


Understand the anti-shake mechanism in PHP and improve user experience
Understand the anti-shake mechanism in PHP and improve user experience
With the rapid development of the Internet, users have higher and higher requirements for the interactive experience of web pages. Some common interactive actions, such as input box input, button clicks, etc., if there is no appropriate processing mechanism, may lead to a decline in user experience. One of the important processing mechanisms is anti-shake.
What is the anti-shake mechanism? Simply put, anti-shake means that after the user triggers an action, it delays for a period of time before performing the corresponding operation. If the same action is triggered again within this delay time, the timing will be restarted and the operation will not be executed until the delay time expires. In this way, repeated operations can be effectively reduced and user experience improved.
In PHP, implementing the anti-shake mechanism is not complicated and can be accomplished by combining front-end and back-end technologies. Below, I will provide you with a specific code example to help you understand and apply the anti-shake mechanism.
First, we need to create a PHP file, such as debounce.php, to handle the anti-shake logic. In this file, we need to define a global variable to save the timestamp of the last operation. The code is as follows:
<?php // 上一次操作的时间戳 $lastActionTimestamp = 0; // 防抖处理函数 function debounce($callback, $delay) { global $lastActionTimestamp; // 获取当前时间戳 $currentTimestamp = time(); // 计算与上一次操作的时间间隔 $timeDiff = $currentTimestamp - $lastActionTimestamp; // 如果时间间隔小于延迟时间,则重新开始计时 if ($timeDiff < $delay) { return; } // 更新上一次操作的时间戳 $lastActionTimestamp = $currentTimestamp; // 执行回调函数 call_user_func($callback); }
In the above code, we define a function named debounce
, which accepts two parameters: callback function and delay time. This function will determine the time interval between the current time and the previous operation. If it is less than the delay time, it will return directly without executing the callback function. Otherwise, update the timestamp of the last operation and execute the callback function.
Next, we can call the debounce
function where the anti-shake mechanism needs to be applied. For example, when the user enters content in the input box, we can use the anti-shake mechanism to reduce the number of requests to the server. The code is as follows:
<?php // 引入 debounce.php 文件 require_once 'debounce.php'; // 输入框输入处理函数 function handleInput($value) { // 模拟请求服务端的操作 echo "请求服务端:$value"; } // 防抖处理 debounce(function() { // 获取输入框的值 $value = $_POST['value']; // 执行输入框输入处理函数 handleInput($value); }, 500);
In the above code, we first introduce the debounce.php file created previously. Then, define a function named handleInput
to actually process the input content of the input box. Next, the anti-shake logic is implemented by calling the debounce
function, in which a callback function and delay time are passed in. In the callback function, we get the value of the input box and call the handleInput
function to process the input content.
Through the above code examples, we can see how to use the anti-shake mechanism in PHP to improve user experience. When the user enters content in the input box, the anti-shake mechanism can ensure that the server will be requested only after a certain interval, thereby reducing unnecessary requests and improving the response speed of the web page and the user's interactive experience.
To sum up, understanding the anti-shake mechanism in PHP can help us optimize the user experience and improve the performance of web pages. By rationally using the anti-shake mechanism, unnecessary operation frequency can be effectively reduced, the load on the server can be reduced, and the smoothness of user operations on the web page can be improved. Hope the above is helpful to you!
The above is the detailed content of Understand the anti-shake mechanism in PHP and improve user experience. For more information, please follow other related articles on the PHP Chinese website!
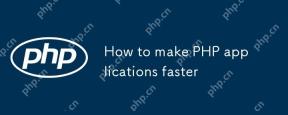
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
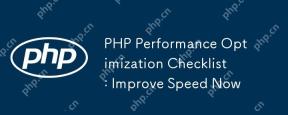
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
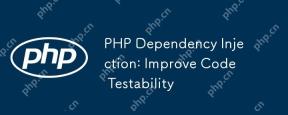
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
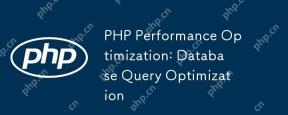
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
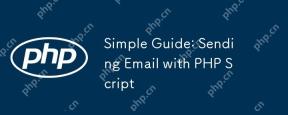
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
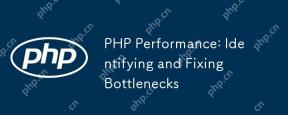
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
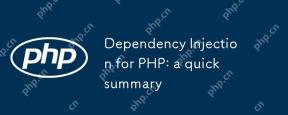
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
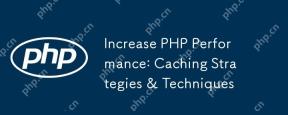
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
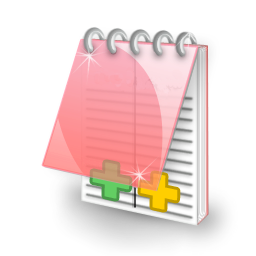
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
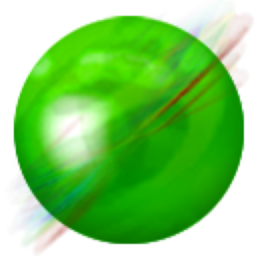
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
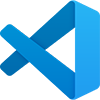
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
