Vue.js适合小型到中型项目,React适合大型项目和复杂应用场景。1) Vue.js易于上手,适用于快速原型开发和小型应用。2) React在处理复杂状态管理和性能优化方面更有优势,适合大型项目。
引言
在现代前端开发的世界里,Vue.js和React无疑是两颗耀眼的明星。无论你是刚入门的前端开发者,还是经验丰富的大牛,选择一个合适的框架往往是项目成功的关键。今天,我们就来深入探讨Vue.js和React各自的用例和应用场景,希望能给你一些启发和指引。通过这篇文章,你将了解到这两大框架的核心特性,以及它们在不同情境下的最佳实践。
在我的开发生涯中,我曾多次在项目中使用过Vue.js和React,每次都感受到它们独特的魅力和挑战。Vue.js以其灵活性和易用性著称,而React则以其强大和社区支持赢得了无数开发者的青睐。让我们从基础知识开始,逐步深入探讨这两者的异同。
基础知识回顾
Vue.js是一个渐进式的JavaScript框架,它的设计理念是可以自底向上逐层应用。它的核心库只关注视图层,非常容易上手,同时也非常容易与其他库或现有项目整合。Vue.js的双向数据绑定和组件化开发模式让开发者能够快速构建用户界面。
React则是由Facebook开发的一个用于构建用户界面的JavaScript库。它采用虚拟DOM和单向数据流的设计理念,强调组件的可复用性和性能优化。React的生态系统非常丰富,有众多第三方库和工具支持,如Redux用于状态管理,React Router用于路由管理等。
核心概念或功能解析
Vue.js的核心概念
Vue.js的核心概念是其响应式数据系统和组件系统。通过使用Vue.js,你可以轻松地将数据和DOM进行绑定,任何数据的变化都会自动反映到视图上。以下是一个简单的Vue.js组件示例:
<template> <div> <h1 id="title">{{ title }}</h1> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { title: 'Hello Vue!', message: 'Welcome to Vue.js' } } } </script>
这个组件展示了Vue.js的基本用法,通过data
属性定义数据,然后在模板中使用插值表达式{{ }}
来显示数据。Vue.js会自动监测数据的变化,并更新视图。
React的核心概念
React的核心概念是组件和虚拟DOM。React通过将UI拆分为独立的、可复用的组件来管理复杂的用户界面。以下是一个简单的React组件示例:
import React from 'react'; const App = () => { const title = 'Hello React!'; const message = 'Welcome to React'; return ( <div> <h1 id="title">{title}</h1> <p>{message}</p> </div> ); }; export default App;
React使用JSX语法来描述UI结构,组件通过return
语句返回JSX元素。React的虚拟DOM机制会高效地更新真实DOM,确保性能最优。
工作原理
Vue.js的响应式系统通过Object.defineProperty
或Proxy
来实现数据的监听。当数据发生变化时,Vue.js会触发相应的更新操作,确保视图与数据保持同步。Vue.js的组件系统则通过模板编译和虚拟DOM来渲染组件,确保高效的DOM操作。
React的工作原理则是通过虚拟DOM和Diff算法来实现高效的DOM更新。React会将组件的渲染结果与上一次的结果进行比较,找出差异部分,然后只更新需要更新的DOM节点。这种方式大大减少了DOM操作,提高了性能。
使用示例
Vue.js的基本用法
Vue.js的基本用法非常简单,只需创建一个Vue实例并挂载到DOM上即可。以下是一个简单的示例:
new Vue({ el: '#app', data: { message: 'Hello Vue!' } })
这个示例创建了一个Vue实例,并将其挂载到id为app
的元素上,data
属性定义了组件的数据。
React的基本用法
React的基本用法是创建一个React组件,并通过ReactDOM.render将组件渲染到DOM上。以下是一个简单的示例:
import React from 'react'; import ReactDOM from 'react-dom'; const App = () => { return <h1 id="Hello-React">Hello React!</h1>; }; ReactDOM.render(<App />, document.getElementById('root'));
这个示例创建了一个简单的React组件,并将其渲染到id为root
的元素上。
高级用法
Vue.js的高级用法
Vue.js的高级用法包括使用计算属性、自定义指令、混入等功能。以下是一个使用计算属性的示例:
<template> <div> <p>Full Name: {{ fullName }}</p> </div> </template> <script> export default { data() { return { firstName: 'John', lastName: 'Doe' } }, computed: { fullName() { return this.firstName + ' ' + this.lastName; } } } </script>
这个示例展示了如何使用计算属性fullName
来计算和显示完整的名字。
React的高级用法
React的高级用法包括使用Hooks、上下文API、自定义Hooks等功能。以下是一个使用Hooks的示例:
import React, { useState, useEffect } from 'react'; const Counter = () => { const [count, setCount] = useState(0); useEffect(() => { document.title = `Count: ${count}`; }, [count]); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter;
这个示例展示了如何使用useState
和useEffect
来管理状态和副作用。
常见错误与调试技巧
Vue.js的常见错误
Vue.js的常见错误包括数据未正确绑定、组件未正确注册等。以下是一些调试技巧:
- 使用Vue Devtools来查看组件树和数据状态。
- 检查模板中的语法错误,确保插值表达式和指令使用正确。
- 确保组件的
data
属性是一个函数,返回一个新的对象实例。
React的常见错误
React的常见错误包括状态管理不当、组件未正确渲染等。以下是一些调试技巧:
- 使用React Devtools来查看组件树和状态。
- 检查JSX语法,确保标签正确闭合和属性正确使用。
- 确保使用
key
属性来优化列表渲染,避免不必要的重新渲染。
性能优化与最佳实践
Vue.js的性能优化
Vue.js的性能优化可以从以下几个方面入手:
- 使用
v-if
和v-show
来控制元素的显示和隐藏,v-if
适用于条件不频繁变化的情况,v-show
适用于条件频繁变化的情况。 - 使用
keep-alive
来缓存组件,避免不必要的重新渲染。 - 使用
async
和await
来处理异步操作,避免阻塞主线程。
以下是一个使用keep-alive
的示例:
<template> <div> <keep-alive> <component :is="currentComponent"></component> </keep-alive> </div> </template> <script> export default { data() { return { currentComponent: 'ComponentA' } } } </script>
这个示例展示了如何使用keep-alive
来缓存组件,避免不必要的重新渲染。
React的性能优化
React的性能优化可以从以下几个方面入手:
- 使用
React.memo
来优化函数组件的重新渲染。 - 使用
useMemo
和useCallback
来优化计算和回调函数的性能。 - 使用
shouldComponentUpdate
或PureComponent
来控制组件的重新渲染。
以下是一个使用React.memo
的示例:
import React from 'react'; const MyComponent = React.memo((props) => { return <div>{props.value}</div>; }); export default MyComponent;
这个示例展示了如何使用React.memo
来优化函数组件的重新渲染,避免不必要的性能开销。
最佳实践
无论是Vue.js还是React,编写高质量代码的最佳实践包括:
- 保持代码的可读性和可维护性,使用有意义的变量名和函数名。
- 遵循组件的单一职责原则,确保每个组件只做一件事。
- 使用模块化和组件化的开发模式,提高代码的复用性和可测试性。
深度见解与建议
在选择Vue.js还是React时,需要考虑项目的具体需求和团队的技术栈。Vue.js适合小型到中型项目,易于上手和快速开发。React则适合大型项目和复杂的应用场景,提供了更强大的性能优化和生态系统支持。
在实际项目中,我发现Vue.js在快速原型开发和小型应用中表现出色,而React在处理复杂的状态管理和性能优化方面更有优势。无论选择哪一个,都需要深入了解其核心概念和最佳实践,才能发挥其最大潜力。
关于踩坑点,Vue.js的双向数据绑定有时会导致难以追踪的数据流,而React的单向数据流则需要更多的代码来管理状态。Vue.js的模板语法虽然易于理解,但有时会限制灵活性,而React的JSX语法则提供了更大的灵活性,但也需要更多的学习成本。
总之,Vue.js和React各有千秋,选择哪一个取决于你的项目需求和团队的技术栈。希望这篇文章能为你提供一些有价值的见解和指引,帮助你做出明智的选择。
The above is the detailed content of Vue.js vs. React: Use Cases and Applications. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.

Vue.js and React each have their own advantages, and the choice should be based on project requirements and team technology stack. 1. Vue.js is community-friendly, providing rich learning resources, and the ecosystem includes official tools such as VueRouter, which are supported by the official team and the community. 2. The React community is biased towards enterprise applications, with a strong ecosystem, and supports provided by Facebook and its community, and has frequent updates.

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
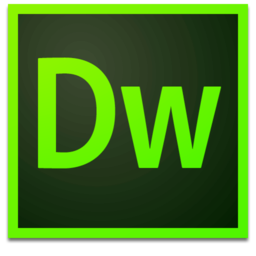
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
