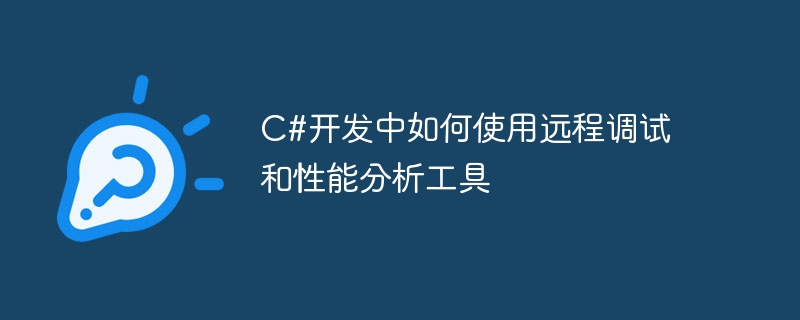
How to use remote debugging and performance analysis tools in C# development
Introduction:
In the C# development process, remote debugging and performance analysis tools can help us solve problems Some difficult to debug issues and optimize program performance. This article will introduce in detail how to use remote debugging tools and performance analysis tools, and provide specific code examples.
1. Remote debugging tool
Remote debugging tool allows us to debug running programs on a remote computer. This is useful for solving problems that only occur in certain environments. Here are the steps to use the remote debugging tools in Visual Studio:
- Install Visual Studio Remote Tools on the target remote computer. This can be downloaded from the official Microsoft website.
- Open the project that needs to be debugged in Visual Studio, right-click the project name, and select the "Properties" option.
- In the properties window, select the "Debug" tab.
- In the "Debugger Launcher" option, select "Remote Windows Debugging".
- In the "Remote Computer" option, enter the name or IP address of the remote computer.
- Click "OK" to save the settings.
- Select "Attach to Process" in the "Debug" menu.
- In the "Attach to Process" dialog box, select the program running on the target remote computer and click the "Attach" button.
- Next, we can debug the program on the remote computer just like we debug on the local computer.
Example:
The following is a simple example to illustrate how to use the remote debugging tool. Suppose we have two computers, one is local and the other is remote. We want to run and debug a C# console application on a remote computer.
- Create a C# console application on the local computer, such as "RemoteDebugSample".
- Modify the application's code to the following:
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
int a = 10;
int b = 0;
int c = a / b;
Console.WriteLine("Result: " + c);
}
}
- Build and publish the executable file of this application.
- Copy the executable file to the remote computer.
- In Visual Studio on the local computer, follow the steps above to set up the remote debugging tool.
- Run the application on the remote computer.
- Enter Visual Studio on the local computer and select "Attach to Process" in the "Debug" menu.
- In the "Attach to Process" dialog box, select the application running on the remote computer and click the "Attach" button.
- The program will stop at the statement where the divisor is 0.
- We can view the values of variables, inspect the call stack, and use other debugger features.
2. Performance analysis tools
Performance analysis tools can help us find the performance bottlenecks of the program and provide optimization suggestions. The following describes the steps to use Visual Studio's performance analysis tool:
- Open the project that needs to be analyzed in Visual Studio.
- In the "Analysis" menu, select "Performance Profiler".
- In the "Performance Profiler" window, click the "Start Performance Analysis" button.
- In the pop-up "Start Performance Analysis Session" dialog box, select the "CPU Sampling" option and click the "Start" button.
- Operate the program during the time period for which performance needs to be measured.
- To stop performance analysis, click the "Stop" button.
- In the "Performance Profiler" window, hover the mouse over a function to see the execution time and number of calls of the function.
- According to the performance analysis results, the program can be optimized.
Example:
The following is a simple example to illustrate how to use the performance analysis tool. Let's say we have a C# application and there is a performance issue in a certain function.
- In the C# application, find the function that needs to analyze performance, such as "CalculateAverage".
- Modify the code of the function to the following:
double CalculateAverage(int[] numbers)
{
double sum = 0;
for (int i = 0; i < numbers.Length; i++)
{
sum += numbers[i];
}
return sum / numbers.Length;
}
- Follow the above steps in Visual Studio to start a performance analysis session and operate the application.
- Stop performance analysis.
- In the "Performance Profiler" window, find the "CalculateAverage" function and view its execution time and number of calls.
- Based on the performance analysis results, the code of the function can be optimized to improve performance.
Conclusion:
This article introduces how to use remote debugging tools and performance analysis tools in C# development. Remote debugging tools can help us debug programs on remote computers and solve problems that occur in specific environments. Performance analysis tools can help us find the performance bottlenecks of the program and provide optimization suggestions. Mastering these tools can improve our development efficiency and program performance.
(Note: The code example is for illustration only and needs to be modified according to the actual situation.)
The above is the detailed content of How to use remote debugging and performance analysis tools in C# development. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn