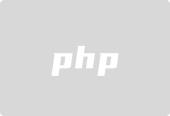
In-depth understanding of the application of Vuex in the Vue project requires specific code examples
Introduction:
In the Vue project, state management is a very important task . As the complexity of projects continues to increase, the communication between components and the management of status become more and more complex. To solve these problems, Vue introduces Vuex, a state management pattern developed specifically for Vue.js applications. This article will provide an in-depth understanding of the application of Vuex in Vue projects and demonstrate its usage through specific code examples.
- What is Vuex
Vuex is a state management model developed specifically for Vue.js applications and is used to manage the data state in the application. Vuex uses centralized storage to manage the state of all components of the application, and uses corresponding rules to ensure that the state can only be modified in a predictable way. It integrates the state management model used in Vue components and provides a centralized state management solution.
- The core concepts of Vuex
The core concepts of Vuex include: state, mutations, actions, getters and modules.
- state: The state in Vuex is used to store the data state in the application. It is unique, meaning that the entire application's state should be stored in one object. Data in state can be easily obtained and modified through the API provided by Vuex.
- mutations: Mutations are a special function in Vuex, used to modify data in state. Trigger mutations through the commit method in the Vue component. Mutations must be synchronous functions to ensure that state changes are trackable.
- actions: Actions are similar to mutations, which are used to handle asynchronous operations. Actions trigger mutations through the commit method. Actions can include asynchronous operations, such as requesting data, changing data in the state, etc., and trigger mutations through the commit method to modify the state.
- Getters: Getters are used to calculate or filter the data in state. Similar to computed properties in Vue components, derived data can be obtained through getters.
- modules: When the application becomes complex, Vuex can be modularized. Each module has its own state, mutations, actions, getters, and can be nested among each other.
- Using Vuex in the Vue project
First, we need to install Vuex in the Vue project. You can use npm or yarn to install:
npm install vuex
Then, introduce and use Vuex in the entry file main.js of the Vue project:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use( Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
},
actions: {
increment(context) {
context.commit('increment')
}
},
getters: {
doubleCount(state) {
return state.count * 2
}
}
})
new Vue({
store,
render : h => h(App)
}).$mount('#app')
- Using Vuex in Vue components
Using Vuex in Vue components is very simple, You only need to introduce Vuex into the component and use the auxiliary functions provided by Vuex to access and modify state, trigger mutations and actions.
- Access state:
this.$store.state.count
- Modify state:
this.$store.commit('increment')
- Trigger actions:
this.$store.dispatch('increment')
- Access getters:
this.$store.getters.doubleCount
- Modular Vuex
When the application becomes complex, Vuex can be modularized. Each module has its own state, mutations, actions, and getters. Modular Vuex makes state management clearer and more flexible.
For example, you can create a todo module to handle state management related to to-do items:
const todo = {
namespaced: true,
state: {
todos: []
},
mutations: {
addTodo(state, todo) {
state.todos.push(todo)
}
},
actions: {
addTodo(context, todo) {
context.commit('addTodo', todo)
}
},
getters: {
completedTodos(state) {
return state.todos.filter(todo => todo.completed)
},
uncompletedTodos(state) {
return state.todos.filter(todo => !todo.completed)
}
}
}
const store = new Vuex.Store({
modules: {
todo
}
})
Then use it in the component Modular Vuex:
- Access state:
this.$store.state.todo.todos
- Modify state:
this.$store.commit(' todo/addTodo', todo)
- Trigger actions:
this.$store.dispatch('todo/addTodo', todo)
- Access getters:
this.$store .getters['todo/completedTodos']
Summary:
Vuex is the state management mode of Vue.js application. It manages the data state of the application through centralized storage, making the communication between components Communication and status management are easier. By deeply understanding the core concepts of Vuex and specific code examples, we can better use and master the application of Vuex in Vue projects. When developing complex Vue projects, proper use of Vuex can improve development efficiency and enhance the maintainability and testability of the project.
The above is the detailed content of In-depth understanding of the application of Vuex in Vue projects. For more information, please follow other related articles on the PHP Chinese website!