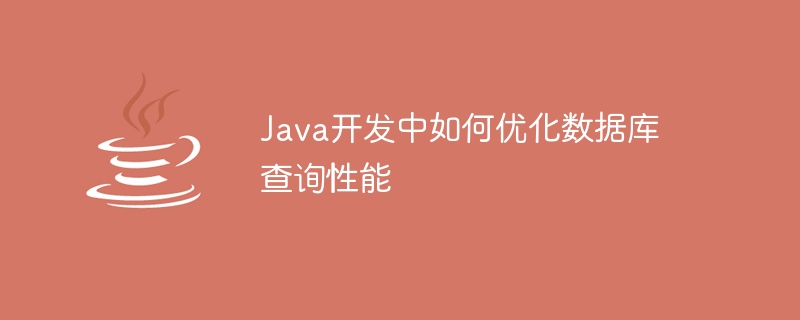
How to optimize database query performance in Java development
Introduction:
In Java development projects, database query is an important and frequent operation. An efficient database query can significantly improve system performance and response speed. This article will discuss how to optimize database query performance from different perspectives, as well as specific code examples.
- Choose appropriate indexes:
Indexes are the key to improving query performance. When designing the database table structure, we should reasonably select and create indexes based on actual needs. Decide whether to create an index based on the frequency of queries and the selectivity of the fields. Too many indexes will increase the load on write operations and slow down query performance. Therefore, we should weigh the number of indexes against the query requirements.
Sample code:
CREATE INDEX idx_username ON users(username);
- Use appropriate data types:
During the database table design process, choosing the appropriate data type can lead to higher query performance performance. For example, for fields that store dates and times, choose an appropriate datetime type and avoid using string types. Using the correct data type can save storage space and improve query efficiency.
Sample code:
ALTER TABLE orders MODIFY COLUMN order_date DATE;
- Batch operations and precompiled statements:
Through batch operations and precompiled statements, the number of communications with the database can be reduced, thereby improving Query performance. Batch operations submit a batch of data to the database at once, while precompiled statements allow query statements to be compiled when the application starts, reducing the overhead of each query.
Sample code:
String sql = "INSERT INTO employees (id, name) VALUES (?, ?)";
PreparedStatement pstmt = connection.prepareStatement(sql);
for(Employee employee : employees) {
pstmt.setInt(1, employee.getId());
pstmt.setString(2, employee.getName());
pstmt.addBatch();
}
pstmt.executeBatch();
- Use paging query:
For queries with large result sets, we should use paging queries to avoid returning too much data at one time . Through the limit and offset statements, you can specify the number of records displayed on each page and the starting position. This reduces data transfer and application memory consumption.
Sample code:
SELECT * FROM orders ORDER BY order_id LIMIT 10 OFFSET 20;
- Avoid using SELECT *:
When querying data, you should only select the required fields and avoid using SELECT . Because the SELECT query will return all fields, even if some fields are not required in the query. Selecting only the fields you need can reduce the amount of data transfer, thereby improving query performance.
Sample code:
SELECT order_id, order_date FROM orders WHERE customer_id = 100;
- Cache query results:
If the results of query data are relatively stable, we can consider caching the query results in memory, Avoid hitting the database for every query. Using cache can significantly improve query performance, especially when queries are frequent and data changes rarely.
Sample code:
Cache cache = new Cache();
ResultSet resultSet = cache.get("SELECT * FROM products WHERE category = 'electronics'");
if(resultSet == null) {
resultSet = executeQuery("SELECT * FROM products WHERE category = 'electronics'");
cache.put("SELECT * FROM products WHERE category = 'electronics'", resultSet);
}
Summary:
Optimizing database query performance is a complex and critical task. This article introduces some common optimization methods in Java development, including choosing appropriate indexes, using appropriate data types, batch operations and prepared statements, using paginated queries, avoiding SELECT *, and caching query results. By implementing these optimization methods, we can significantly improve the performance and responsiveness of the system.
Reference:
- "How to Optimize Database Queries" - https://www.codeofaninja.com/2013/07/optimize-mysql-queries-for-fast- websites.html
- "10 Tips to Improve Your Database Design" - https://www.simple-talk.com/sql/database-administration/ten-common-database-design-mistakes/
Word count: 740
The above is the detailed content of How to optimize database query performance in Java development. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn