


How to use indexes to improve the efficiency of paging queries in PHP and MySQL?
Introduction:
When using PHP and MySQL for paging queries, in order to improve query efficiency, database indexes can be used to speed up query operations. This article will explain how to create indexes correctly and how to perform paginated queries in PHP code.
1. What is an index
The index is a special data structure in the database, which can help the database system quickly locate the data records stored in the table. By creating indexes, query efficiency can be greatly improved.
2. How to create an index
In MySQL, you can add indexes by specifying columns as indexes when creating a table, or by using the ALTER TABLE statement after creating a table. Commonly used index types include ordinary indexes, unique indexes, primary key indexes and full-text indexes. When performing paging queries, normal indexes are usually used.
Sample code:
CREATE TABLE `user` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) NOT NULL, `age` int(11) NOT NULL, PRIMARY KEY (`id`), INDEX `idx_name` (`name`) ) ENGINE=InnoDB;
The above code creates a table named user and creates an ordinary index named idx_name on the name column.
3. How to use index for paging query
When performing paging query in PHP code, you can limit the number and sorting method of query results by using the LIMIT keyword and ORDER BY clause. Combined with the use of indexes, the efficiency of paging queries can be improved.
Sample code:
<?php $servername = "localhost"; $username = "your_username"; $password = "your_password"; $dbname = "your_database"; // 创建数据库连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 分页参数 $pageSize = 10; // 每页显示的记录数 $page = isset($_GET['page']) ? intval($_GET['page']) : 1; // 当前页码 // 查询总记录数 $sql = "SELECT COUNT(*) AS total FROM user"; $res = $conn->query($sql); $row = $res->fetch_assoc(); $total = $row['total']; // 查询分页数据 $offset = ($page - 1) * $pageSize; // 偏移量 $sql = "SELECT * FROM user ORDER BY name LIMIT $offset, $pageSize"; $res = $conn->query($sql); // 输出分页数据 if ($res->num_rows > 0) { while($row = $res->fetch_assoc()) { echo "ID: " . $row["id"]. " - Name: " . $row["name"]. " - Age: " . $row["age"]. "<br>"; } } else { echo "0 结果"; } // 输出分页导航 $totalPages = ceil($total / $pageSize); // 总页数 $prevPage = $page - 1; // 上一页页码 $nextPage = $page + 1; // 下一页页码 echo "<br>"; echo "<a href='?page=$prevPage'>上一页</a> "; for ($i = 1; $i <= $totalPages; $i++) { echo "<a href='?page=$i'>$i</a> "; } echo "<a href='?page=$nextPage'>下一页</a>"; // 关闭数据库连接 $conn->close(); ?>
The above code first creates a database connection and sets the paging parameters (the number of records displayed on each page and the current page number). Then use the query statement to obtain the total number of records, and query the paging data based on the paging parameters and LIMIT keyword. Finally, the paging data and paging navigation are output through a loop.
Summary:
By correctly creating indexes and performing paging queries in PHP code, the efficiency of paging queries in PHP and MySQL can be improved. In actual development, based on specific business needs and data volume, the operation of paging queries can be further optimized to provide better user experience and query performance.
The above is the detailed content of How to use indexes to improve the efficiency of paging queries in PHP and MySQL?. For more information, please follow other related articles on the PHP Chinese website!
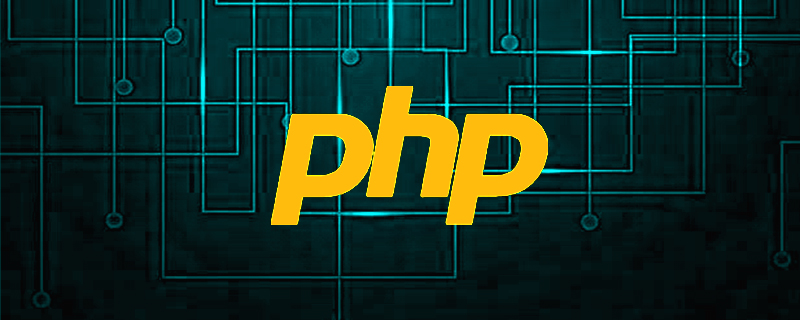
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
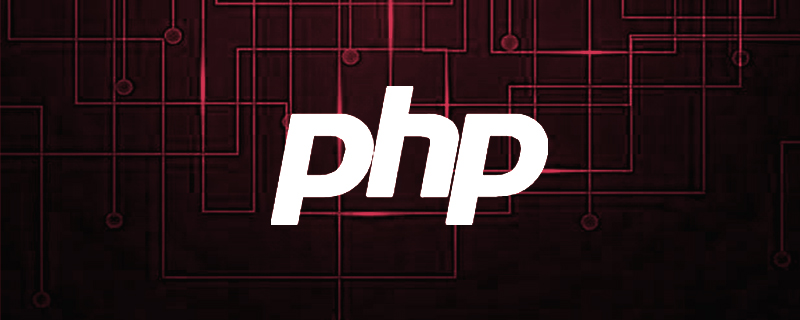
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
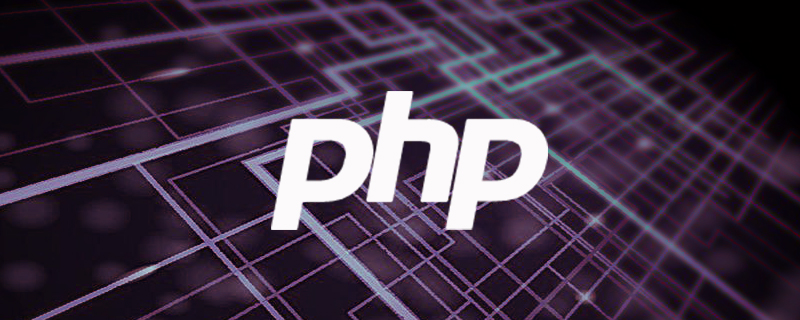
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
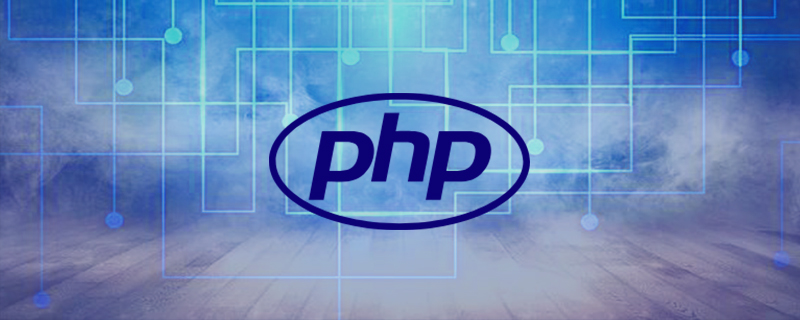
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
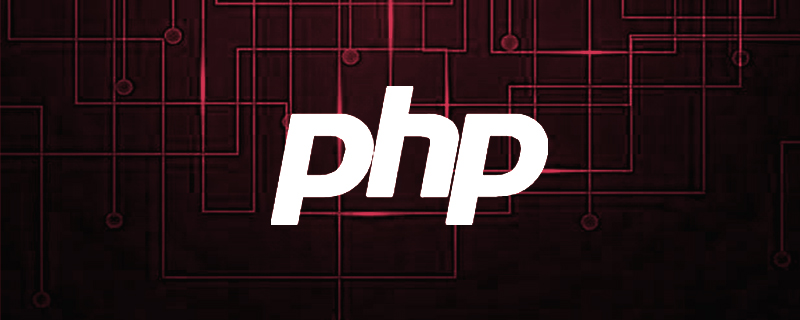
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
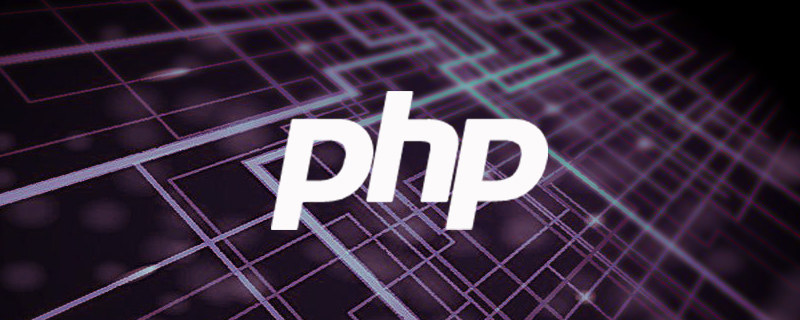
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
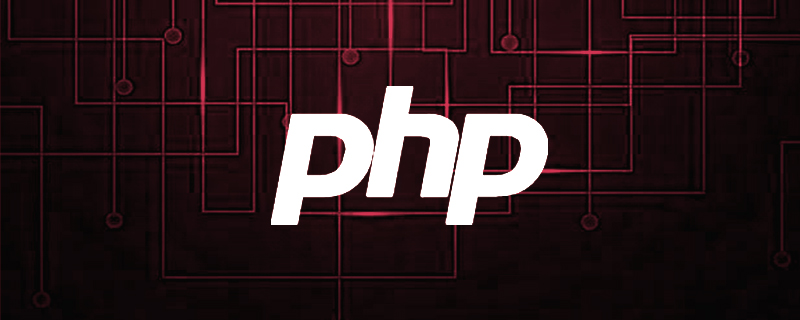
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
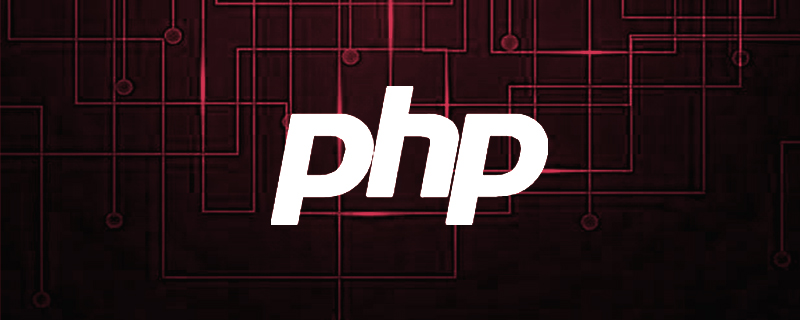
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
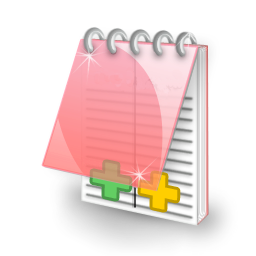
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
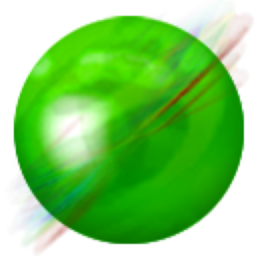
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
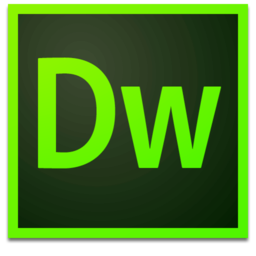
Dreamweaver Mac version
Visual web development tools
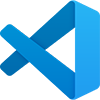
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
