PHP study notes: WeChat applet and public account development
PHP study notes: WeChat applet and public account development
With the rapid development of the mobile Internet, WeChat has become one of the most widely used social media platforms. . In order to meet the needs of users, WeChat provides two development methods: mini programs and official accounts. This article will introduce how to use PHP language to develop WeChat mini programs and public accounts, and provide some specific code examples.
1. WeChat Mini Program Development
- Preparation work
First, we need to apply for a Mini Program account on the WeChat public platform and obtain the Mini Program account The AppID and AppSecret of the program. Then, build a PHP development environment locally and ensure that the PHP running environment and related extension libraries are installed.
- Mini program login
Mini program login is an important function in mini program development. You can use the login API provided by WeChat to implement the user login and registration functions of the mini program. The following is a simple sample code:
<?php // 获取小程序登录凭证code $code = $_GET['code']; // 调用接口,获取session_key和openid $url = "https://api.weixin.qq.com/sns/jscode2session?appid=YOUR_APPID&secret=YOUR_APP_SECRET&js_code=$code&grant_type=authorization_code"; $response = file_get_contents($url); $result = json_decode($response, true); $session_key = $result['session_key']; $openid = $result['openid']; // 根据openid查询用户信息,如果不存在则注册新用户 // ... ?>
- Data operation
Mini programs usually need to interact with the background database for data, and the PHP language can be used to operate the database. The following is a sample code using a MySQL database:
<?php // 连接数据库 $mysqli = new mysqli('localhost', 'username', 'password', 'dbname'); // 查询数据 $query = "SELECT * FROM users"; $result = $mysqli->query($query); // 处理查询结果 while ($row = $result->fetch_assoc()) { echo $row['name']; } // 插入数据 $name = $_POST['name']; $age = $_POST['age']; $query = "INSERT INTO users (name, age) VALUES ('$name', '$age')"; $mysqli->query($query); // 更新数据 $id = $_POST['id']; $name = $_POST['name']; $query = "UPDATE users SET name='$name' WHERE id=$id"; $mysqli->query($query); // 删除数据 $id = $_POST['id']; $query = "DELETE FROM users WHERE id=$id"; $mysqli->query($query); // 关闭数据库连接 $mysqli->close(); ?>
2. WeChat public account development
- Preparation work
Similarly, we need to create a WeChat public account The platform applies for a public account and obtains the AppID and AppSecret of the public account. Then, configure the URL and Token in the official account settings for message reception and verification.
- Receive messages
The public account can receive text messages, picture messages, audio messages, etc. sent by users. The following is a sample code for receiving text messages:
<?php // 验证消息的合法性 $signature = $_GET['signature']; $timestamp = $_GET['timestamp']; $nonce = $_GET['nonce']; $token = 'YOUR_TOKEN'; $tmpArr = array($token, $timestamp, $nonce); sort($tmpArr); $tmpStr = implode('', $tmpArr); $tmpStr = sha1($tmpStr); if ($tmpStr == $signature) { // 验证成功 // 处理接收的消息 $postStr = file_get_contents('php://input'); $postObj = simplexml_load_string($postStr, 'SimpleXMLElement', LIBXML_NOCDATA); $type = $postObj->MsgType; switch ($type) { case 'text': $content = $postObj->Content; echo "接收到文本消息:$content"; break; // 其他类型的消息 // ... } } else { // 验证失败 echo "验证失败"; } ?>
- Send message
Official accounts can send text messages, picture messages, graphic messages, etc. to users. The following is a sample code for sending text messages:
<?php // 发送文本消息 $access_token = 'YOUR_ACCESS_TOKEN'; $openid = 'USER_OPENID'; $content = 'Hello, World!'; $url = "https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token=$access_token"; $data = array( 'touser' => $openid, 'msgtype' => 'text', 'text' => array('content' => $content) ); $options = array( 'http' => array( 'method' => 'POST', 'header' => 'Content-Type:application/json', 'content' => json_encode($data) ) ); $context = stream_context_create($options); $response = file_get_contents($url, false, $context); $result = json_decode($response, true); if ($result['errcode'] == 0) { echo "发送成功"; } else { echo "发送失败"; } ?>
The above are some basic operations for developing WeChat applets and public accounts using PHP language. I hope it can be helpful to everyone. Of course, there is still a lot involved in WeChat development, which requires further in-depth study and practice. I wish everyone will go further and further on the road of WeChat development.
The above is the detailed content of PHP study notes: WeChat applet and public account development. For more information, please follow other related articles on the PHP Chinese website!
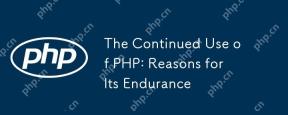
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
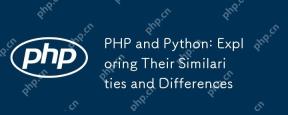
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
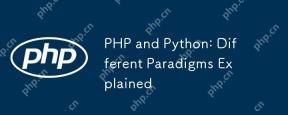
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
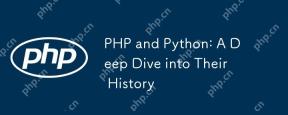
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
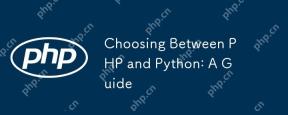
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
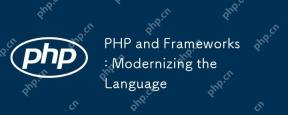
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
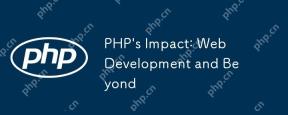
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
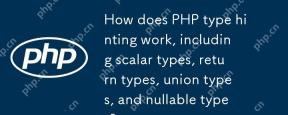
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
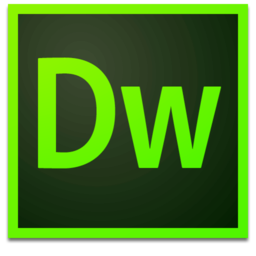
Dreamweaver Mac version
Visual web development tools
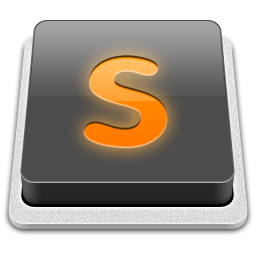
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
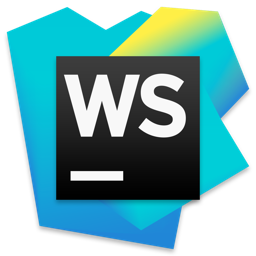
WebStorm Mac version
Useful JavaScript development tools