


Performance Optimization and Countermeasures of Java Warehouse Management System
Performance Optimization and Countermeasures of Java Warehouse Management System
Abstract: With the rapid development of the e-commerce industry, the load of the warehouse management system is increasing. As a result, the demand for system performance is getting higher and higher. This article will introduce some performance optimization techniques and coping strategies for Java warehouse management systems, and provide specific code examples.
1. Performance optimization skills
- Reasonable use of cache: In the warehouse management system, many data can be cached, such as product information, order information, etc. Reasonable use of cache can reduce database load and improve system response speed. You can use caching technologies such as Redis to store popular data in memory and reduce the frequency of database queries.
Sample code:
// 使用Redis缓存商品信息 public class ProductCache { private RedisTemplate<String, Product> redisTemplate; public Product getProductById(String id) { Product product = redisTemplate.opsForValue().get(id); if (product == null) { // 从数据库中查询商品信息 product = getProductFromDatabase(id); // 存入缓存 redisTemplate.opsForValue().set(id, product); } return product; } private Product getProductFromDatabase(String id) { // 从数据库中查询商品信息的具体实现 } }
- Reduce the number of database queries: Database queries are time-consuming operations. In high concurrency situations, frequent database queries will lead to a decrease in system performance. . Therefore, when designing the data access layer, the number of database queries should be minimized, which can be optimized through caching, batch queries, and the use of indexes.
Sample code:
// 使用批量查询减少数据库查询次数 public class OrderDao { private JdbcTemplate jdbcTemplate; public List<Order> getOrdersByUserId(String userId) { String sql = "SELECT * FROM orders WHERE user_id = ?"; return jdbcTemplate.query(sql, new Object[]{userId}, new OrderRowMapper()); } // OrderRowMapper的具体实现 // ... }
- Multi-threaded concurrent processing: In the warehouse management system, many business operations can be processed concurrently, such as order processing, inventory management, etc. By using multi-threading technology, tasks can be distributed to multiple threads for parallel processing, improving the concurrency and throughput of the system.
Sample code:
// 使用线程池并发处理订单 public class OrderService { private ExecutorService executorService; public void processOrders(List<Order> orders) { for (Order order : orders) { executorService.submit(new OrderProcessor(order)); } } // OrderProcessor的具体实现 // ... }
2. Countermeasures
- Monitor system performance: In the running environment, monitor the performance indicators of the system in real time, including CPU Utilization, memory usage, number of database connections, etc., detect bottlenecks and anomalies in a timely manner, and make corresponding adjustments.
- Asynchronous processing: For some time-consuming operations, you can use asynchronous processing to improve the concurrency capability of the system. For example, the product warehousing operation can be put into the message queue for asynchronous processing, decoupling the long time-consuming operation from the user request.
Sample code:
// 使用消息队列异步处理商品入库操作 public class ProductQueue { private BlockingQueue<Product> queue; public void enqueue(Product product) { queue.offer(product); } public void startConsuming() { Thread consumer = new Thread(() -> { while (true) { try { Product product = queue.take(); // 处理商品入库操作的具体实现 } catch (InterruptedException e) { e.printStackTrace(); } } }); consumer.start(); } }
- Database optimization: For database performance bottlenecks, you can perform some database optimization operations, such as adding indexes, optimizing SQL statements, and adjusting database connection pool parameters. etc. to improve database query performance.
- Vertical expansion and horizontal expansion: If the load on the system is too large, you can consider using vertical expansion or horizontal expansion to improve system performance. Vertical expansion means increasing the hardware resources of a single server, such as CPU, memory, etc.; horizontal expansion means increasing the number of servers and distributing requests to multiple servers through load balancing.
Conclusion: Through reasonable use of cache, reducing the number of database queries, multi-threaded concurrent processing and other performance optimization techniques, as well as strategies to monitor system performance and make corresponding adjustments, the Java warehouse management system can be effectively improved. performance to meet the needs of the e-commerce industry for system response speed and concurrency capabilities.
Note: The above example code is only for demonstration. The actual situation needs to be adjusted and expanded according to the specific business and system architecture.
The above is the detailed content of Performance Optimization and Countermeasures of Java Warehouse Management System. For more information, please follow other related articles on the PHP Chinese website!
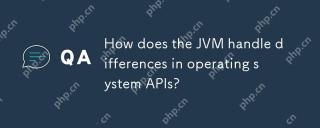
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
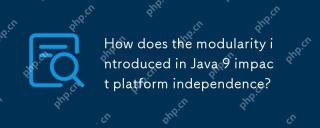
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
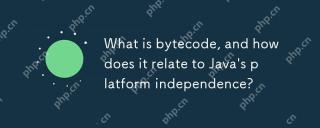
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
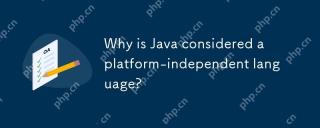
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
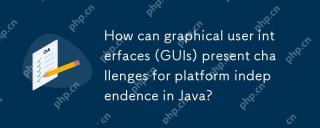
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
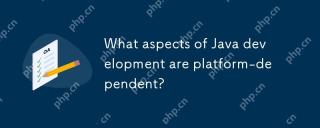
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
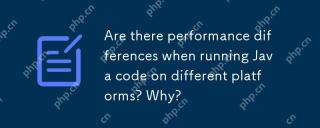
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
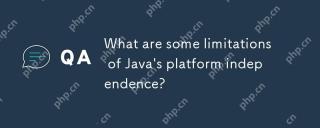
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
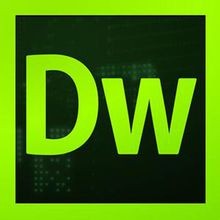
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
