


How to implement the backup and synchronization function of answer records in online answer questions
How to implement the answer record backup and synchronization function in online answering requires specific code examples
With the rapid development of Internet technology, more and more educational institutions and online education platforms began to transform traditional paper test papers into online answering formats. The backup and synchronization functions of answer records are of great significance to teachers and students. This article will introduce how to implement the answer record backup and synchronization function in online answering, and provide specific code examples.
1. Backup of answer records
Backup of answer records refers to saving students’ answer records to the server or cloud storage so that they can be restored and viewed at any time. The following are the key steps to implement answer record backup:
- Create database table structure
First, we need to create a MySQL database and create a table named The "answer_records" table is used to store students' answer records. The fields of the table include: student ID, question ID, answers, scores, etc.
CREATE TABLE answer_records ( student_id INT NOT NULL, question_id INT NOT NULL, answer VARCHAR(255), score FLOAT, PRIMARY KEY (student_id, question_id) );
- Writing a data operation class
Next, we need to write a data operation class for inserting, updating and querying answer records into the database. The following is a simple PHP example:
class AnswerRecordDAO { private $conn; // 数据库连接对象 public function __construct() { // 连接数据库 $this->conn = new mysqli("localhost", "username", "password", "database"); if ($this->conn->connect_error) { die("数据库连接失败:" . $this->conn->connect_error); } } public function insertAnswerRecord($studentId, $questionId, $answer, $score) { $stmt = $this->conn->prepare("INSERT INTO answer_records (student_id, question_id, answer, score) VALUES (?, ?, ?, ?)"); $stmt->bind_param("iisd", $studentId, $questionId, $answer, $score); $stmt->execute(); $stmt->close(); } public function updateAnswerRecord($studentId, $questionId, $answer, $score) { $stmt = $this->conn->prepare("UPDATE answer_records SET answer = ?, score = ? WHERE student_id = ? AND question_id = ?"); $stmt->bind_param("sdii", $answer, $score, $studentId, $questionId); $stmt->execute(); $stmt->close(); } public function getAnswerRecord($studentId, $questionId) { $stmt = $this->conn->prepare("SELECT answer, score FROM answer_records WHERE student_id = ? AND question_id = ?"); $stmt->bind_param("ii", $studentId, $questionId); $stmt->execute(); $stmt->bind_result($answer, $score); $stmt->fetch(); $stmt->close(); $record = array("answer" => $answer, "score" => $score); return $record; } // ...其他方法 }
- Call the data operation class
In the online question answering system, when students submit their answers, we need to call the data operation class The method saves the answer records to the database. The following is a simple PHP example:
$answerRecordDAO = new AnswerRecordDAO(); $answerRecordDAO->insertAnswerRecord($studentId, $questionId, $answer, $score);
So far, we have completed the implementation of answer record backup in online answering. The following will introduce how to implement the synchronization function of answer records.
2. Answer record synchronization
Answer record synchronization means that when students answer questions on different devices, the consistency of the answer records can be ensured. The following are the key steps to achieve synchronization of answer records:
- Use the account system
In order to achieve synchronization of answer records, we need to introduce an account system. When students log in on different devices, use the same account so that answer records can be backed up and synchronized correctly.
- Data synchronization strategy
When students answer questions on different devices, we need to develop a data synchronization strategy. A common strategy is to compare the timestamp with the last update time of the server-side record. If the student's answer record is newer, synchronize the server-side answer record to the student's device.
- Mobile client sample code
The following is a simple Android client sample code for obtaining answer records from the server and saving them locally:
class AnswerRecordSyncTask extends AsyncTask<Void, Void, Void> { @Override protected Void doInBackground(Void... voids) { // 从服务器端获取答题记录 String url = "http://example.com/get_answer_record.php"; HttpClient client = new DefaultHttpClient(); HttpGet httpGet = new HttpGet(url); HttpResponse response; try { response = client.execute(httpGet); HttpEntity entity = response.getEntity(); String jsonString = EntityUtils.toString(entity); // 将答题记录保存到本地 SharedPreferences sharedPreferences = getSharedPreferences("answer_record", MODE_PRIVATE); SharedPreferences.Editor editor = sharedPreferences.edit(); editor.putString("answer", jsonString); editor.apply(); } catch (IOException e) { e.printStackTrace(); } return null; } } // 调用答题记录同步任务 new AnswerRecordSyncTask().execute();
So far, we have completed the implementation of the backup and synchronization function of answer records in online answering.
Summary
This article introduces the implementation of answer record backup and synchronization functions in online answering, and provides specific code examples. By implementing the backup and synchronization functions of answer records, teachers and students can view and restore answer records at any time to improve teaching and learning effects. Of course, the specific implementation method can also be adjusted and expanded according to actual needs. Hope this article helps you!
The above is the detailed content of How to implement the backup and synchronization function of answer records in online answer questions. For more information, please follow other related articles on the PHP Chinese website!
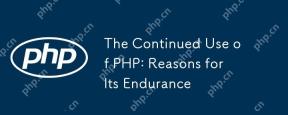
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
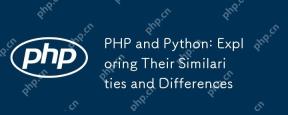
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
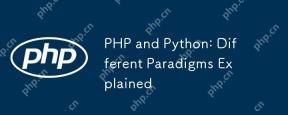
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
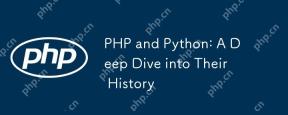
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
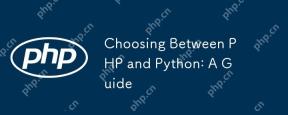
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
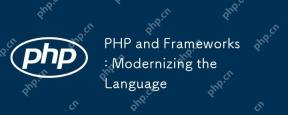
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
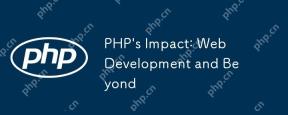
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
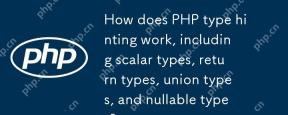
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
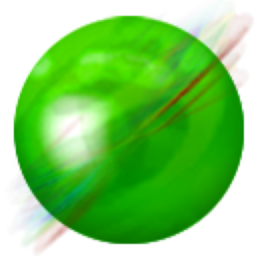
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
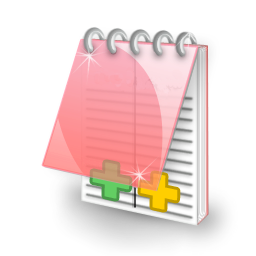
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor