Netflix's Frontend: A Deep Dive into Its Technology Stack
Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.
introduction
Netflix's user experience has always been a benchmark in the industry. Its front-end technology stack not only supports the smooth viewing experience of hundreds of millions of users, but also provides other companies with templates for learning. Today we will explore Netflix's front-end technology stack in depth and uncover the mysterious and powerful technical forces behind it. Read this article and you will learn how Netflix uses modern front-end technologies to build its platform, from architectural design to specific technical implementations, we will unveil it one by one.
Review of basic knowledge
Netflix's front-end technology stack is a complex and sophisticated system. To understand its overall picture, we need to first review some basic concepts. Netflix's front-end is mainly based on React, a JavaScript library for building user interfaces, widely used to build high-performance single-page applications (SPAs). In addition, Netflix also uses Redux to manage application state, making the data flow between components clearer and more controllable.
Netflix's front-end development is inseparable from modern toolchains, such as Webpack for module packaging, Babel for JavaScript translation, and Jest and Enzyme for unit testing. Together, these tools form the front-end development environment of Netflix to ensure the quality and performance of the code.
Core concept or function analysis
React and component development
React is the core of Netflix's front-end technology stack. It greatly improves the reusability and maintenance of code through component development. In Netflix applications, components are not only part of the UI, but also the carrier of business logic. Through React's virtual DOM, Netflix can efficiently perform DOM operations to ensure the smoothness of the user interface.
// A simple React component example import React from 'react'; <p>const MovieCard = ({ title, year }) => ( </p><div classname="movie-card"> <h2 id="title">{title}</h2> <p>Year: {year}</p> </div> );<p> export default MovieCard;</p>
React's componentized development not only improves development efficiency, but also allows Netflix to iterate and update its UI quickly, which is particularly important in the rapidly changing streaming market.
State Management and Redux
Netflix uses Redux to manage application state, which makes changes in states predictable and traceable. With Redux, Netflix maintains consistency and maintainability in complex applications.
// Redux state management example import { createStore } from 'redux'; <p>const initialState = { movies: [] };</p><p> function moviesReducer(state = initialState, action) { switch (action.type) { case 'ADD_MOVIE': return { ...state, movies: [...state.movies, action.payload] }; default: return state; } }</p><p> const store = createStore(moviesReducer);</p><p> store.dispatch({ type: 'ADD_MOVIE', payload: { title: 'Inception', year: 2010 } });</p>
The advantage of using Redux is that it provides a single trusted data source, making state management simpler and more intuitive, but it also means more boilerplate code and learning curves are required.
Example of usage
Basic usage
In the front-end development of Netflix, the basic usage of React components is the basis for building a user interface. With simple and intuitive syntax, developers can quickly create and combine components.
// Basic React component usage import React from 'react'; <p>const App = () => ( </p><div> <h1 id="Welcome-to-Netflix">Welcome to Netflix</h1> <moviecard title="The Matrix" year="1999"></moviecard> </div> );<p> export default App;</p>
This basic usage allows developers to quickly build page structures and implement complex UIs through combinations of components.
Advanced Usage
In front-end development of Netflix, advanced usage includes using Hooks to manage state and side effects, and using the Context API to pass data. Hooks enable function components to have the functions of class components, while the Context API simplifies data transfer between components.
// Advanced usage of using Hooks import React, { useState, useEffect } from 'react'; <p>const MovieList = () => { const [movies, setMovies] = useState([]);</p><p> useEffect(() => { fetchMovies().then(setMovies); }, []);</p><p> Return ( </p>
-
{movies.map(movie => (
- {movie.title} ))}
export default MovieList;
This advanced usage makes Netflix's front-end development more flexible and efficient, but also requires developers' in-depth understanding of React.
Common Errors and Debugging Tips
Common errors in Netflix's front-end development include incorrect components updates, improper state management, and performance issues. When debugging these issues, you can use React DevTools to view component tree and state changes, and Chrome DevTools to analyze performance bottlenecks.
For example, if the component is not updated correctly, it may be because the state change is not detected correctly. At this time, you can use React's useEffect Hook to listen for the state change and perform corresponding processing.
Performance optimization and best practices
In Netflix's front-end development, performance optimization is a key link. Netflix uses a variety of technologies to improve application performance, including code segmentation, lazy loading, and server-side rendering (SSR).
Code segmentation allows Netflix to load components on demand, reducing initial loading time, while lazy loading further optimizes the user experience. Server-side rendering greatly improves the loading speed of Netflix's first screen, enhancing the user's first impression.
// Code segmentation example import React, { lazy, Suspense } from 'react'; <p>const MovieDetails = lazy(() => import('./MovieDetails'));</p><p> const App = () => ( <suspense fallback="{<div">Loading...}> <moviedetails></moviedetails> </suspense> );</p><p> export default App;</p>
In terms of best practices, Netflix emphasizes the readability and maintainability of the code. By using ESLint and Prettier, Netflix ensures consistency and quality of code. In addition, Netflix also advocates the use of TypeScript to enhance the type safety of code, which is particularly important in complex front-end projects.
In general, Netflix's front-end technology stack is a highly optimized and carefully designed system. Through React, Redux and a series of modern tools, Netflix not only provides users with a smooth viewing experience, but also provides developers with a platform for learning and growth.
The above is the detailed content of Netflix's Frontend: A Deep Dive into Its Technology Stack. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
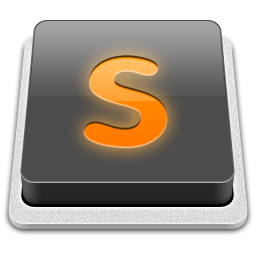
SublimeText3 Mac version
God-level code editing software (SublimeText3)
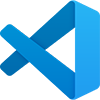
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
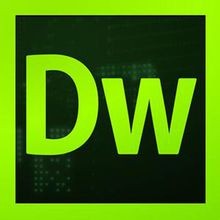
Dreamweaver CS6
Visual web development tools
