Detailed explanation of operator overloading in C
Operator overloading is a powerful and useful feature in C. By overloading operators, objects of a certain class can be made You can use various operators just like basic type data to perform various operations conveniently. This article explains the concept of operator overloading in detail and provides concrete code examples.
In C, operator overloading is achieved by defining member functions or global functions of the class. The name of an operator overloaded function consists of the keyword operator and a symbol. For example, the name of a function that overloads the addition operator is operator. Through operator overloading, we can define addition, subtraction, multiplication, division and other operations between objects, as well as operations between objects and basic data types.
The specific code examples are as follows. First, we define a complex number class named Complex and overload the addition and subtraction operators:
class Complex { private: double real; double imag; public: Complex(double r = 0, double i = 0) : real(r), imag(i) {} Complex operator+(const Complex& c) { return Complex(real + c.real, imag + c.imag); } Complex operator-(const Complex& c) { return Complex(real - c.real, imag - c.imag); } }; int main() { Complex a(3, 4); Complex b(1, 2); Complex c = a + b; Complex d = a - b; cout << "c = " << c.real << " + " << c.imag << "i" << endl; cout << "d = " << d.real << " + " << d.imag << "i" << endl; return 0; }
In the above example, we define a Complex class , which contains two member variables real and imag, which represent the real part and imaginary part of the complex number respectively. By overloading the addition operator and the subtraction operator -, complex number objects can be added and subtracted like ordinary integers or floating point numbers.
In the main function, we define two Complex type objects a and b, and assign the results of their addition and subtraction to c and d respectively. Then use the cout statement to output the results.
In addition, in addition to member function overloading operators, we can also overload operators through global functions. For example, we can overload the auto-increment operator so that objects of a class can implement increment operations through the auto-increment operator. The specific code example is as follows:
class Counter { private: int count; public: Counter(int c = 0) : count(c) {} Counter operator++() { return Counter(++count); } }; int main() { Counter c(5); ++c; cout << "count: " << c.getCount() << endl; return 0; }
In the above example, we defined a Counter class, which contains a member variable count. By overloading the prefixed increment operator, we enable objects of the Counter class to use the operator to implement increment operations. In the main function, we create a Counter object c, implement the increment operation through c, and finally use the cout statement to output the result.
Through the above code example, we can see that through operator overloading, we can make objects of custom classes use various operators like basic data types and perform various operations conveniently. However, when using operator overloading, we also need to pay attention to the syntax rules and usage restrictions of operator overloading to avoid errors or unpredictable results.
To summarize, operator overloading is a powerful and useful feature in C. Through operator overloading, we can define various operations between objects of a class. This article provides specific code examples, hoping to help readers better understand the concept and usage of operator overloading.
The above is the detailed content of Detailed explanation of operator overloading in C++. For more information, please follow other related articles on the PHP Chinese website!
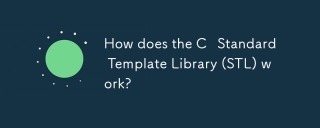
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
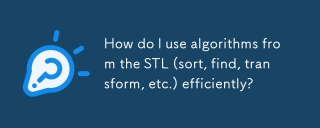
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
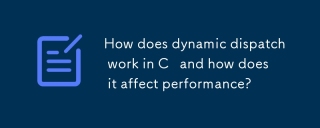
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
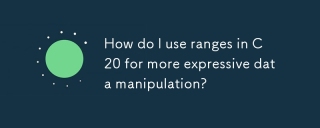
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
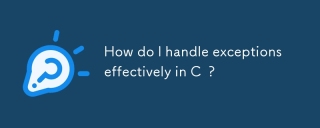
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
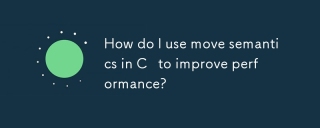
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
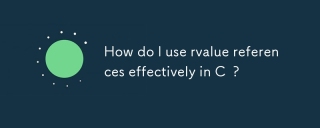
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
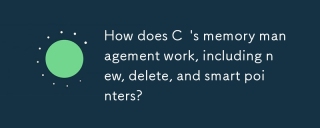
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
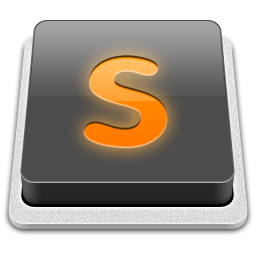
SublimeText3 Mac version
God-level code editing software (SublimeText3)
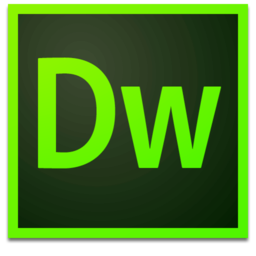
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
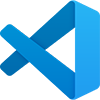
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
