以根据需要有多个返回语句。另一


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
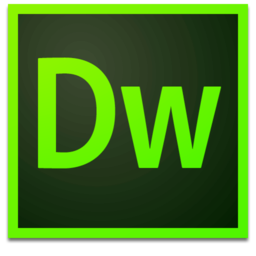
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
