How to use third-party libraries to extend and optimize projects in Vue
Vue.js is a popular front-end development framework with concise syntax and flexible architecture. Although it already provides rich functionality, during the development process, we often need to use third-party libraries to extend and optimize our projects. This article will introduce how to use third-party libraries in Vue and provide specific code examples.
1. Introduction of third-party libraries
To use third-party libraries in Vue, you first need to introduce them into the project. There are many ways to achieve this, two common methods are introduced below.
1. Use npm to install
Most third-party libraries can be installed through npm and then introduced using the import statement. For example, if we want to use a third-party library called Axios to send HTTP requests, we can first run the following command in the terminal to install it:
npm install axios
After the installation is complete, use the import statement in a file of the project to introduce it :
import axios from 'axios';
2. Import using CDN
If the third-party library provides a CDN (content distribution network) link, we can also introduce it directly in the HTML file. For example, if we want to use the Font Awesome icon library in our project, we can add the following code to the head tag of the HTML file:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css">
2. Use a third-party library
After introducing the third-party library, we can Use them in Vue. Two typical application scenarios will be introduced below and specific code examples will be provided.
1. Use UI component library
In actual development, UI component library is often used to quickly build the interface. These component libraries provide rich styles and components, which can greatly improve development efficiency. For example, if we want to use a component library called Element UI, we can first introduce it as above, and then use them in Vue components:
// 引入 Element UI import ElementUI from 'element-ui'; import 'element-ui/lib/theme-chalk/index.css'; // 注册组件 Vue.use(ElementUI); // 使用组件 <template> <el-button>Click me</el-button> </template>
2. Use the tool library
In addition to the UI component library, we You can also use some tool libraries to easily handle some common needs. For example, if we want to use a tool library called Lodash to handle data operations, we can first introduce it as mentioned above, and then use them in Vue components:
// 引入 Lodash import _ from 'lodash'; // 使用 export default { data() { return { numbers: [1, 2, 3, 4, 5], }; }, computed: { sum() { return _.sum(this.numbers); }, max() { return _.max(this.numbers); }, min() { return _.min(this.numbers); }, }, };
3. Expansion and optimization projects
Use Chapter 1 Third-party libraries can not only expand the functions of the project, but also be used to optimize the performance and development efficiency of the project. Two common application scenarios are introduced below.
1. Network request
In projects with separate front-end and back-end, we usually need to send network requests to obtain data. Vue officially recommends using Axios to send network requests. The following is a specific code example:
import axios from 'axios'; export default { mounted() { axios.get('/api/user') .then(response => { console.log(response.data); }) .catch(error => { console.error(error); }); }, };
2. State management
When the state of the application becomes complex, you can use a third-party library to manage the global state. Vuex is the state management library officially recommended by Vue. It can easily manage all the states of the application. The following is a specific code example:
import Vuex from 'vuex'; Vue.use(Vuex); const store = new Vuex.Store({ state: { count: 0, }, mutations: { increment(state) { state.count++; }, decrement(state) { state.count--; }, }, }); export default store;
The above are just two simple examples. In actual applications, appropriate third-party libraries can be selected according to project requirements to expand and optimize the project.
Summary:
This article introduces how to use third-party libraries in Vue and provides specific code examples. Using third-party libraries can expand the functionality of the project and optimize the performance and development efficiency of the project. In actual development, we can choose appropriate third-party libraries to apply in Vue projects based on project needs, giving full play to the advantages of Vue.
The above is the detailed content of How to use third-party libraries to extend and optimize projects in Vue. For more information, please follow other related articles on the PHP Chinese website!

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
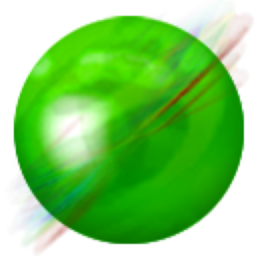
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
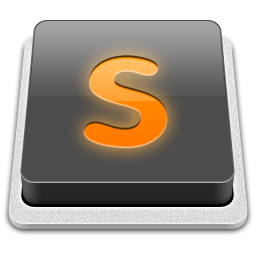
SublimeText3 Mac version
God-level code editing software (SublimeText3)
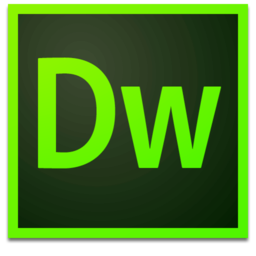
Dreamweaver Mac version
Visual web development tools
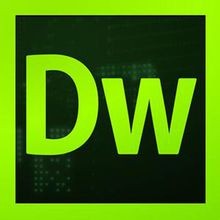
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
