Concurrent programming skills: Advanced usage of Go WaitGroup
Concurrent programming skills: Advanced usage of Go WaitGroup
In concurrent programming, coordinating and managing the execution of multiple concurrent tasks is an important task. The Go language provides a very practical concurrency primitive - WaitGroup, which can help us implement concurrency control elegantly. This article will introduce the basic usage of WaitGroup, and focus on its advanced usage, using specific code examples to help readers better understand and apply it.
WaitGroup is a concurrency primitive built into the Go language, which can help us wait for the completion of concurrent tasks. It provides three methods: Add, Done and Wait. The Add method is used to set the number of waiting tasks, the Done method is used to reduce the number of waiting tasks, and the Wait method is used to block the current coroutine until all waiting tasks are completed.
The following is a simple example showing the basic usage of WaitGroup:
package main import ( "fmt" "sync" "time" ) func main() { var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func(num int) { defer wg.Done() time.Sleep(time.Second) fmt.Println("Task", num, "done") }(i) } wg.Wait() fmt.Println("All tasks done") }
In the above code, we create a WaitGroup object wg and create 5 concurrent tasks through a loop . During the execution of each task, we use the Add method to increase the number of waiting tasks, and at the end of the task, we use the Done method to reduce the number of waiting tasks. Finally, we call the Wait method to block the main coroutine until all waiting tasks are completed.
In addition to basic usage, WaitGroup also provides some advanced usage, which can control the execution of concurrent tasks more flexibly. Below we will introduce several commonly used advanced usages in detail.
- Execute a set of tasks and set the maximum number of concurrencies
If we need to execute a set of tasks at the same time but want to limit the maximum number of concurrencies, we can use buffered channel combination WaitGroup to achieve. The code below shows how to execute a set of tasks at the same time, but only allows up to 3 tasks to execute concurrently:
package main import ( "fmt" "sync" "time" ) func main() { var wg sync.WaitGroup maxConcurrency := 3 tasks := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} sem := make(chan struct{}, maxConcurrency) for _, task := range tasks { wg.Add(1) sem <- struct{}{} // 获取令牌,控制最大并发数 go func(num int) { defer wg.Done() time.Sleep(time.Second) fmt.Println("Task", num, "done") <-sem // 释放令牌,允许新的任务执行 }(task) } wg.Wait() fmt.Println("All tasks done") }
In the above code, we create a buffered channel sem and set its size is the maximum number of concurrencies. Before each task starts, we obtain a token through the sem
- Timeout controls the execution of concurrent tasks
Sometimes we want to control the execution time of concurrent tasks and terminate the execution of the task when it times out. By using buffered channels and timers, we can easily implement this functionality. The following code shows how to set the timeout of concurrent tasks to 3 seconds:
package main import ( "fmt" "sync" "time" ) func main() { var wg sync.WaitGroup tasks := []int{1, 2, 3, 4, 5, 6, 7} timeout := 3 * time.Second done := make(chan struct{}) for _, task := range tasks { wg.Add(1) go func(num int) { defer wg.Done() // 模拟任务执行时间不定 time.Sleep(time.Duration(num) * time.Second) fmt.Println("Task", num, "done") // 判断任务是否超时 select { case <-done: // 任务在超时前完成,正常退出 return default: // 任务超时,向通道发送信号 close(done) } }(task) } wg.Wait() fmt.Println("All tasks done") }
In the above code, we create a channel done, and determine whether the channel is closed during task execution to determine whether the task is time out. When a task is completed, we use the close(done) statement to send a signal to the done channel to indicate that the task has timed out. Choose different branches through select statements to handle different situations.
Through the above sample code, we can see that the advanced usage of WaitGroup is very practical in actual concurrent programming. Mastering these techniques, we can better control the execution of concurrent tasks and improve the performance and maintainability of the code. I hope readers can gain a deep understanding of the usage of WaitGroup through the introduction and sample code of this article, and then apply it to actual projects.
The above is the detailed content of Concurrent programming skills: Advanced usage of Go WaitGroup. For more information, please follow other related articles on the PHP Chinese website!
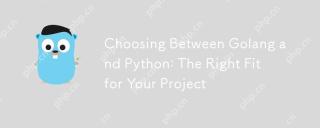
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
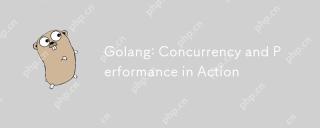
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
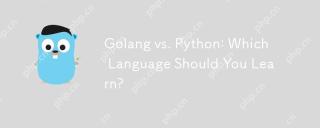
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
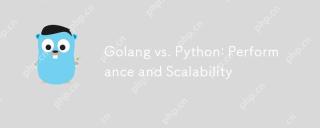
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
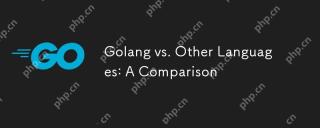
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
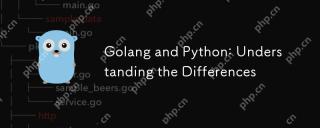
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
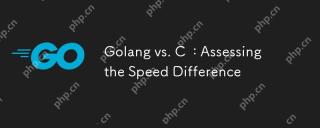
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
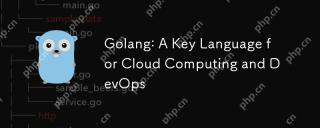
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
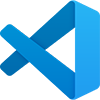
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft