How to use custom instructions to implement special functions in Vue
How to use custom instructions in Vue to achieve special functions
In Vue development, custom instructions are a very useful function, which can help us achieve some Special needs. Custom instructions can add some DOM operations, event binding and other functions to Vue, allowing us to control and manage page elements more conveniently.
Below I will use a specific example to demonstrate how to use custom instructions to implement special functions in Vue.
Suppose we need to implement an auto-focus function in the input box, that is, when the page is loaded, the input box automatically gains focus. This can improve the user experience in some cases.
First, we need to define a custom instruction in Vue to implement the auto-focus function. In the instruction definition, we can use the hook function provided by Vue to listen to life cycle events and execute corresponding logic when specific events are triggered.
// 自定义指令定义 Vue.directive('autofocus', { // 当绑定元素插入到DOM中时被调用 inserted(el) { // 使用setTimeout延迟执行,确保视图已经渲染完成 setTimeout(() => { el.focus() // 输入框获取焦点 }, 0) } })
Next, in the Vue instance, we can use the v-autofocus directive to achieve the automatic focus effect. Just add this directive to the input box element.
<template> <input type="text" v-autofocus> </template>
With the above code, when the page is loaded, the input box will automatically gain focus.
In addition to the auto-focus function, we can also use custom instructions to achieve some other special needs, such as:
-
Anti-shake instructions: when the input box is continuously input , the event is only triggered some time after the input has stopped.
Vue.directive('debounce', { inserted(el, binding) { let timeout = null el.addEventListener('input', () => { clearTimeout(timeout) timeout = setTimeout(() => { binding.value() }, binding.arg || 500) }) } })
-
Scroll loading instructions: When the page scrolls to the bottom, automatically load more data or execute corresponding logic.
Vue.directive('scroll-load', { inserted(el, binding) { const handleScroll = () => { const { scrollTop, clientHeight, scrollHeight } = document.documentElement if (scrollTop + clientHeight >= scrollHeight - 10) { binding.value() } } window.addEventListener('scroll', handleScroll) } })
Through custom instructions, we can quickly implement some special functions and improve development efficiency and user experience. It should be noted that when using custom instructions, you must follow Vue's development principles to avoid maintenance and understanding difficulties caused by misuse of instructions.
To summarize, using custom instructions in Vue can easily implement some special functions, reduce code duplication and redundancy, and improve development efficiency. By rationally using custom directives, we can make Vue applications more flexible and feature-rich.
The above is the detailed content of How to use custom instructions to implement special functions in Vue. For more information, please follow other related articles on the PHP Chinese website!

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.

Vue.js and React each have their own advantages, and the choice should be based on project requirements and team technology stack. 1. Vue.js is community-friendly, providing rich learning resources, and the ecosystem includes official tools such as VueRouter, which are supported by the official team and the community. 2. The React community is biased towards enterprise applications, with a strong ecosystem, and supports provided by Facebook and its community, and has frequent updates.

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
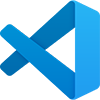
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
