How do modules and packages work in Python?
As a powerful programming language, Python has a rich standard library and also supports custom modules and packages, which makes program organization and reuse simpler and more efficient. This article will introduce the basic concepts of modules and packages in Python and illustrate how they work through specific code examples.
1. The concept and use of modules
In Python, a module is a file containing functions, variables and classes. Each Python file can be regarded as an independent module and introduced into other programs for use through the import statement. The following is a simple module example, saved as an example_module.py file:
# example_module.py PI = 3.14159 def circle_area(radius): return PI * radius * radius def square_area(side_length): return side_length ** 2
In another program, you can use the import statement to import this module and call the functions in it:
import example_module print(example_module.circle_area(2)) print(example_module.square_area(4))
Run The output results of the above code are 12.56636 and 16 respectively.
2. The Concept and Use of Packages
Package is a way to organize multiple modules. In Python, a package is a folder containing an __init__.py file. The __init__.py file can be an empty file, but its existence indicates that the folder is a package. The following is a simple package example, containing two modules circle.py and square.py, and an empty __init__.py file:
my_package/ __init__.py circle.py square.py
The contents of the circle.py file are as follows:
# circle.py PI = 3.14159 def area(radius): return PI * radius * radius## The content of the #square.py file is as follows:
# square.py def area(side_length): return side_length ** 2In another program, you can use the import statement to import the package and call the modules and functions in it:
import my_package.circle import my_package.square print(my_package.circle.area(2)) print(my_package.square.area(4))Run the above code and the output results are respectively is 12.56636 and 16. 3. How to import modules and packagesIn addition to the above import statements, Python also provides several other import methods to meet different needs.
- Import the specified function or variable from a module or package
from example_module import circle_area print(circle_area(2))
- Specify an alias for the imported module or function
import example_module as em print(em.circle_area(2))
- Import all modules in the package
from my_package import * print(circle.area(2)) print(square.area(4))
import sys print(sys.path)Running the above code will output a list containing multiple paths. 5. SummaryThis article introduces the basic concepts of modules and packages in Python, and demonstrates their usage through specific code examples. Modules and packages greatly improve the organization and reusability of programs, making programming simpler and more efficient. At the same time, mastering the import methods and search paths of modules and packages is also an important basis for writing Python programs. I hope that through the introduction of this article, readers can better understand and apply how modules and packages in Python work. Learning more about the use of modules and packages will make Python programming more flexible and powerful.
The above is the detailed content of How do modules and packages work in Python?. For more information, please follow other related articles on the PHP Chinese website!
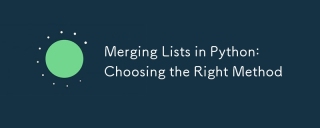
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
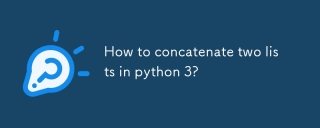
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
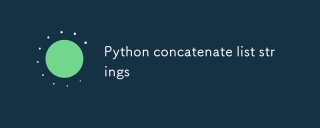
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
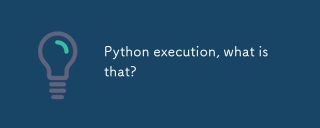
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
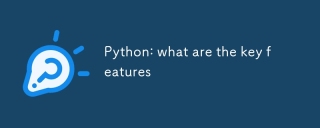
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
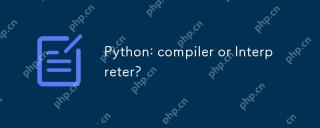
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
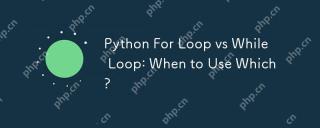
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
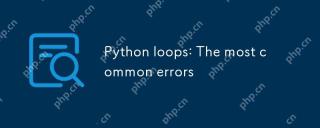
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
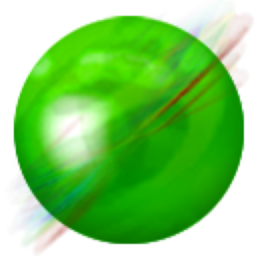
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
