Implementation of C++ polymorphism and analysis of common problems
Realization of C Polymorphism and Analysis of Common Problems
Introduction:
Polymorphism is an important feature of object-oriented programming languages, and it is also used in C Has been widely used. Polymorphism allows different types of objects to be processed in the same way, improving code flexibility and maintainability. This article will introduce the implementation of polymorphism in C and analyze common polymorphism problems.
1. How to implement polymorphism
- Virtual functions (Virtual Functions)
Virtual functions are the basis of polymorphism in C. By declaring a member function of a base class as a virtual function, you can override it in a derived class. When a virtual function is called through a pointer or reference to a base class object, the function in the derived class is actually executed. The following is a sample code:
class Shape{ public: virtual void draw() { cout << "This is a shape." << endl; } }; class Circle : public Shape{ public: void draw() { cout << "This is a circle." << endl; } }; class Rectangle : public Shape{ public: void draw() { cout << "This is a rectangle." << endl; } }; int main(){ Shape* shape = new Circle(); shape->draw(); // 输出 "This is a circle." shape = new Rectangle(); shape->draw(); // 输出 "This is a rectangle." delete shape; return 0; }
- Pure Virtual Functions and Abstract Classes
Pure virtual functions refer to those declared in the base class but not implemented Virtual functions are marked with "= 0". Pure virtual functions are only used for implementation in derived classes, base classes cannot instantiate objects. In C, a class containing pure virtual functions is called an abstract class. Abstract classes cannot be instantiated directly and can only be instantiated and used through derived classes. The following is a sample code:
class Shape{ public: virtual void draw() = 0; }; class Circle : public Shape{ public: void draw() { cout << "This is a circle." << endl; } }; class Rectangle : public Shape{ public: void draw() { cout << "This is a rectangle." << endl; } }; int main(){ Shape* shape = new Circle(); shape->draw(); // 输出 "This is a circle." shape = new Rectangle(); shape->draw(); // 输出 "This is a rectangle." delete shape; return 0; }
2. Analysis of common problems
- Pointer type issues
When using polymorphism, you need to pay attention to pointer type issues. Since a derived class object can be assigned to a pointer or reference pointing to a base class object, when a method is called through a virtual function, the function to be called will be determined based on the pointer type. If the pointer type is incorrect, the correct derived class function will not be called. Here is an example:
class Shape{ public: virtual void draw(){ cout << "This is a shape." << endl; } }; class Circle : public Shape{ public: void draw(){ cout << "This is a circle." << endl; } }; class Rectangle : public Shape{ public: void draw(){ cout << "This is a rectangle." << endl; } }; int main(){ Shape* shape = new Shape(); shape->draw(); // 输出 "This is a shape." shape = new Circle(); shape->draw(); // 输出 "This is a circle." shape = new Rectangle(); shape->draw(); // 输出 "This is a rectangle." delete shape; return 0; }
- Calling order issue
In polymorphism, the calling order of virtual functions is determined based on the actual type of the pointer or reference. If you call a virtual function in a constructor or destructor, it may lead to unexpected results. This is because the type of the object is determined when the constructor or destructor is called, and the call to the virtual function is based on subsequent assignment operations. The following is an example:
class Shape{ public: Shape(){ draw(); // 虚函数调用 } virtual void draw(){ cout << "This is a shape." << endl; } }; class Circle : public Shape{ public: void draw(){ cout << "This is a circle." << endl; } }; int main(){ Shape* shape = new Circle(); shape->draw(); // 输出 "This is a shape." 和 "This is a circle." delete shape; return 0; }
Summary:
This article introduces the implementation of polymorphism in C and analyzes common polymorphism issues. By understanding the basic concepts and usage of polymorphism, you can improve the flexibility and maintainability of your code and better cope with daily development needs. However, when using polymorphism, you need to pay attention to issues such as pointer types and calling order to avoid unexpected results. I hope this article can help readers better understand and apply polymorphism.
The above is the detailed content of Implementation of C++ polymorphism and analysis of common problems. For more information, please follow other related articles on the PHP Chinese website!
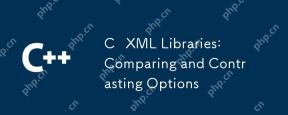
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
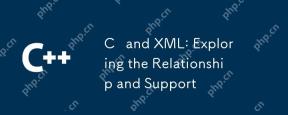
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
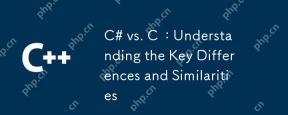
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
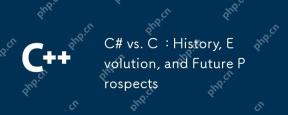
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
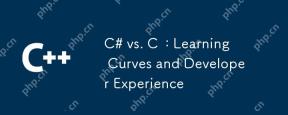
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
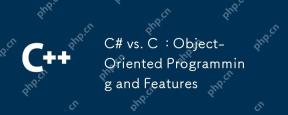
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
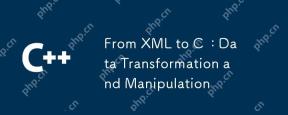
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
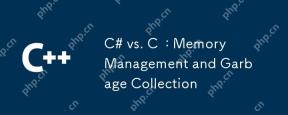
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
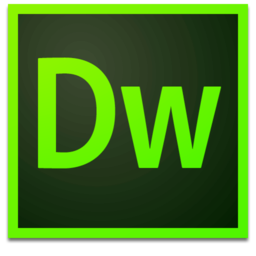
Dreamweaver Mac version
Visual web development tools
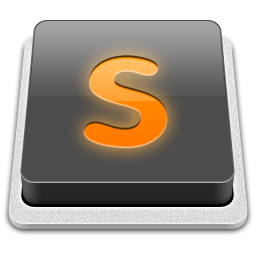
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
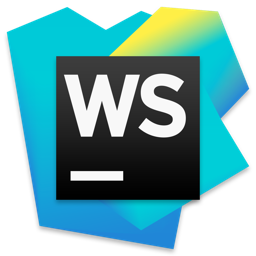
WebStorm Mac version
Useful JavaScript development tools