How to use Vuex to implement state management in Vue projects
How to use Vuex to implement state management in Vue projects
Introduction:
In Vue.js development, state management is an important topic. As the complexity of an application increases, passing and sharing data between components becomes complex and difficult. Vuex is the official state management library of Vue.js, providing developers with a centralized state management solution. In this article, we will discuss the use of Vuex, along with specific code examples.
- Installing and configuring Vuex:
First, we need to install Vuex. In your Vue project, use npm or yarn to install Vuex:
npm install vuex
Then, create a file named store.js in your Vue project to configure Vuex. In this file, introduce Vue and Vuex, and create a new store instance:
import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) const store = new Vuex.Store({ // 在这里配置你的状态和相关的mutations,getters,actions等 }) export default store
- Define state and changes:
In Vuex, the state is called "store", which can be passedstate
attribute to declare. For example, we can add a state namedcount
to the store.js file:
const store = new Vuex.Store({ state: { count: 0 } })
We also need to define functions that will be triggered when the state changes. These functions are Called "mutations". In mutations, we can modify the state. For example, we can add a mutation named increment
to increase the value of count:
const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count++ } } })
- Using state in the component:
Once we have configured the Vuex state and changes, we can use them in the component. In the component, you can access the Vuex store throughthis.$store
. For example, to use the count state in a component'stemplate
:
<template> <div> <p>Count: {{ this.$store.state.count }}</p> <button @click="increment">Increment</button> </div> </template> <script> export default { methods: { increment () { this.$store.commit('increment') } } } </script>
In the above code, we pass this.$store.state.count
To get the value of count status, and trigger the mutation of increment through this.$store.commit('increment')
.
- Computed properties and getters:
Sometimes, we need to derive some new calculated properties from the state, such as filtering or calculating the state. In Vuex, this can be achieved usinggetters
. In store.js, we can add a getter namedevenOrOdd
to determine whether the value of count is odd or even:
const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count++ } }, getters: { evenOrOdd: state => state.count % 2 === 0 ? 'even' : 'odd' } })
Then use the getter in the component, you can pass this.$store.getters
to access. For example, use evenOrOdd calculated property in component's template
:
<template> <div> <p>Count: {{ this.$store.state.count }}</p> <p>Count is {{ this.$store.getters.evenOrOdd }}</p> <button @click="increment">Increment</button> </div> </template> <script> export default { methods: { increment () { this.$store.commit('increment') } } } </script>
- Asynchronous operations and actions:
Sometimes, we need to perform some asynchronous operations in mutation, such as Send a request or delay updating status. In Vuex, this can be achieved usingactions
. In store.js, we can add an action namedincrementAsync
to implement the operation of asynchronously increasing the count:
const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count++ } }, actions: { incrementAsync ({ commit }) { setTimeout(() => { commit('increment') }, 1000) } } })
Then trigger the action in the component, you can pass this.$store.dispatch
to access. For example, trigger the incrementAsync action in the component's methods
:
export default { methods: { increment () { this.$store.dispatch('incrementAsync') } } }
Summary:
In this article, we discussed how to use Vuex to implement state management in Vue projects. We demonstrate the use of Vuex through examples of installing and configuring Vuex, defining states and changes, using states and changes, and using computed properties and actions. I hope this article has provided some help for you to use Vuex in your Vue project.
The above is the detailed content of How to use Vuex to implement state management in Vue projects. For more information, please follow other related articles on the PHP Chinese website!

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.

Vue.js and React each have their own advantages, and the choice should be based on project requirements and team technology stack. 1. Vue.js is community-friendly, providing rich learning resources, and the ecosystem includes official tools such as VueRouter, which are supported by the official team and the community. 2. The React community is biased towards enterprise applications, with a strong ecosystem, and supports provided by Facebook and its community, and has frequent updates.

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
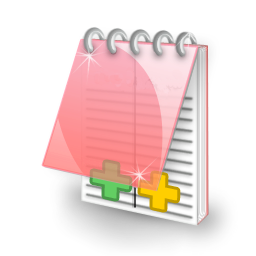
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
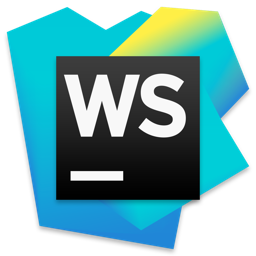
WebStorm Mac version
Useful JavaScript development tools
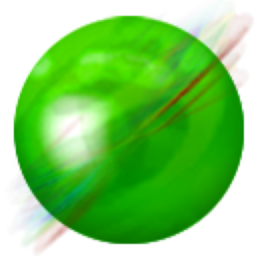
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
