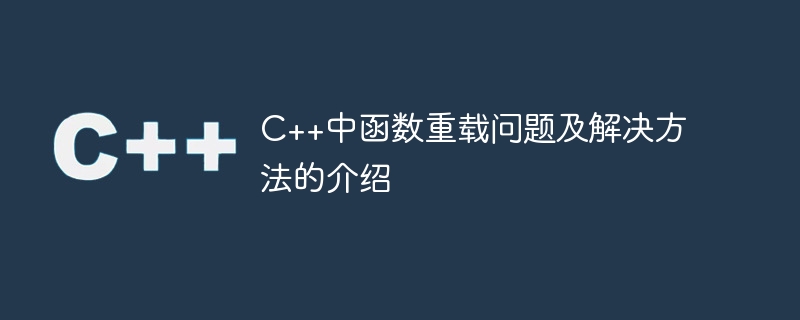
Introduction to function overloading problems and solutions in C
In C, function overloading refers to using the same function name in the same scope. However, it is a mechanism to define multiple functions when the type, number or order of function parameters are different. Through function overloading, we can provide different implementations for the same operation or function to meet different needs.
However, function overloading may also cause some problems. For example, when calling a function with a similar function signature, the compiler may not be able to determine which function to call, resulting in compilation errors. The following will introduce some solutions to the function overloading problem in C and give examples.
- Function overload resolution
Function overload resolution refers to the process by which the compiler determines which function to call based on the type, number and order of the actual parameters when calling an overloaded function. When performing function overload resolution, the compiler will match possible candidate functions one by one according to certain rules until the best matching function is found or resolution failure occurs.
The resolution process of function overloading follows the following principles:
- Exact match: If a function completely matches the type, number and order of the actual parameters, then this The function will be selected as a candidate function.
- Implicit type conversion: If the parameter type of a function does not exactly match the actual parameter type, but there is an implicit type conversion that can make it match, then this function will also be selected as a candidate function.
- Default parameter matching: If the number of parameters of a function is more than the actual number of parameters, and the extra parameters have default values, then this function will also be selected as a candidate function.
Give an example to illustrate the resolution process of function overloading:
void foo(int x) { cout << "int" << endl; }
void foo(double x) { cout << "double" << endl; }
void foo(char x) { cout << "char" << endl; }
int main() {
foo(10); // 会调用foo(int)
foo(3.14); // 会调用foo(double)
foo('a'); // 会调用foo(char)
return 0;
}
In the above code, the function foo
is overloaded three times, accepting# respectively. Parameters of type ##int,
double, and
char. In the
main function, the integer
10, floating point number
3.14 and character
'a' are passed in to call
fooFunction. According to the resolution rules of function overloading, the compiler will select the best matching function to call based on the type of the actual parameters.
Function overloading ambiguity- Sometimes, function overloading will cause the compiler to be unable to determine which function to call, resulting in function overloading ambiguity. Function overloading ambiguity usually occurs when there are multiple candidate functions with equal matching degrees, and the specific function cannot be clearly selected through the actual parameters when calling the function.
To resolve function overloading ambiguity, you can take one of the following approaches:
Explicit type conversion: by explicitly using the type conversion operator in the function call or Make a type conversion function call and specify the specific intention of the function call. For example, - foo(static_cast(10))
.
Function pointer overloading solution: Use function pointer variables to call specific functions to avoid function overloading ambiguity. When defining a function pointer variable, you need to explicitly specify the candidate function that needs to be called. For example, - void (*pFoo)(int) = foo; pFoo(10);
.
Function renaming: Give a function with overload ambiguity a new and distinctive name to eliminate ambiguity. For example, rename the - foo
function to
fooInt,
fooDouble, and
fooChar.
The following example demonstrates function overloading ambiguity and its resolution:
void foo(int x) { cout << "int" << endl; }
void foo(double x) { cout << "double" << endl; }
int main() {
foo(10); // 函数重载歧义,编译错误
foo(3.14); // 函数重载歧义,编译错误
foo(static_cast<double>(10)); // 使用显示类型转换解决
return 0;
}
In the above code, there are two candidate functions
foo(int)and
foo(double), their matching degrees are equal. When calling
foo(10) and
foo(3.14), the compiler cannot determine which function to call, resulting in a compilation error. To resolve function overloading ambiguity, we can use explicit type casting to explicitly specify the called function, such as
foo(static_cast(10)).
Through the above introduction, we have learned about the solution to the function overloading problem in C, and explained it through specific code examples. Function overloading can enhance the flexibility and readability of the program. Proper use of function overloading under appropriate circumstances can improve the reusability and maintainability of the code.
The above is the detailed content of Introduction to function overloading problems and solutions in C++. For more information, please follow other related articles on the PHP Chinese website!