


Comparison of implementation methods of PHP anti-shake and anti-duplicate submission
Comparison of implementation methods of PHP anti-shake and anti-duplicate submission
When developing web applications, we often encounter situations that require anti-shake (Debounce) and Preventing Duplicate Form Submission. Anti-shake means that when the user frequently triggers an event, we want to only execute the last triggered action, while anti-repetitive submission means that when the user submits the form multiple times in a short period of time, we need to ensure that only one submission is processed. This article will focus on comparing and introducing several ways to achieve anti-shaking and anti-resubmission in PHP, and provide specific code examples.
- How to implement anti-shake
Anti-shake can be set up by setting a timer, and after the last trigger event, the corresponding operation will be performed after a certain period of delay. The following is a sample code that uses PHP to achieve anti-shake:
function debounce($callback, $delay) { $timer = null; return function() use ($callback, $delay, &$timer) { if ($timer !== null) { clearTimeout($timer); } $timer = setTimeout($callback, $delay); }; } // 使用防抖函数处理表单提交事件 $debouncedHandler = debounce(function() { // 处理表单提交逻辑 }, 1000); // 绑定事件处理函数 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $debouncedHandler(); }
- How to prevent repeated submission
Preventing repeated submission of forms can be achieved in the following ways:
2.1. Token verification
By using Token verification, we can generate a unique identifier for each form request and store the identifier in the session. When the user submits the form, we first check whether the identifier exists and whether it is consistent with the identifier in the request to determine whether it is a repeated submission. The following is a sample code that uses PHP to implement Token verification:
session_start(); function generateToken() { return md5(uniqid(rand(), true)); } function validateToken($token) { // 从 session 中获取 token $storedToken = $_SESSION['token']; return $storedToken && $token === $storedToken; } function removeToken() { // 从 session 中移除 token unset($_SESSION['token']); } // 生成 Token 并存储在 session 中 $_SESSION['token'] = generateToken(); // 处理表单提交逻辑 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $token = $_POST['token']; if (validateToken($token)) { // 执行表单提交操作 // ... // 提交完成后移除 Token removeToken(); } else { // Token 验证失败,可能是重复提交 // 提示用户不要重复提交 } }
2.2. Redirection
Using redirection to handle page jumps after form submission can effectively prevent users from repeatedly submitting the form. When the user submits the form, we first handle the submission logic and then use the header function to redirect the user to a new page. The following is a sample code that uses PHP to implement redirection:
// 处理表单提交逻辑 if ($_SERVER['REQUEST_METHOD'] === 'POST') { // 执行表单提交操作 // ... // 重定向到新页面 header("Location: success.php"); exit; }
success.php
in the above code is a page that prompts the user to submit successfully, so that even if the user presses the refresh button, the Only the success.php
page will be refreshed and the form will not be submitted again.
Summary:
By comparing the implementation methods of PHP anti-shaking and anti-duplicate submission, we can choose the appropriate method to protect our application according to specific needs. Anti-shake is suitable for situations where users need to avoid triggering events frequently, while preventing repeated submission of forms is to prevent users from submitting forms multiple times. Using Token verification and redirection are two common and feasible methods. Developers can choose the appropriate method to prevent repeated submissions based on the actual situation.
The above is the detailed content of Comparison of implementation methods of PHP anti-shake and anti-duplicate submission. For more information, please follow other related articles on the PHP Chinese website!
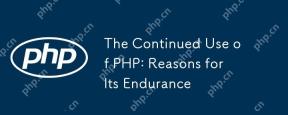
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
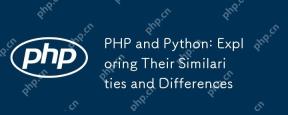
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
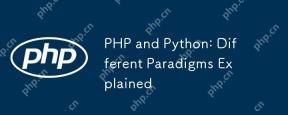
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
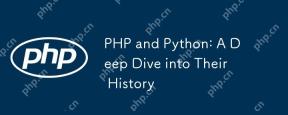
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
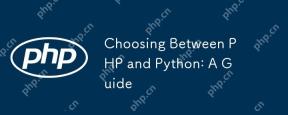
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
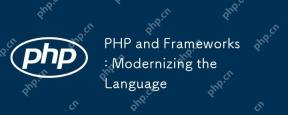
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
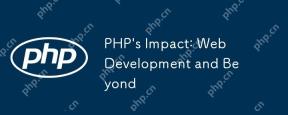
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
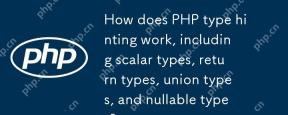
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor