Form validation and submission problems encountered in using Vue development
Vue is a popular JavaScript framework for building user interfaces. One of its main features is its ability to easily handle form validation and submission. However, during development we may encounter some issues related to form validation and submission. This article describes some common problems and provides specific code examples to resolve them.
Question 1: How to use Vue for form validation?
Vue provides an easy way to handle form validation, mainly by using v-model and custom validation rules. Here is an example:
<template> <div> <input v-model="name" placeholder="请输入姓名" /> <span v-if="!nameValid">姓名不能为空</span> <input v-model="email" placeholder="请输入邮箱" /> <span v-if="!emailValid">请输入有效的邮箱</span> <button @click="submitForm">提交</button> </div> </template> <script> export default { data() { return { name: '', email: '' } }, computed: { nameValid() { return this.name !== ''; }, emailValid() { // 正则表达式验证邮箱格式 const regex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,4}$/; return regex.test(this.email); } }, methods: { submitForm() { if (this.nameValid && this.emailValid) { // 提交表单的逻辑 } } } } </script>
In the above code, we use v-model to bind the input value to the attribute in data. Then, verify whether the input is legal through computed properties. Finally, we check all validation rules in the submit button's click event and submit the form only if all rules are met.
Question 2: How to deal with asynchronous issues when submitting forms?
When we handle form submission, sometimes we need to wait for the asynchronous operation to complete before proceeding to the next step. This problem can be solved by using async/await or Promise. Here is an example:
<template> <div> <input v-model="name" placeholder="请输入姓名" /> <button @click="submitForm">提交</button> </div> </template> <script> export default { data() { return { name: '' } }, methods: { async submitForm() { if (this.name === '') { return; } // 模拟异步操作 await new Promise(resolve => setTimeout(resolve, 1000)); // 异步操作完成后的逻辑 // 提交表单的逻辑 } } } </script>
In the above code, we use the async/await keyword to wait for the asynchronous operation to complete. In this example, we use a simulated asynchronous operation to simulate an actual asynchronous request by delaying it for 1 second. After the asynchronous operation is completed, we can continue to perform other operations, such as the logic of submitting the form.
Summary:
In developing with Vue, form validation and submission is a common requirement. We can implement form validation by using v-model and custom validation rules, while using async/await or Promise to handle asynchronous issues. By using these technologies appropriately, we can better handle form validation and submission, improve user experience, and ensure data validity.
The above is the detailed content of Form validation and submission problems encountered in using Vue development. For more information, please follow other related articles on the PHP Chinese website!

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
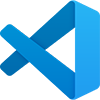
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
