How to use Redis and Dart to develop cache penetration defense functions
In modern web applications, caching is a common performance optimization technology. However, caching systems may be vulnerable to cache penetration attacks. Cache penetration refers to requesting data that does not exist in the cache. When requests are frequent, it will cause a large number of invalid requests to directly access the database or other back-end services, thus affecting the performance of the system.
In order to solve the problem of cache penetration, we can use Redis and Dart language to develop a cache penetration defense function. The following are the specific implementation steps and sample code:
- Import the Redis library in the Dart project
First, use the pubspec.yaml file to import the redis library in the Dart project, As shown below:
dependencies: redis: ^4.0.0
Then, run the pub get
command to get the required dependencies.
- Connect to the Redis server
Use the following code to connect to the Redis server:
import 'package:redis/redis.dart'; Future<void> main() async { final redis = await RedisConnection.connect('localhost', 6379); }
Please make sure to replace localhost
with # Replace ##6379 with the correct hostname and port number of your Redis server.
- Create cache key
/api/data?id=123, we can use
data_123 as the cache key.
String createCacheKey(String dataType, String id) { return '$dataType_$id'; }
- Implementation of cache penetration defense function
import 'package:redis/redis.dart'; class Cache { final RedisConnection _redis; Cache(this._redis); Future<String?> get(String key) async { final value = await _redis.get(key); if (value == null) { return null; } else if (value.isEmpty) { // 如果值为空字符串,则表示请求结果为空 return ''; } else { return value; } } Future<void> set(String key, String value, {Duration? expiration}) async { await _redis.set(key, value); if (expiration != null) { await _redis.expire(key, expiration.inSeconds); } } } class DataService { final Cache _cache; DataService(this._cache); Future<String> getData(String id) async { final cacheKey = createCacheKey('data', id); final cachedValue = await _cache.get(cacheKey); if (cachedValue != null) { return cachedValue; } // 从后端服务获取数据 final data = await fetchDataFromBackendService(id); // 如果数据不存在,则将空字符串存储到缓存中,避免重复查询 final expiration = data.isNotEmpty ? Duration(minutes: 5) : Duration(seconds: 30); await _cache.set(cacheKey, data, expiration: expiration); return data; } Future<String> fetchDataFromBackendService(String id) async { // 从后端服务获取数据的实现代码 } } Future<void> main() async { final redis = await RedisConnection.connect('localhost', 6379); final cache = Cache(redis); final dataService = DataService(cache); final data = await dataService.getData('123'); print('Data: $data'); }On top In the example, we first encapsulate the interaction with Redis by creating the
Cache class. Then, implement the data acquisition logic by creating the
DataService class. In the
getData method, we first try to get the requested data from the cache, if the data does not exist, then get the data from the backend service and store the result into the cache.
The above is the detailed content of How to use Redis and Dart to develop cache penetration defense functions. For more information, please follow other related articles on the PHP Chinese website!
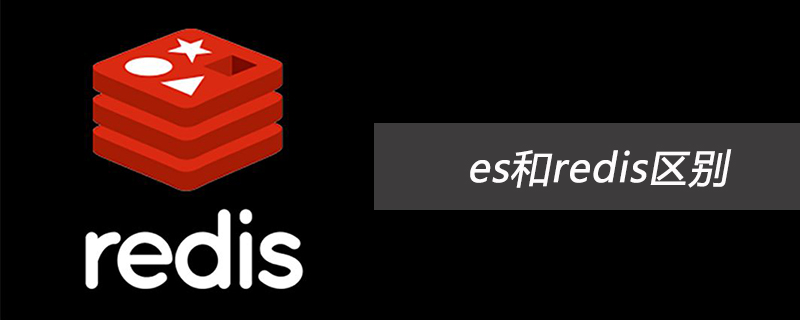
Redis是现在最热门的key-value数据库,Redis的最大特点是key-value存储所带来的简单和高性能;相较于MongoDB和Redis,晚一年发布的ES可能知名度要低一些,ES的特点是搜索,ES是围绕搜索设计的。
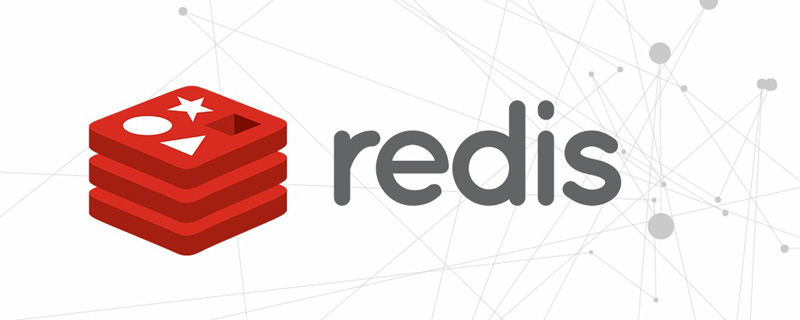
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了关于redis的一些优势和特点,Redis 是一个开源的使用ANSI C语言编写、遵守 BSD 协议、支持网络、可基于内存、分布式存储数据库,下面一起来看一下,希望对大家有帮助。
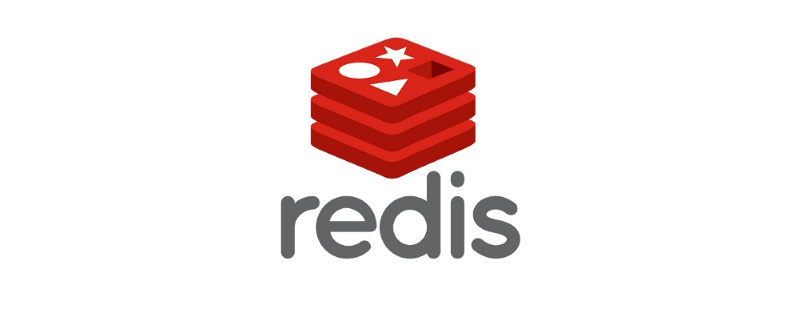
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了Redis Cluster集群收缩主从节点的相关问题,包括了Cluster集群收缩概念、将6390主节点从集群中收缩、验证数据迁移过程是否导致数据异常等,希望对大家有帮助。
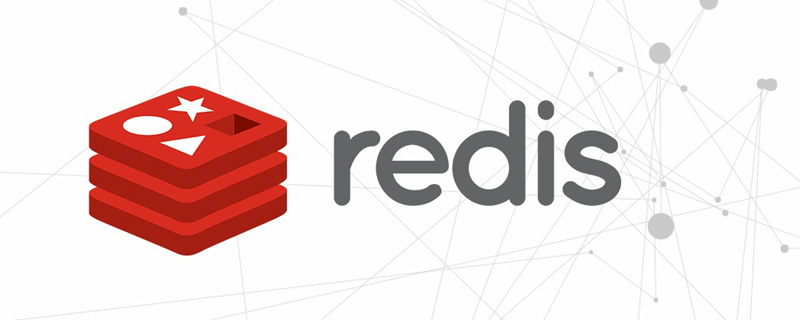
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了Redis实现排行榜及相同积分按时间排序,本文通过实例代码给大家介绍的非常详细,对大家的学习或工作具有一定的参考借鉴价值,希望对大家有帮助。
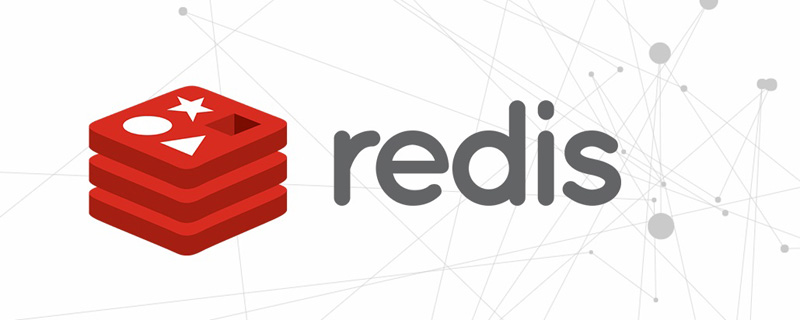
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了关于原子操作中命令原子性的相关问题,包括了处理并发的方案、编程模型、多IO线程以及单命令的相关内容,下面一起看一下,希望对大家有帮助。
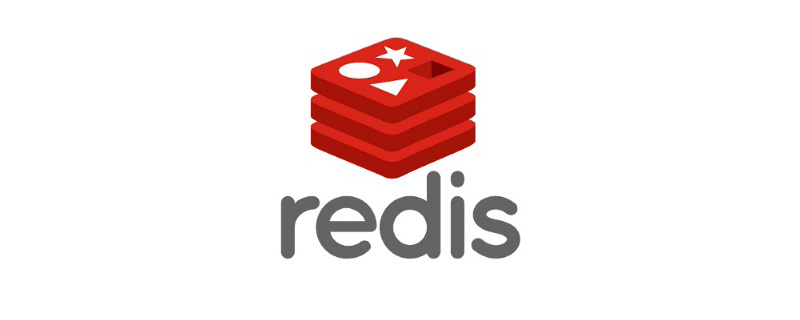
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了Redis实现排行榜及相同积分按时间排序,本文通过实例代码给大家介绍的非常详细,下面一起来看一下,希望对大家有帮助。
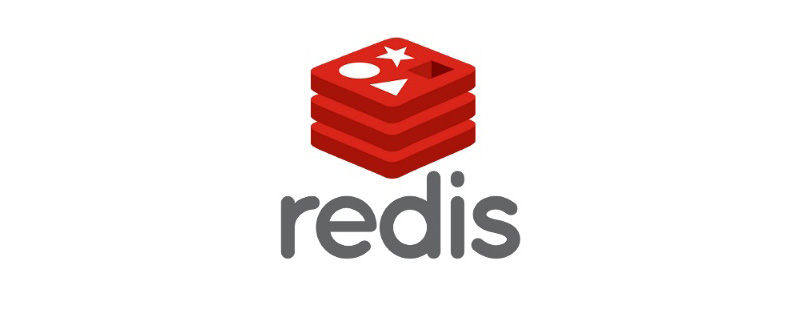
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了bitmap问题,Redis 为我们提供了位图这一数据结构,位图数据结构其实并不是一个全新的玩意,我们可以简单的认为就是个数组,只是里面的内容只能为0或1而已,希望对大家有帮助。
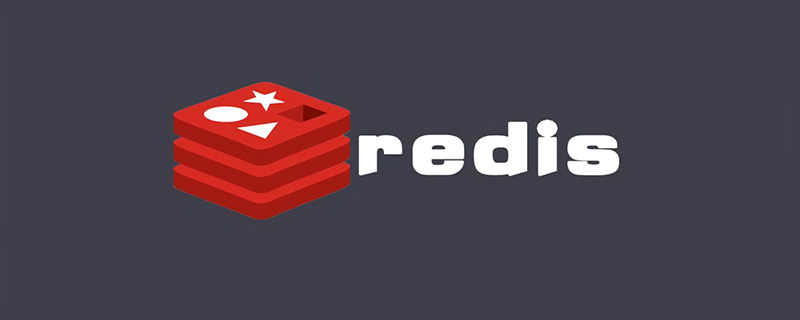
本篇文章给大家带来了关于redis的相关知识,其中主要介绍了关于实现秒杀的相关内容,包括了秒杀逻辑、存在的链接超时、超卖和库存遗留的问题,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
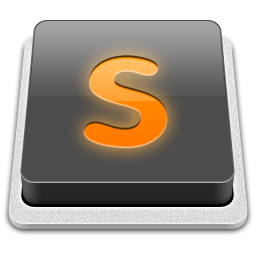
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
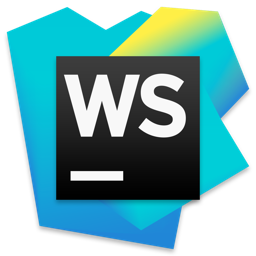
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
