How to share and transfer data in Vue components
How to realize data sharing and transfer in Vue components
In Vue, components are the core part of building web applications. Within components, the sharing and transfer of data is very important. This article will introduce in detail how to share and transfer data in Vue components, and provide specific code examples.
Data sharing refers to the sharing of the same data between multiple components. Data sharing can be achieved even if these components are located in different hierarchies. The transfer of data refers to passing data from one component to another component, usually through the transfer between parent and child components.
- Data Sharing
1.1 Using Vuex
Vuex is a state management mode provided by Vue, which can easily enable multiple components to share the same copies of data. The following is a simple example of using Vuex to achieve data sharing:
// store.js import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) export default new Vuex.Store({ state: { count: 0 }, mutations: { increment(state) { state.count++ } } }) // App.vue <template> <div> <h1 id="count">{{ count }}</h1> <button @click="increment">Increment</button> </div> </template> <script> export default { computed: { count() { return this.$store.state.count } }, methods: { increment() { this.$store.commit('increment') } } } </script>
In the above example, we share data by importing Vuex and creating a store object. In the App.vue component, obtain the shared count data through the computed attribute, and call the increment method through the methods attribute to update the data. It should be noted that in each component that needs to share data, you need to import Vuex and use the corresponding state.
1.2 Using provide and inject
In addition to using Vuex, Vue also provides a simpler way to share data, using provide and inject. The following is an example of using provide and inject to achieve data sharing:
// App.vue <template> <div> <h1 id="count">{{ count }}</h1> <button @click="increment">Increment</button> </div> </template> <script> export default { data() { return { count: 0 } }, provide() { return { count: this.count, increment: this.increment } }, methods: { increment() { this.count++ } } } </script> // Child.vue <template> <div> <h2 id="count">{{ count }}</h2> <button @click="increment">Increment in Child</button> </div> </template> <script> export default { inject: ['count', 'increment'] } </script>
In the above example, we use provide in the parent component App.vue to share the count data and increment method. Use inject in the child component Child.vue to inject the data and methods provided by the parent component. In this way, the data of the parent component can be directly accessed and updated in the child component.
- Transmission of data
2.1 Using props
In Vue, data can be passed from the parent component to the child component through the props attribute. The following is an example of using props to pass data:
// Parent.vue <template> <div> <h1 id="message">{{ message }}</h1> <Child :message="message" /> </div> </template> <script> import Child from './Child.vue' export default { data() { return { message: 'Hello Vue!' } }, components: { Child } } </script> // Child.vue <template> <div> <h2 id="message">{{ message }}</h2> </div> </template> <script> export default { props: ['message'] } </script>
In the above example, we pass the message data in the parent component Parent.vue to the child component Child.vue through the props attribute, and in the child component through the props attribute to receive data passed by the parent component.
- Summary
Through the above sample code, we have learned how to share and transfer data in Vue components. You can choose a suitable solution based on specific business needs to realize data sharing and transfer, and improve the development efficiency and maintainability of Web applications.
The above is the detailed content of How to share and transfer data in Vue components. For more information, please follow other related articles on the PHP Chinese website!

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
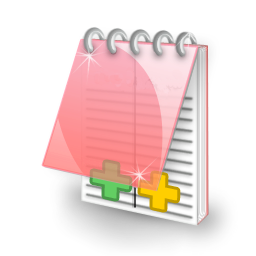
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
