Analysis and repair solutions for common data type problems in C++
Analysis and repair solutions for common data type problems in C
Abstract:
In C language, data type is a very important concept. Proper data type selection and use can improve program performance and robustness. However, some common data type problems still arise and can cause program errors or inefficiencies. This article will analyze several common data type problems and provide corresponding fixes and code examples.
- Integer overflow
In C, integer types have range restrictions. If an integer variable exceeds the range it can hold, overflow occurs. Overflows can cause unexpected results or undefined behavior. The following is an example of an integer overflow:
int a = INT_MAX; int b = a + 1; // 溢出发生 cout << "a: " << a << endl; cout << "b: " << b << endl; // b的值是未定义的
Fix:
You can use a larger integer type, such as long long
, to avoid overflow. In addition, appropriate bounds checking can be performed to prevent overflows from occurring.
#include <limits> long long a = INT_MAX; long long b = a + 1; // 不会发生溢出 if (b > std::numeric_limits<int>::max()) { // 处理溢出情况的代码 } cout << "a: " << a << endl; cout << "b: " << b << endl; // 正常输出
- Floating point number precision issue
In C, floating point number types are represented approximately. Due to the limited precision of floating point numbers, some precision issues may result. Here is an example of a floating point precision problem:
float a = 0.1; float b = 0.2; float c = 0.3; if (a + b == c) { // 不一定会进入这里 cout << "Equal" << endl; } else { cout << "Not Equal" << endl; }
Fix:
You can use an error margin to compare floating point numbers for equality instead of directly comparing their values. For example, you can use the std::abs function to calculate the difference between two floating point numbers and compare it to a small error margin.
#include <cmath> float a = 0.1; float b = 0.2; float c = 0.3; float epsilon = 0.0001; // 误差范围 if (std::abs(a + b - c) < epsilon) { cout << "Equal" << endl; } else { cout << "Not Equal" << endl; }
- String length issue
In C, a string is a character array terminated by a null character. If the length of the string is not handled correctly, buffer overflows and memory errors can result. The following is an example of a string length problem:
char str[10] = "Hello, World!"; // 长度超过数组大小
Fix:
You can use string classes to handle strings, such as std::string. Use the std::string class to dynamically allocate memory and automatically handle string lengths. Make sure the length of the string does not exceed the allocated memory.
#include <string> std::string str = "Hello, World!";
Conclusion:
In C, the correct selection and use of data types is the key to writing high-quality code. This article analyzes integer overflow, floating point precision issues, and string length issues, and provides corresponding fixes and code examples. Programmers should be fully aware of these issues and take appropriate precautions to avoid potential errors and inefficiencies.
The above is the detailed content of Analysis and repair solutions for common data type problems in C++. For more information, please follow other related articles on the PHP Chinese website!
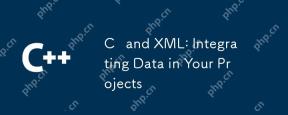
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
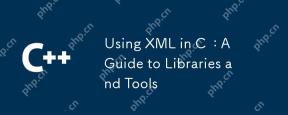
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
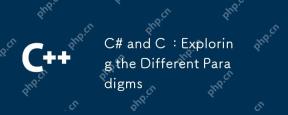
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
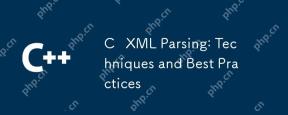
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
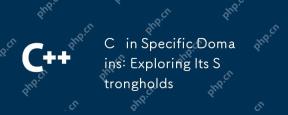
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
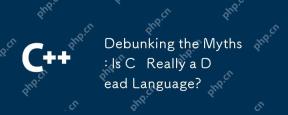
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
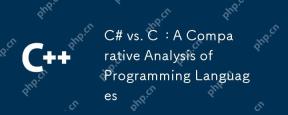
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
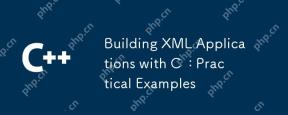
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
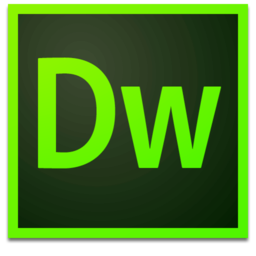
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
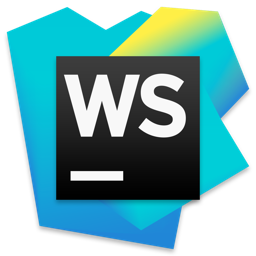
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
