


How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
Efficiently using STL algorithms hinges on understanding their underlying mechanics and applying best practices. Firstly, ensure your data is appropriately organized. For algorithms like sort
, using a vector (dynamic array) is generally more efficient than a list (doubly linked list) because vectors provide contiguous memory access, crucial for many sorting algorithms. Lists require pointer traversal, making sorting significantly slower.
Secondly, understand the algorithm's complexity. sort
typically uses an introspective sort (a hybrid of quicksort, heapsort, and insertion sort) with O(n log n) average-case complexity. However, if you know your data is nearly sorted, std::partial_sort
or even a simple insertion sort might be faster. Similarly, find
has linear O(n) complexity; if you need frequent searches, consider using a std::set
or std::unordered_set
(for unsorted and sorted data respectively) which offer logarithmic or constant time complexity for lookups.
Thirdly, use iterators effectively. STL algorithms operate on iterators, not containers directly. Passing iterators to the beginning and end of a range avoids unnecessary copying of data, improving performance, especially for large datasets. For example, instead of std::sort(myVector)
, use std::sort(myVector.begin(), myVector.end())
. Use the correct iterator type (e.g., const_iterator
if you don't need to modify the data).
Finally, consider using execution policies. For algorithms supporting parallel execution (like std::sort
), using execution policies like std::execution::par
or std::execution::par_unseq
can significantly speed up processing on multi-core machines, especially for large datasets. However, remember that the overhead of parallelization might outweigh the benefits for small datasets.
What are the common pitfalls to avoid when using STL algorithms?
Several common pitfalls can hinder the efficiency and correctness of STL algorithm usage:
- Incorrect iterator ranges: Providing incorrect start or end iterators is a frequent error, leading to undefined behavior or incorrect results. Always double-check your iterator ranges.
- Modifying containers during algorithm execution: Modifying the container being processed by an algorithm (e.g., adding or removing elements) while the algorithm is running can lead to unpredictable results, crashes, or data corruption.
- Ignoring algorithm preconditions: Many STL algorithms have preconditions (e.g., sorted input for certain algorithms). Failing to meet these preconditions can result in incorrect output or undefined behavior.
-
Inefficient data structures: Choosing the wrong data structure for the task can significantly impact performance. For example, using a
std::list
when astd::vector
would be more appropriate for frequent random access. - Unnecessary copies: Avoid unnecessary copying of data. Use iterators to process data in-place whenever possible.
- Overuse of algorithms: For simple operations, a custom loop might be more efficient than using a general-purpose STL algorithm. Profiling your code can help determine if an STL algorithm is truly necessary.
How can I choose the most efficient STL algorithm for a specific task?
Selecting the most efficient STL algorithm requires understanding the task's requirements and the algorithms' characteristics:
- Identify the operation: Determine what needs to be done (sorting, searching, transforming, etc.).
- Analyze the data: Consider the data's size, organization (sorted, unsorted), and properties.
-
Choose the appropriate algorithm: Based on the operation and data characteristics, select the algorithm with the best time and space complexity. For example, for searching in a sorted range,
std::lower_bound
orstd::binary_search
are more efficient thanstd::find
. For transforming data, considerstd::transform
orstd::for_each
. - Consider parallelization: If the dataset is large and the algorithm supports parallel execution, explore using execution policies for potential performance gains.
- Profile and benchmark: After choosing an algorithm, measure its performance using profiling tools to ensure it meets your requirements. Compare different algorithms to validate your choice.
Are there performance differences between different STL algorithms for the same task, and how can I measure them?
Yes, significant performance differences can exist between different STL algorithms designed for similar tasks. For instance, std::sort
might outperform a custom insertion sort for large, unsorted datasets, but the custom sort might be faster for small, nearly-sorted datasets. Similarly, std::find
is linear, while searching a std::set
is logarithmic.
To measure these differences, use profiling tools and benchmarking techniques:
- Profiling tools: Tools like gprof (for Linux) or Visual Studio Profiler (for Windows) can help identify performance bottlenecks in your code, showing the time spent in different functions, including STL algorithms.
-
Benchmarking: Create test cases with varying data sizes and characteristics. Time the execution of different algorithms using high-resolution timers (e.g.,
std::chrono
in C ). Repeat the measurements multiple times and average the results to minimize noise. - Statistical analysis: Use statistical methods to compare the performance results and determine if the differences are statistically significant.
By combining profiling and benchmarking, you can accurately assess the performance of different STL algorithms and make informed decisions for your specific needs. Remember to test with representative datasets to get meaningful results.
The above is the detailed content of How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?. For more information, please follow other related articles on the PHP Chinese website!
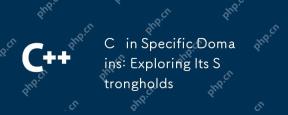
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
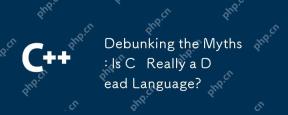
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
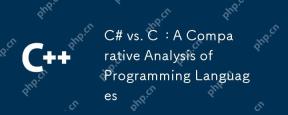
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
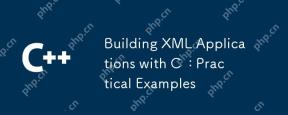
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
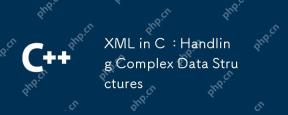
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
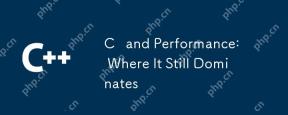
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
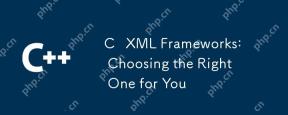
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
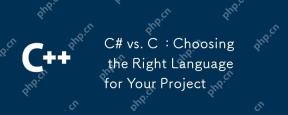
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
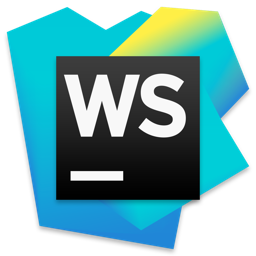
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
