


Queue message preprocessing and message retry strategy in PHP and MySQL
Message preprocessing and message retry strategy of queues in PHP and MySQL
Introduction:
In modern network applications, message queue is an important The concurrent processing mechanism is widely used. Queues can process time-consuming tasks asynchronously, thereby improving the concurrency performance and stability of applications. This article will introduce how to use PHP and MySQL to implement queue message preprocessing and message retry strategy, and provide specific code examples.
1. The concept and function of message queue
Message queue is a common asynchronous communication mechanism. It involves the message producer putting tasks into the queue, and the message consumer getting the tasks from the queue and processing them. This method can avoid direct blocking or timeout of tasks under high concurrency conditions, and improve the response speed and availability of the application. Common message queue systems include RabbitMQ, Kafka, ActiveMQ, etc.
2. Methods of implementing queues with PHP and MySQL
Although Redis is the preferred database for queue implementation, in some cases, it may be necessary to use MySQL as the storage medium for the message queue. The following will introduce the methods of implementing queues in PHP and MySQL, and provide specific code examples.
-
Create MySQL data table
First, we need to create a MySQL data table to store messages in the queue. The structure of the table can be defined as the following three fields:CREATE TABLE message_queue ( id INT(11) AUTO_INCREMENT PRIMARY KEY, message TEXT NOT NULL, status INT(11) DEFAULT 0 );
Here, the
message
field is used to store the specific content of the task, and thestatus
field is used to identify the execution of the task state. -
Producer code example
The producer is responsible for adding tasks to the queue. Here we use PHP's mysqli extension to implement MySQL connection and data insertion operations.<?php $mysqli = new mysqli("localhost", "username", "password", "database"); if ($mysqli->connect_errno) { die("Failed to connect to MySQL: " . $mysqli->connect_error); } $message = "Task message"; $query = "INSERT INTO message_queue (message) VALUES ('$message')"; $result = $mysqli->query($query); if ($result) { echo "Message added to the queue"; } else { echo "Failed to add message to the queue"; } $mysqli->close(); ?>
In the above example, we insert tasks into the
message_queue
table through theINSERT
statement. -
Consumer Code Example
Consumers are responsible for getting tasks from the queue and processing them. The following example uses PHP's mysqli extension to implement MySQL connection and query operations.<?php $mysqli = new mysqli("localhost", "username", "password", "database"); if ($mysqli->connect_errno) { die("Failed to connect to MySQL: " . $mysqli->connect_error); } $query = "SELECT * FROM message_queue WHERE status = 0 LIMIT 1"; $result = $mysqli->query($query); if ($result->num_rows > 0) { $row = $result->fetch_assoc(); $message = $row['message']; // 处理任务的逻辑 // ... // 标记任务为已执行 $id = $row['id']; $updateQuery = "UPDATE message_queue SET status = 1 WHERE id = $id"; $mysqli->query($updateQuery); echo "Task processed successfully"; } else { echo "No pending tasks in the queue"; } $result->free(); $mysqli->close(); ?>
In the above example, we first obtain the unexecuted tasks from the
message_queue
table through theSELECT
statement, then perform the task processing operation, and finally pass the ## The #UPDATEstatement marks the task as executed.
Queue message preprocessing is to prepare and handle some common error situations in advance to prevent problems in task execution. Specific preprocessing strategies vary from application to application. Here are some common examples of message preprocessing strategies:
- Message duplicate detection: Before adding a task to the queue, check whether the message already exists in the queue. You can avoid the insertion of duplicate messages by adding a unique index to the table.
- Task timeout processing: Before the consumer processes the task, determine whether the task exceeds the preset time limit. When a task times out, you can choose to mark the task as failed and log it, or add the task back to the queue for subsequent processing.
- Message loss prevention measures: Before the consumer processes the task, the task can be marked as "locked" to indicate that the task is being processed. If a consumer stops processing tasks when a timeout or error occurs, the polling and timeout mechanisms can be used to reacquire unfinished tasks and add them back to the queue.
Message retry means that when task execution fails, the task is re-added to the queue for retry execution. The following are some common examples of message retry strategies:
- Retry limit: You can set the maximum retry count for a task. When the task reaches the maximum retry count and still fails, the task can be marked as Fails and logs.
- Retry delay setting: You can set the retry delay time of the task. When the task fails, wait for a period of time and then re-add the task to the queue. The retry delay time can be set according to business needs.
- Retry times exponential backoff: After each retry failure, the number of retries can be increased exponentially to avoid frequent retry failures. For example, the first retry interval is 1 second, the second retry interval is 2 seconds, the third retry interval is 4 seconds, and so on.
By using the message preprocessing and message retry strategy of the queue, the concurrency performance and stability of the application can be improved. This article introduces how to use PHP and MySQL to implement queue message preprocessing and message retry strategies, and provides specific code examples. Hope this article is helpful to you.
The above is the detailed content of Queue message preprocessing and message retry strategy in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!
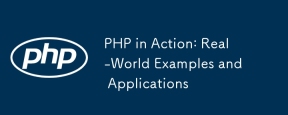
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
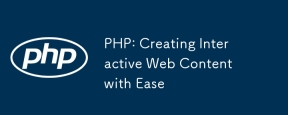
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
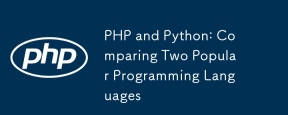
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
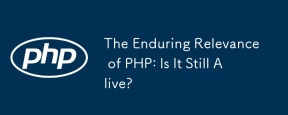
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
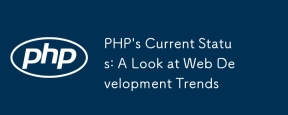
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
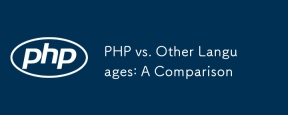
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
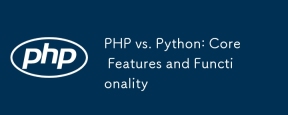
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
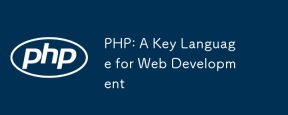
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
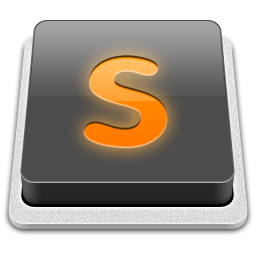
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
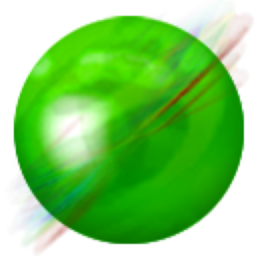
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment