


React cross-domain request solution: how to deal with cross-domain access issues in front-end applications
React cross-domain request solution: How to deal with cross-domain access issues in front-end applications, specific code examples are needed
In front-end development, we often encounter cross-domain requests Requested Questions. Cross-domain request means that the target address (domain name, port, protocol) of the HTTP request sent by the front-end application is inconsistent with the address of the current page. Due to the browser's same-origin policy, cross-domain requests are restricted. However, in real development, we often need to communicate with different servers, so the solution for cross-domain requests is particularly important.
This article will introduce the solution for React cross-domain requests and give specific code examples.
1. JSONP
JSONP is a solution for cross-domain requests. It takes advantage of the fact that the <script></script>
tag has no cross-domain restrictions. The specific implementation steps are as follows:
- In the front-end application, add a
<script></script>
tag and use the server URL as the value of itssrc
attribute. - On the server side, process the request and return a function call, which serves as a callback function and passes data to the front-end application in the form of parameters.
- After the front-end application loads the
<script></script>
tag, it can obtain the data returned from the server.
The following is a sample code:
function jsonp(url, callback) { const script = document.createElement('script'); script.src = url; window[callback] = function(data) { delete window[callback]; document.body.removeChild(script); callback(data); }; document.body.appendChild(script); } function fetchUserData() { jsonp('http://api.example.com/user', 'handleUserData'); } function handleUserData(data) { // 处理从服务端返回的数据 } fetchUserData();
2. CORS
CORS (Cross-Origin Resource Sharing) is a cross-domain request provided by the browser Solution, which implements permission control for cross-domain requests by adding specific fields in HTTP request headers. The following is a sample code for using CORS to make cross-domain requests:
fetch('http://api.example.com/user', { method: 'GET', headers: { 'Content-Type': 'application/json', 'Access-Control-Allow-Origin': '*' // 设置允许跨域的域名 }, }) .then(response => response.json()) .then(data => { // 处理从服务端返回的数据 }) .catch(error => { console.error(error); });
On the server side, you need to set the Access-Control-Allow-Origin
field in the response header to specify the cross-domain access allowed domain name. If cross-domain access is allowed for all domain names, the value of this field can be set to *
.
3. Use a reverse proxy
Another common way to solve the problem of cross-domain requests is to use a reverse proxy. The specific steps are as follows:
- Open a proxy server locally and forward the request of the target server to the proxy server.
- The proxy server then sends the request to the target server and returns the response to the front-end application.
In this way, requests sent by the front-end application can bypass the browser's same-origin policy and implement cross-domain requests.
The following is a sample code using a reverse proxy:
const express = require('express'); const proxy = require('http-proxy-middleware'); const app = express(); app.use('/api', proxy({ target: 'http://api.example.com', changeOrigin: true })); app.listen(3000, () => { console.log('Proxy server is running on port 3000'); });
Through the above code, we forward the request starting with /api
to http:// api.example.com
.
Summary:
This article introduces three solutions for React cross-domain requests: JSONP, CORS and using reverse proxy. In actual development, according to specific application scenarios and requirements, you can choose an appropriate solution to handle cross-domain requests. I hope the content of this article will be helpful in solving React cross-domain request problems.
The above is the detailed content of React cross-domain request solution: how to deal with cross-domain access issues in front-end applications. For more information, please follow other related articles on the PHP Chinese website!
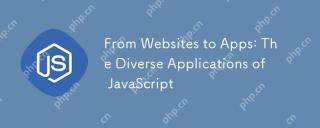
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
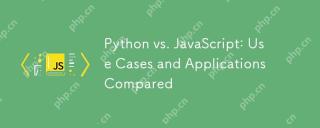
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
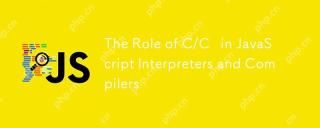
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
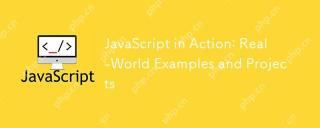
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
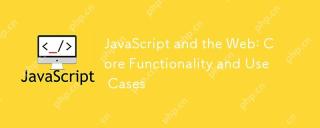
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
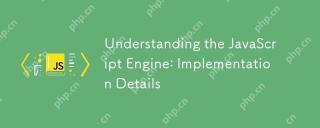
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
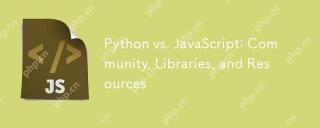
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.