How to perform full-text retrieval and search in Java
Full-text retrieval and search is a technique for finding specific keywords or phrases in large-scale text data. In applications that process large amounts of text data, such as search engines, email systems, and document management systems, full-text retrieval and search functions are very important.
As a widely used programming language, Java provides a wealth of libraries and tools that can help us implement full-text retrieval and search functions. This article will introduce how to use the Lucene library to implement full-text retrieval and search, and provide some specific code examples.
1. Introduce the Lucene library
First, we need to introduce the Lucene library into the project. The Lucene library can be introduced into the Maven project in the following ways:
<dependencies> <dependency> <groupId>org.apache.lucene</groupId> <artifactId>lucene-core</artifactId> <version>8.10.1</version> </dependency> <dependency> <groupId>org.apache.lucene</groupId> <artifactId>lucene-analyzers-common</artifactId> <version>8.10.1</version> </dependency> </dependencies>
2. Create an index
Before performing full-text search, we need to create an index first. This index contains relevant information about the text data to be searched, so that we can perform subsequent search operations. The following is a simple example code for creating an index:
import org.apache.lucene.analysis.Analyzer; import org.apache.lucene.analysis.standard.StandardAnalyzer; import org.apache.lucene.document.Document; import org.apache.lucene.document.Field; import org.apache.lucene.index.IndexWriter; import org.apache.lucene.index.IndexWriterConfig; import org.apache.lucene.store.Directory; import org.apache.lucene.store.FSDirectory; import java.io.IOException; import java.nio.file.Paths; public class Indexer { private IndexWriter indexWriter; public Indexer(String indexDir) throws IOException { Directory dir = FSDirectory.open(Paths.get(indexDir)); Analyzer analyzer = new StandardAnalyzer(); IndexWriterConfig config = new IndexWriterConfig(analyzer); indexWriter = new IndexWriter(dir, config); } public void close() throws IOException { indexWriter.close(); } public void addDocument(String content) throws IOException { Document doc = new Document(); doc.add(new TextField("content", content, Field.Store.YES)); indexWriter.addDocument(doc); } }
In the above example code, we use IndexWriter
to create the index and TextField
to define the Indexed fields. When adding content to be indexed to the index, we need to first create a Document
object, then add fields to the object, and finally call the addDocument
method to add Document
Object is added to the index.
3. Perform search
After creating the index, we can perform search operations. The following is a simple search sample code:
import org.apache.lucene.analysis.Analyzer; import org.apache.lucene.analysis.standard.StandardAnalyzer; import org.apache.lucene.document.Document; import org.apache.lucene.index.DirectoryReader; import org.apache.lucene.index.IndexReader; import org.apache.lucene.queryparser.classic.QueryParser; import org.apache.lucene.search.IndexSearcher; import org.apache.lucene.search.Query; import org.apache.lucene.search.ScoreDoc; import org.apache.lucene.search.TopDocs; import org.apache.lucene.store.Directory; import org.apache.lucene.store.FSDirectory; import java.io.IOException; import java.nio.file.Paths; public class Searcher { private IndexSearcher indexSearcher; private QueryParser queryParser; public Searcher(String indexDir) throws IOException { Directory dir = FSDirectory.open(Paths.get(indexDir)); Analyzer analyzer = new StandardAnalyzer(); IndexReader indexReader = DirectoryReader.open(dir); indexSearcher = new IndexSearcher(indexReader); queryParser = new QueryParser("content", analyzer); } public ScoreDoc[] search(String queryString, int numResults) throws Exception { Query query = queryParser.parse(queryString); TopDocs topDocs = indexSearcher.search(query, numResults); return topDocs.scoreDocs; } public Document getDocument(int docID) throws IOException { return indexSearcher.doc(docID); } }
In the above sample code, we use IndexSearcher
to perform the search operation. Before performing a search, we need to create a Query
object to represent the query to be searched, and use QueryParser
to parse the query string into a Query
object. We then use the search
method of IndexSearcher
to perform the search and return the ranking of the search results.
4. Usage example
The following is a sample code that uses the full-text retrieval and search function:
public class Main { public static void main(String[] args) { String indexDir = "/path/to/index/dir"; try { Indexer indexer = new Indexer(indexDir); indexer.addDocument("Hello, world!"); indexer.addDocument("Java is a programming language."); indexer.addDocument("Lucene is a full-text search engine."); indexer.close(); Searcher searcher = new Searcher(indexDir); ScoreDoc[] results = searcher.search("Java", 10); for (ScoreDoc result : results) { Document doc = searcher.getDocument(result.doc); System.out.println(doc.getField("content").stringValue()); } } catch (IOException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } }
In the above sample code, we first create a Indexer
to create an index and add some text data. Then, we create a Searcher
to perform the search and print out the text content of the search results.
Through the above sample code, we can use the Lucene library to easily implement full-text retrieval and search functions in Java. Using Lucene, we can efficiently find specific keywords or phrases in large-scale text data, thereby improving the efficiency and performance of text processing applications.
The above is the detailed content of How to perform full text retrieval and search in Java. For more information, please follow other related articles on the PHP Chinese website!
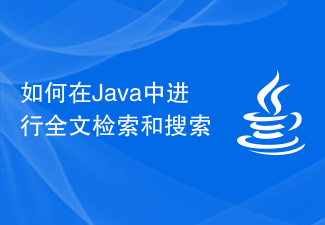
如何在Java中进行全文检索和搜索全文检索和搜索是在大规模文本数据中查找特定关键字或短语的一种技术。在处理大量文本数据的应用中,如搜索引擎、电子邮件系统和文档管理系统中,全文检索和搜索功能都是非常重要的。Java作为一种广泛使用的编程语言,提供了丰富的库和工具,可以帮助我们实现全文检索和搜索功能。本文将介绍如何利用Lucene库来实现全文检索和搜索,并提供一
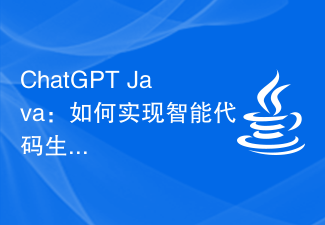
ChatGPTJava:如何实现智能代码生成与优化引言:随着人工智能技术的快速发展,智能代码生成和优化成为了编程领域的热门话题。ChatGPT是一种基于OpenAI的强大语言模型,可以实现自然语言与机器之间的交互。本文将介绍如何使用ChatGPT来实现智能代码生成与优化的操作,以及提供一些具体的代码示例。一、智能代码生成:使用ChatGPT构建智能代码生成
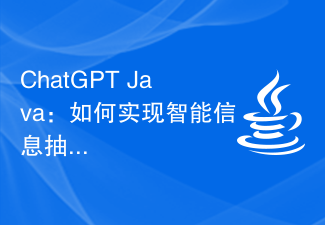
ChatGPTJava:如何实现智能信息抽取和结构化处理,需要具体代码示例引言:随着人工智能技术的快速发展,智能信息抽取和结构化处理在数据处理领域中扮演着越来越重要的角色。在本文中,我们将介绍如何使用ChatGPTJava来实现智能信息抽取和结构化处理的功能,并提供具体的代码示例。一、智能信息抽取智能信息抽取是指从非结构化数据中提取关键信息的过程。在Ja
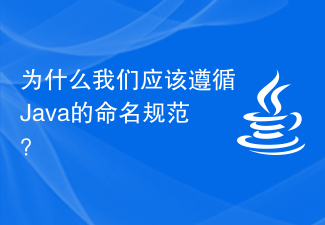
Java命名约定通过使程序更易于阅读,使其更易于理解。在Java中,类名通常应该是名词,以大写字母开头的标题形式,每个单词的首字母大写。接口名通常应该是形容词,以大写字母开头的标题形式,每个单词的首字母大写。为什么应该遵循Java命名标准减少阅读和理解源代码所需的工作量。使代码审查能够专注于比语法和命名标准更重要的问题。使代码质量审查工具能够主要关注重要问题而不是语法和风格偏好。不同类型标识符的命名约定包包名应全部小写。示例packagecom.tutorialspoint;接口接口名称应以大写
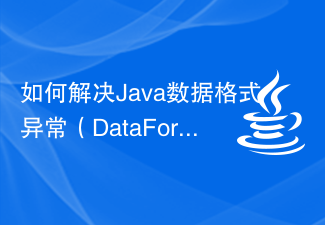
如何解决Java数据格式异常(DataFormatException)在Java编程中,我们经常会遇到各种异常情况。其中,数据格式异常(DataFormatException)是一个常见但也很具挑战性的问题。当输入的数据无法满足指定的格式要求时,就会抛出这个异常。解决这个异常需要一定的技巧和经验。本文将详细介绍如何解决Java数据格式异常,并提供一些代码示例
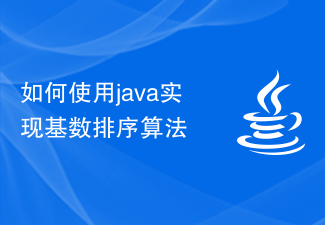
如何使用Java实现基数排序算法?基数排序算法是一种非比较排序算法,它基于元素的位值进行排序。它的核心思想是将待排序的数字按照个位、十位、百位等位数进行分组,然后依次对各位进行排序,最终得到有序的序列。下面将详细介绍如何使用Java实现基数排序算法,并提供代码示例。首先,基数排序算法需要准备一个二维数组来保存待排序的数字。数组的行数由位数决定,例如待
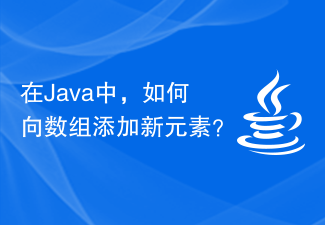
Java中向数组中添加新元素是一种常见的操作,可以使用多种方法实现。本文将介绍几种常见的添加元素到数组的方法,并提供相应的代码示例。一、使用新数组一种常见的方法是创建一个新的数组,将原数组的元素复制到新数组中,并在新数组的末尾添加新元素。具体步骤如下:创建一个新的数组,大小比原数组大1。这是因为要添加一个新元素。将原数组的元素复制到新数组中。在新数组的末尾添
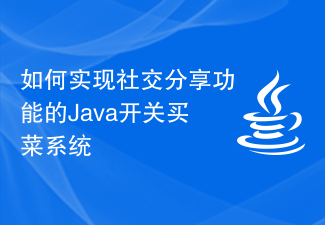
如何实现社交分享功能的Java开关买菜系统随着科技的发展和社交媒体的普及,越来越多的人习惯在购物时分享自己的购买体验和心得。为了满足用户的需求,一个好的购物系统不仅需要方便快捷地完成购买,还需要提供社交分享功能。本文将介绍如何实现社交分享功能的Java开关买菜系统。首先,我们需要确定要实现的社交分享渠道,常见的有微信、微博、QQ等。在Java中,可以使用第三


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
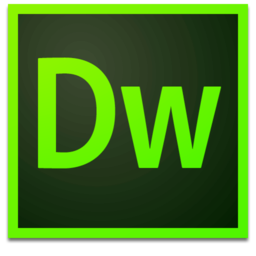
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
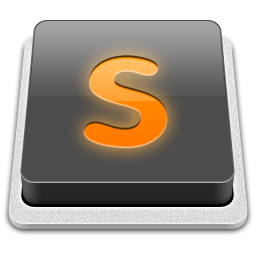
SublimeText3 Mac version
God-level code editing software (SublimeText3)
