How to handle asynchronous data requests and responses in Vue
How to handle asynchronous data requests and responses in Vue requires specific code examples
In front-end development, asynchronous data requests are often encountered, and Vue as A popular JavaScript framework that provides many convenient methods for handling asynchronous data requests and responses. This article will introduce some common techniques for handling asynchronous data in Vue and give specific code examples.
1. Using Vue’s life cycle hook function
Vue’s life cycle hook function is a set of functions that are called at different stages of the Vue instance. We can use the created and mounted hook functions to handle asynchronous data requests and corresponding logic.
- Initiate an asynchronous data request in the created hook function:
export default { data() { return { users: [] }; }, created() { this.fetchUsers(); }, methods: { fetchUsers() { axios.get('/api/users') .then(response => { this.users = response.data; }) .catch(error => { console.log(error); }); } } }
In the above example, we used the axios library to send a GET request to obtain user data. When the request is successful, the returned data is saved to the users attribute in the data of the Vue instance.
- Operation on asynchronous data in the mounted hook function:
export default { data() { return { users: [] }; }, mounted() { this.fetchUsers(); }, methods: { fetchUsers() { axios.get('/api/users') .then(response => { this.users = response.data; this.$nextTick(() => { // 对DOM进行操作 }); }) .catch(error => { console.log(error); }); } } }
In the above example, we also used axios to send a GET request to obtain user data. When the request is successful, the returned data is saved to the users attribute in the data of the Vue instance, and some operations are performed after the DOM update is completed.
2. Using Vue’s asynchronous components
Vue’s asynchronous components provide a way to delay loading components, which can effectively increase the speed of page initialization. We can pass the asynchronous request data as props of the asynchronous component to the sub-component for rendering.
The following is an example:
// 异步组件定义 const UserList = () => import('./UserList.vue'); export default { data() { return { users: [] }; }, created() { this.fetchUsers(); }, components: { UserList }, methods: { fetchUsers() { axios.get('/api/users') .then(response => { this.users = response.data; }) .catch(error => { console.log(error); }); } } } // UserList.vue <template> <div> <ul> <li v-for="user in users" :key="user.id">{{ user.name }}</li> </ul> </div> </template> <script> export default { props: ['users'] // 接收父组件传递过来的数据 } </script>
In the above example, we load the UserList component through the import statement and register it in the components attribute in the Vue instance. Then, the user data is requested asynchronously in the parent component and the data is passed as props to the child component UserList for rendering.
3. Utilize Vue’s responsive data
Vue’s data responsive mechanism can handle asynchronous data changes well. We can directly use the data attribute of the Vue instance to save the data returned by the asynchronous request, and use the watch attribute to monitor data changes.
The sample code is as follows:
export default { data() { return { users: [] }; }, created() { this.fetchUsers(); }, watch: { users(newVal) { // 对异步数据的变化进行处理 } }, methods: { fetchUsers() { axios.get('/api/users') .then(response => { this.users = response.data; }) .catch(error => { console.log(error); }); } } }
In the above example, we use the watch attribute to monitor changes in users data and perform some operations when the data changes.
Summary:
This article introduces common techniques for handling asynchronous data requests and responses in Vue, and gives specific code examples. By taking advantage of Vue's life cycle hook functions, asynchronous components and reactive data, we can process asynchronous data more easily and improve the efficiency of front-end development. I hope this article can be helpful to Vue developers when dealing with asynchronous data.
The above is the detailed content of How to handle asynchronous data requests and responses in Vue. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
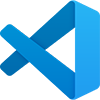
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
