How to implement a simple music player using PHP
How to use PHP to implement a simple music player
Introduction:
Music player is a common web application that can play and manage audio file. In this article, we will learn how to implement a simple music player using PHP language and some open source libraries. Through the study of this article, readers will learn how to process audio files, create a simple player interface, and how to implement audio playback and control.
- Preparation
Before we start, we need to prepare some basic resources.
- PHP environment: Make sure you have PHP installed and can run PHP programs locally.
- Audio files: Prepare some audio files, which can be in MP3 format or other common formats.
- Open source library: We will use an open source library called jPlayer, which is a powerful, flexible and compatible HTML5 audio player. You can download the latest version from the jPlayer official website.
- Create a basic HTML structure
Before using jPlayer, we need to create a basic HTML structure for the player. Here is a simple example:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>音乐播放器</title> </head> <body> <audio id="myAudio" src=""></audio> <button onclick="play()">播放</button> <button onclick="pause()">暂停</button> <button onclick="stop()">停止</button> </body> </html>
In the above example, we have created an audio element for playing audio files. We also added a few buttons to control the player's play, pause, and stop functionality.
- Introducing jPlayer library
Next step, we need to introduce jPlayer library into our project. After downloading or obtaining the code for jPlayer, unzip it and copy the required files into your project directory.
Then, introduce jPlayer’s CSS and JS files into our HTML page. Add the following code to the
tag:<link rel="stylesheet" type="text/css" href="path/to/jplayer.css"> <script type="text/javascript" src="path/to/jplayer.js"></script>
Make sure you have path/to/jplayer.css
and path/to/jplayer.js## set correctly #path of.
- Processing Audio Files
- In the player, we need to handle the uploading, adding and deleting of audio files. Here we will use a simple upload form to upload audio files.
<form action="upload.php" method="POST" enctype="multipart/form-data"> <input type="file" name="file"> <input type="submit" value="上传"> </form>
upload.php script for processing.
upload.php script to handle the uploaded audio files. Here is a simple example:
<?php if(isset($_FILES['file'])){ $file = $_FILES['file']; $targetDir = "uploads/"; $targetFile = $targetDir . basename($file['name']); move_uploaded_file($file['tmp_name'], $targetFile); } ?>In the above example, we first check if the file has been uploaded. We then move the temporary path of the uploaded file to the specified destination path.
- Implement audio playback and control
- Next, we need to use PHP to implement audio file playback and control. Here is a simple example:
<?php $audioFiles = glob("uploads/*.mp3"); if(isset($_GET['file'])){ $selectedFile = $_GET['file']; echo '<audio id="myAudio" src="'.$selectedFile.'"></audio>'; }else{ $selectedFile = $audioFiles[0]; echo '<audio id="myAudio" src="'.$selectedFile.'"></audio>'; } ?> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>音乐播放器</title> <link rel="stylesheet" type="text/css" href="path/to/jplayer.css"> <script type="text/javascript" src="path/to/jplayer.js"></script> <script type="text/javascript"> function play(){ $("#myAudio").jPlayer("play"); } function pause(){ $("#myAudio").jPlayer("pause"); } function stop(){ $("#myAudio").jPlayer("stop"); } </script> </head> <body> <div class="playlist"> <?php foreach($audioFiles as $file): ?> <a href="?file=<?php echo $file ?>"><?php echo basename($file) ?></a><br> <?php endforeach; ?> </div> <button onclick="play()">播放</button> <button onclick="pause()">暂停</button> <button onclick="stop()">停止</button> </body> </html>
So far, we have completed the creation of a simple music player. This player can upload audio files, display playlists, and play, pause, and stop audio files.
The above is the detailed content of How to implement a simple music player using PHP. For more information, please follow other related articles on the PHP Chinese website!
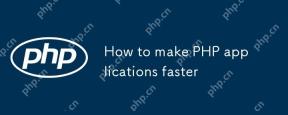
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
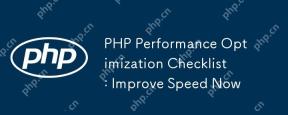
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
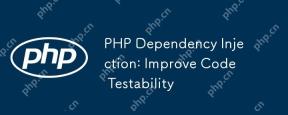
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
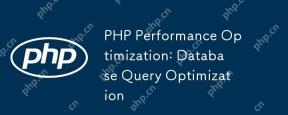
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
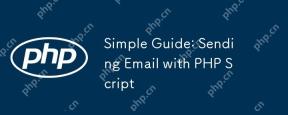
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
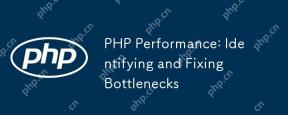
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
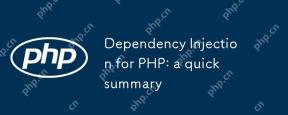
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
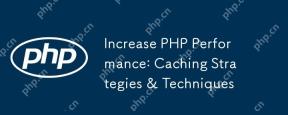
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
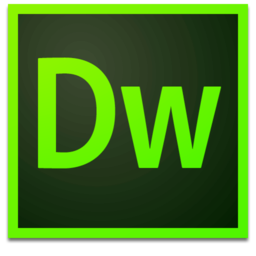
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
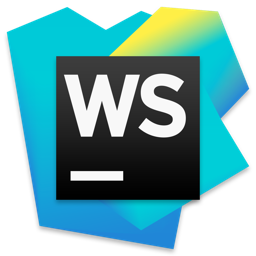
WebStorm Mac version
Useful JavaScript development tools
