


Application of queue technology in message persistence and lazy loading in PHP and MySQL
Application of Queue Technology in Message Persistence and Lazy Loading in PHP and MySQL
Introduction
Queue technology is widely used in various computers A data structure in the system that enables asynchronous processing of messages and optimizes system performance. In the development of PHP and MySQL, queue technology also plays an important role. This article will introduce how to use queue technology to achieve message persistence and lazy loading, and provide corresponding PHP and MySQL code examples.
Message persistence
Message persistence refers to saving messages to persistent storage media to ensure that messages will not be lost even after a system failure or restart. In PHP and MySQL development, we can use the MySQL database to achieve persistent storage of messages.
First, we create a data table named "messages" to save the content and status of messages.
CREATE TABLE messages ( id INT AUTO_INCREMENT PRIMARY KEY, content TEXT NOT NULL, status ENUM('pending', 'processed') NOT NULL DEFAULT 'pending' );
We can then save the message to the database using the following PHP code:
<?php // 连接到数据库 $connection = new mysqli('localhost', 'username', 'password', 'database'); // 插入消息到数据库 $content = '这是一条示例消息'; $statement = $connection->prepare('INSERT INTO messages (content) VALUES (?)'); $statement->bind_param('s', $content); $statement->execute(); // 关闭数据库连接 $connection->close(); ?>
By saving the message to the database, even if the system encounters a failure or restarts, we can still retrieve the message from the database Read saved messages and ensure that the messages will not be lost.
Lazy loading
Lazy loading refers to deferring message processing until later to reduce system load or provide a better user experience. In PHP and MySQL development, we can use queue technology to implement lazy loading.
First, we create a data table named "delayed_messages" to save delayed loading messages.
CREATE TABLE delayed_messages ( id INT AUTO_INCREMENT PRIMARY KEY, content TEXT NOT NULL, delay_time INT NOT NULL DEFAULT 0, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
Then, we can use the following PHP code to save the lazy-loaded message to the database:
<?php // 连接到数据库 $connection = new mysqli('localhost', 'username', 'password', 'database'); // 插入延迟加载消息到数据库 $content = '这是一条延迟加载消息'; $delayTime = 60; // 延迟时间为60秒 $statement = $connection->prepare('INSERT INTO delayed_messages (content, delay_time) VALUES (?, ?)'); $statement->bind_param('si', $content, $delayTime); $statement->execute(); // 关闭数据库连接 $connection->close(); ?>
By saving the lazy-loaded message to the database, and setting the delay time, we can Lazy loading is achieved by processing messages at a later point in time.
Implementing delayed loading of messages requires a scheduled task (such as a cron task) to check the delayed loading messages in the database and send the messages to the consumer for processing when the delay time arrives. The following is a simple PHP code example:
<?php // 连接到数据库 $connection = new mysqli('localhost', 'username', 'password', 'database'); // 查询需要发送的延迟加载消息 $result = $connection->query('SELECT * FROM delayed_messages WHERE delay_time <= UNIX_TIMESTAMP()'); // 发送消息到消费者进行处理 while ($row = $result->fetch_assoc()) { $messageId = $row['id']; $content = $row['content']; // 执行消息处理逻辑 // ... // 删除已处理的消息 $connection->query("DELETE FROM delayed_messages WHERE id = $messageId"); } // 关闭数据库连接 $connection->close(); ?>
By regularly executing the above code through scheduled tasks, we can implement delayed loading message processing.
Conclusion
The application of queue technology in message persistence and lazy loading in PHP and MySQL is very important for optimizing system performance and providing a better user experience. By saving messages to the MySQL database, we can achieve persistent storage of messages to ensure that system failures or restarts will not cause message loss. At the same time, through lazy loading of queue technology, we can postpone messages to be processed later, thereby reducing the system load or providing a better user experience.
The above is the detailed content of Application of queue technology in message persistence and lazy loading in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!
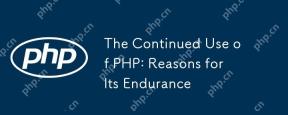
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
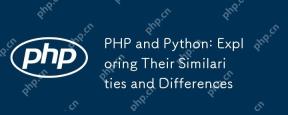
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
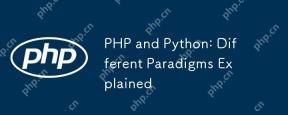
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
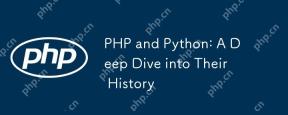
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
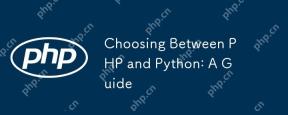
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
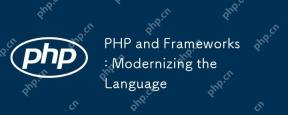
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
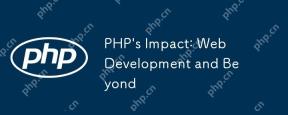
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
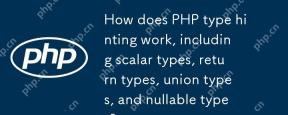
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
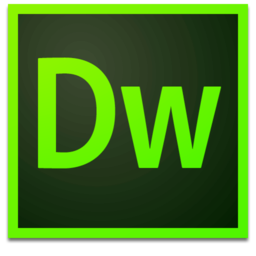
Dreamweaver Mac version
Visual web development tools
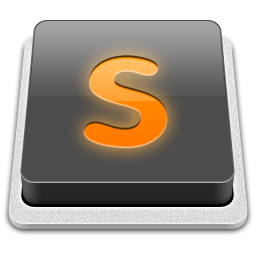
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
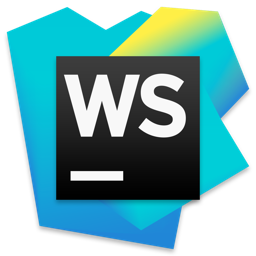
WebStorm Mac version
Useful JavaScript development tools