


Common code duplication and redundancy problems and solutions in Java development
Code duplication and redundancy are one of the common problems in Java development. When we write code, we often encounter similar or even identical code blocks. Such code duplication will lead to a decrease in the readability and maintainability of the code, and increase the redundancy and complexity of the code. It also affects development efficiency. This article will start by analyzing the causes of code duplication and redundancy problems, provide some solutions, and give specific code examples.
1. Analysis of the causes of code duplication and redundancy issues
1.1 Lack of good code design and architecture
Duplication and redundancy of code are often due to the code design of the project and the structure is not good enough. If code reusability and maintainability are not considered in the early stages of code design, it can easily lead to similar functions being implemented repeatedly, or the same functions appearing in different locations.
1.2 Lack of unified code specifications
The lack of unified code specifications among team members is also a cause of code duplication and redundancy problems. Different people may have different ways of implementing the same problem, and there may also be multiple ways of writing code for the same function.
1.3 Lack of understanding and use of public modules and tool classes
Sometimes we may not fully understand or make full use of existing public modules and tool classes, but choose to re-implement them ourselves Again. This not only wastes time and resources, but also increases code redundancy.
2. Solution
2.1 Improve the design and architecture level of the code
Excellent code design and architecture are important means to solve the problem of code duplication and redundancy. When designing code, you should fully consider the reusability and maintainability of the code, follow the object-oriented design principles, and try to reduce the coupling of the code as much as possible. Through reasonable abstraction, encapsulation and organization of code, code with the same function can be avoided from being implemented repeatedly.
2.2 Develop unified code specifications
Developing unified code specifications is one of the effective ways to solve the problem of code duplication and redundancy. Through unified code specifications, team members can have greater consistency when writing code and reduce unnecessary duplication. Code specifications should include naming rules, code comments, code formats, etc., and at the same time, full consideration should be given to the readability and maintainability of the code.
2.3 Improve the understanding and use of public modules and tool classes
Improving the understanding and use of public modules and tool classes is another aspect of solving the problem of code duplication and redundancy. During the development process, we must make good use of existing public modules and tool classes to reduce code redundancy. Commonly used functions and methods can be encapsulated into tool classes for easy reuse.
3. Specific code examples
The following are some specific code examples to demonstrate how to solve code duplication and redundancy problems.
3.1 Use inheritance or interfaces to achieve code reuse
In object-oriented programming, we can achieve code reuse through inheritance or interfaces. The following is a simple example to achieve code reuse through inheritance:
public abstract class Animal { public abstract void eat(); public abstract void sleep(); } public class Dog extends Animal { public void eat() { System.out.println("狗吃骨头"); } public void sleep() { System.out.println("狗睡觉"); } } public class Cat extends Animal { public void eat() { System.out.println("猫吃鱼"); } public void sleep() { System.out.println("猫睡觉"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog(); dog.eat(); dog.sleep(); Animal cat = new Cat(); cat.eat(); cat.sleep(); } }
defines the common characteristics of animals through the abstract class Animal, and then implements the functions of each specific animal through inheritance.
3.2 Use tool classes to implement code reuse
The following is an example of using tool classes to implement code reuse:
public class StringUtil { public static boolean isEmpty(String str) { return str == null || str.length() == 0; } } public class Main { public static void main(String[] args) { String str = "Hello World"; System.out.println(StringUtil.isEmpty(str)); } }
The isEmpty method in the StringUtil tool class can be used To determine whether a string is empty, you can reuse this function by directly calling this method.
To sum up, code duplication and redundancy are common problems in Java development. These problems can be effectively solved by improving the design and architecture level of the code, formulating unified code specifications, and improving the understanding and use of public modules and tool classes. With concrete code examples, we can better understand and apply these solutions. I hope this article will be helpful to Java developers.
The above is the detailed content of Common code duplication and redundancy problems and solutions in Java development. For more information, please follow other related articles on the PHP Chinese website!
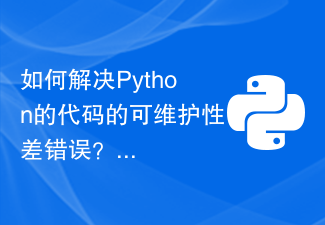
Python作为一门高级编程语言,在软件开发中得到了广泛应用。虽然Python有许多优点,但很多Python程序员经常面临的问题是,代码的可维护性较差。Python代码的可维护性包括代码的易读性、可扩展性、可重用性等方面。在本篇文章中,我们将着重讨论如何解决Python代码的可维护性差的问题。一、代码的易读性代码可读性是指代码的易读程度,它是代码可维护性的核
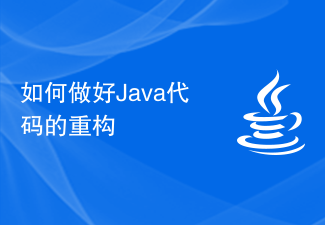
作为世界上最流行的编程语言之一,Java已成为许多企业和开发者的首选语言。然而,代码的重构对于保持代码质量以及开发效率至关重要。Java代码由于其复杂性,随着时间的推移可能会变得越来越难以维护。本文将讨论如何进行Java代码的重构,以提高代码质量和可维护性。了解重构的原则Java代码重构的目的在于改进代码的结构、可读性和可维护性,而不是简单的“改变代码”。因
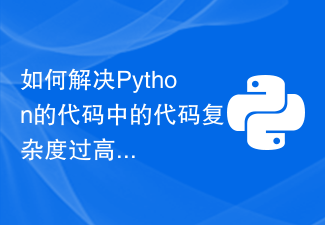
Python是一门简单易学高效的编程语言,但是当我们在编写Python代码时,可能会遇到一些代码复杂度过高的问题。这些问题如果不解决,会使得代码难以维护,容易出错,降低代码的可读性和可扩展性。因此,在本文中,我们将讨论如何解决Python代码中的代码复杂度过高错误。了解代码复杂度代码复杂度是一种度量代码难以理解和维护的性质。在Python中,有一些指标可以用
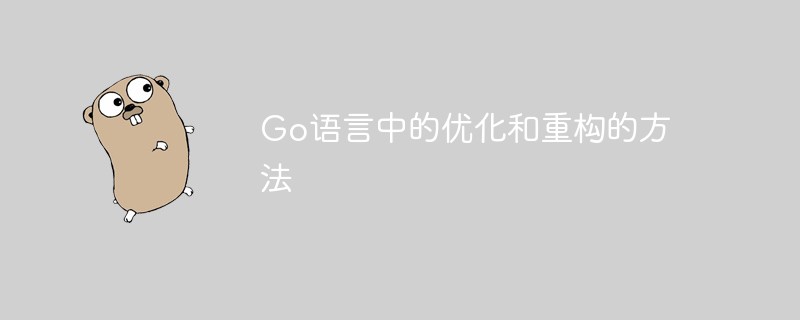
Go语言是一门相对年轻的编程语言,虽然从语言本身的设计来看,其已经考虑到了很多优化点,使得其具备高效的性能和良好的可维护性,但是这并不代表着我们在开发Go应用时不需要优化和重构,特别是在长期的代码积累过程中,原来的代码架构可能已经开始失去优势,需要通过优化和重构来提高系统的性能和可维护性。本文将分享一些在Go语言中优化和重构的方法,希望能够对Go开发者有所帮
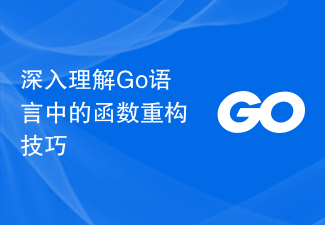
在Go语言程序开发中,函数重构技巧是十分重要的一环。通过优化和重构函数,不仅可以提高代码质量和可维护性,还可以提升程序的性能和可读性。本文将深入探讨Go语言中的函数重构技巧,结合具体的代码示例,帮助读者更好地理解和应用这些技巧。1.代码示例1:提取重复代码片段在实际开发中,经常会遇到重复使用的代码片段,这时就可以考虑将重复代码提取出来作为一个独立的函数,以
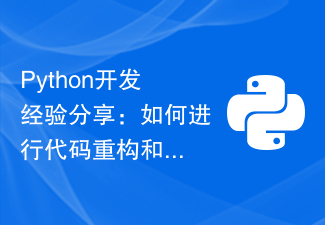
Python开发经验分享:如何进行代码重构和优化引言:随着软件开发的不断发展,代码的重构和优化已成为开发过程中不可或缺的一环。而Python作为一门动态、简洁的高级编程语言,也同样需要进行代码重构和优化来提高程序的性能和可维护性。本文将分享一些Python代码重构和优化的经验,帮助开发者写出更高效、更可靠的Python代码。第一部分:代码重构代码重构是指对已
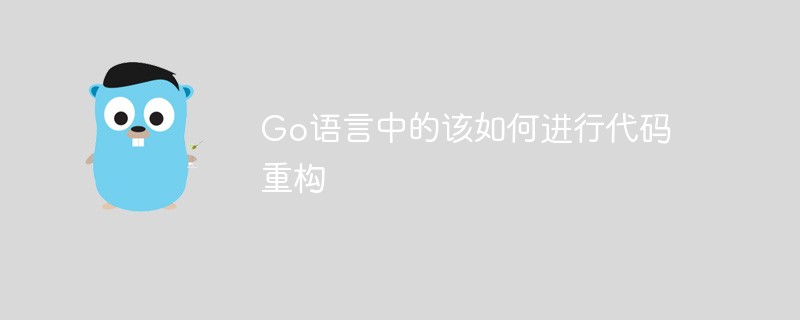
随着软件开发的不断深入和代码的不断积累,代码重构已经成为了现代软件开发过程中不可避免的一部分。它是一种对系统的既定代码进行修改,以改善其结构、性能、可读性或其他相关方面的过程。在本文中,我们将探讨如何在Go语言中进行代码重构。定义好重构的目标在开始代码重构之前,我们应该制定一个清晰的重构目标。我们需要问自己一些问题,比如这段代码存在哪些问题?我们要通过重构
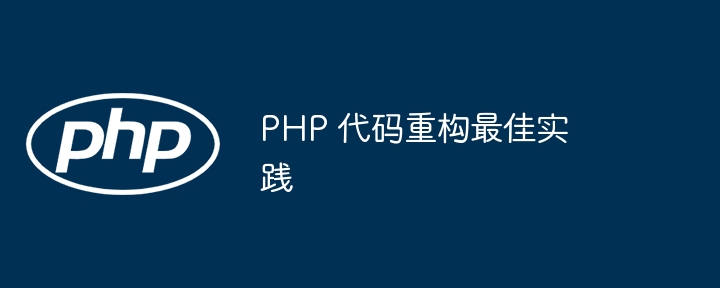
答案:PHP代码重构遵循提高解耦性、可读性、可维护性、减少复杂性的原则。实践:使用命名空间组织代码。用依赖注入容器解耦组件。重构冗余代码。分解大型类。使用现代代码风格。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
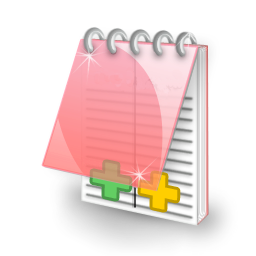
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
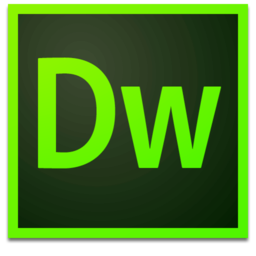
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
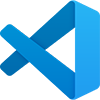
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
