


How to solve the problem of graceful shutdown and smooth restart of concurrent tasks in Go language?
How to solve the problem of graceful shutdown and smooth restart of concurrent tasks in Go language?
In the Go language, we often encounter situations where we need to handle concurrent tasks. However, the shutdown and restart of concurrent tasks may cause problems such as resource leakage or data loss. In order to solve these problems, we need to adopt some graceful shutdown and smooth restart methods.
1. Graceful shutdown
When faced with a scenario where concurrent tasks need to be stopped, we hope to allow all tasks to complete the current operation and exit safely after receiving the stop signal. The following is a sample code that uses channels to achieve graceful shutdown:
package main import ( "fmt" "os" "os/signal" "syscall" ) func main() { // 创建一个通道用于接收停止信号 stop := make(chan os.Signal, 1) // 使用通道接收所有停止信号 signal.Notify(stop, syscall.SIGINT, syscall.SIGTERM) // 模拟并发任务 go func() { for { fmt.Println("Doing something...") } }() // 等待停止信号 <-stop fmt.Println("Stopping...") // 执行停止操作,如关闭数据库连接,释放资源等 fmt.Println("Stopped") }
In the above code, we register the stop signal that needs to be received by calling the signal.Notify
function. Here we choose SIGINT
and SIGTERM
signals. Then, we started a concurrent task and continuously output a sentence. Finally, will block and wait for the stop signal. Once the stop signal is received, the program will perform the stop operation and output relevant information.
2. Smooth Restart
In some cases, we hope to be able to reload the configuration file or perform some hot update operations without stopping the entire application. The following is a sample code that uses the graceful
library to achieve smooth restart:
First, we need to pass in our listening address through parameters on the command line:
$ go run main.go -addr=:8080
Then, In the code, we can achieve the smooth restart effect by listening to the USR2
signal of the operating system:
package main import ( "flag" "fmt" "log" "net" "net/http" "os" "os/signal" "syscall" "time" "github.com/tylerb/graceful" ) var ( addr string ) func main() { flag.StringVar(&addr, "addr", ":8080", "server address") flag.Parse() // 创建一个HTTP服务器 server := &graceful.Server{ Timeout: 10 * time.Second, Server: &http.Server{ Addr: addr, Handler: http.HandlerFunc(handler), }, } // 启动HTTP服务器 go func() { log.Printf("Listening on %s ", addr) if err := server.ListenAndServe(); err != nil { log.Fatal(err) } }() // 监听USR2信号 stop := make(chan os.Signal, 1) signal.Notify(stop, syscall.SIGUSR2) // 等待USR2信号 <-stop log.Println("Received signal to reload") // 重启HTTP服务器 err := server.Close() if err != nil { log.Fatal(err) } log.Println("Reloading server...") // 执行重启操作,如重新加载配置文件 time.Sleep(1 * time.Second) log.Println("Server reloaded") // 重新启动HTTP服务器 go func() { log.Printf("Listening on %s ", addr) if err := server.ListenAndServe(); err != nil { log.Fatal(err) } }() // 等待停止信号 <-stop log.Println("Stopping server...") // 停止HTTP服务器 err = server.Stop(10 * time.Second) if err != nil { log.Fatal(err) } log.Println("Server stopped") } func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello, world!") }
In the above code, we first pass graceful.NewServer
The function creates an HTTP server and sets some related configurations. Then, we listened to the USR2
signal through the signal.Notify
function. When receiving this signal, we first shut down the current HTTP server and perform restart operations, such as reloading the configuration file. Finally, we start the HTTP server again.
Through the above code examples, we can see how to achieve graceful shutdown and smooth restart of concurrent tasks in Go language. These methods can help us solve problems that concurrent tasks may encounter when shutting down and restarting, and ensure the stability and reliability of the program.
The above is the detailed content of How to solve the problem of graceful shutdown and smooth restart of concurrent tasks in Go language?. For more information, please follow other related articles on the PHP Chinese website!
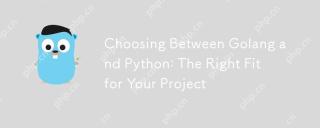
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
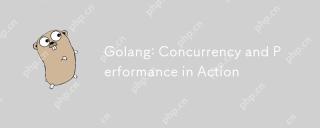
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
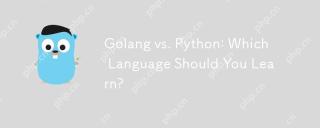
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
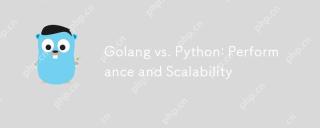
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
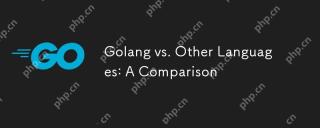
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
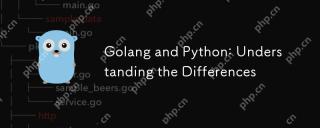
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
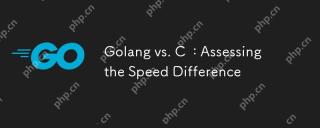
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
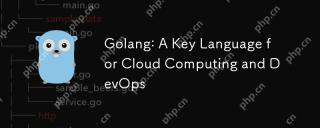
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
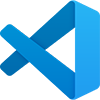
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
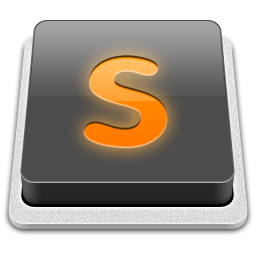
SublimeText3 Mac version
God-level code editing software (SublimeText3)