Beginner's Guide to Java Development: From Getting Started to Giving Up
Java is a programming language widely used in software development. Its concise syntax and powerful functions make it the first choice for many developers. However, for beginners, learning Java may feel a little difficult. This article will provide a guide for Java development beginners to help them from getting started to giving up.
- Learn basic syntax
The basic syntax of Java includes variables, data types, operators, conditional statements, loop statements, etc. Beginners should start with these basic concepts and write simple code examples to deepen their understanding. The following is a sample code that calculates the sum of two numbers:
public class HelloWorld { public static void main(String[] args) { int a = 10; int b = 20; int sum = a + b; System.out.println("Sum: " + sum); } }
- Understanding object-oriented programming
Java is an object-oriented programming language. Beginners should learn classes and objects. , inheritance, encapsulation and other concepts, and write corresponding code examples to practice. The following is a simple sample code for a student class:
public class Student { private String name; private int age; public Student(String name, int age) { this.name = name; this.age = age; } public void introduce() { System.out.println("Name: " + name); System.out.println("Age: " + age); } public static void main(String[] args) { Student student = new Student("John", 20); student.introduce(); } }
- Master common APIs
Java provides many commonly used APIs, such as string processing, file operations, collections, etc. Beginners should have a deep understanding of these APIs and write relevant code examples to deepen their memory and understanding. The following is a sample code using a collection:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); for (String name : names) { System.out.println(name); } } }
- Learn debugging skills
During the development process, debugging is a very important skill. Beginners should learn to use debugging tools to find errors in their code and fix them. Here is a sample code that uses debugging skills to find errors:
public class DebugExample { public static void main(String[] args) { int sum = 0; for (int i = 1; i <= 10; i++) { sum += i; } System.out.println("Sum: " + sum); } }
- Learn common programming practices
Good programming practices are about writing clear and readable code The essential. Beginners should learn and abide by common programming conventions, such as naming conventions, indentation conventions, comment conventions, etc. The following is a sample code that complies with programming standards:
public class CodeExample { private static final int MAX_COUNT = 100; public static void main(String[] args) { int sum = 0; for (int i = 1; i <= MAX_COUNT; i++) { sum += i; } System.out.println("Sum: " + sum); } }
Through the above-mentioned aspects, beginners can gradually master the basic knowledge and skills of Java programming. Of course, learning programming is not an overnight process and requires continuous practice and accumulation of experience. I hope this article can provide some guidance for beginners and help them from getting started to giving up. However, remember that giving up is not the answer to the problem; perseverance is the key to success.
The above is the detailed content of Beginner's Guide to Java Development: From Getting Started to Giving Up. For more information, please follow other related articles on the PHP Chinese website!
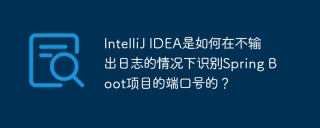
Start Spring using IntelliJIDEAUltimate version...
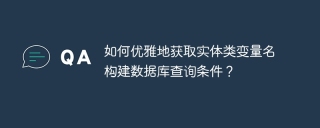
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
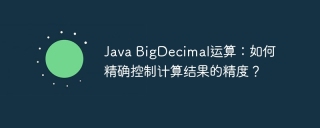
Java...
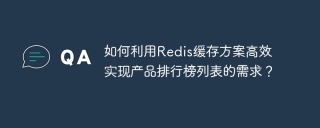
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
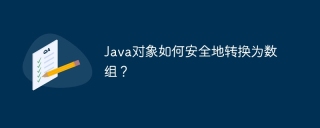
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
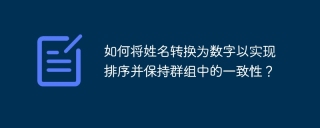
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
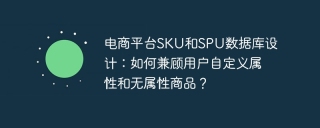
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
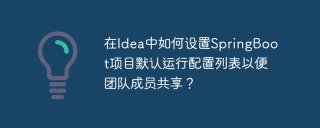
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Notepad++7.3.1
Easy-to-use and free code editor